Suggested Videos
Part 3 - Creating your first asp.net mvc application
Part 4 - Controllers in an mvc application
Part 5 - Views in an mvc application
In this video we will discuss
1. What is ViewData
2. What is ViewBag
3. Difference between ViewData and ViewBag
Both ViewData and ViewBag are used to pass data from a controller to a view. ViewData is a dictionary of objects that are stored and retrieved using strings as keys. The syntax of ViewData is very similar to that of ViewState, SessionState and ApplicationState.
// Storing data in ViewData
ViewData["YourData"] = "SomeData";
// Retrieving data from ViewData
string strData = ViewData["YourData"].ToString();
ViewData does not provide compile time error checking. For example, if you mis-spell the key names you wouldn't get any compile time error. You get to know about the error only at runtime.
ViewBag uses the dynamic feature that was introduced in to C# 4.0. It allows an object to have properties dynamically added to it. Using ViewBag the above code can be rewritten as below.
// Storing data in ViewBag
ViewBag.YourData = "SomeData";
// Retrieving data from ViewBag
string strData = ViewBag.YourData;
Just like ViewData, ViewBag does not provide compile time error checking. For example, if you mis-spell the property name, you wouldn't get any compile time error. You get to know about the error only at runtime.
Internally ViewBag properties are stored as name/value pairs in the ViewData dictionary.
Please Note: To pass data from controller to a view, It's always a good practice to use strongly typed view models instead of using ViewBag & ViewData. Strongly typed view models provide compile time error checking. We will discuss view models in a later video session.
Part 3 - Creating your first asp.net mvc application
Part 4 - Controllers in an mvc application
Part 5 - Views in an mvc application
In this video we will discuss
1. What is ViewData
2. What is ViewBag
3. Difference between ViewData and ViewBag
Both ViewData and ViewBag are used to pass data from a controller to a view. ViewData is a dictionary of objects that are stored and retrieved using strings as keys. The syntax of ViewData is very similar to that of ViewState, SessionState and ApplicationState.
// Storing data in ViewData
ViewData["YourData"] = "SomeData";
// Retrieving data from ViewData
string strData = ViewData["YourData"].ToString();
ViewData does not provide compile time error checking. For example, if you mis-spell the key names you wouldn't get any compile time error. You get to know about the error only at runtime.
ViewBag uses the dynamic feature that was introduced in to C# 4.0. It allows an object to have properties dynamically added to it. Using ViewBag the above code can be rewritten as below.
// Storing data in ViewBag
ViewBag.YourData = "SomeData";
// Retrieving data from ViewBag
string strData = ViewBag.YourData;
Just like ViewData, ViewBag does not provide compile time error checking. For example, if you mis-spell the property name, you wouldn't get any compile time error. You get to know about the error only at runtime.
Internally ViewBag properties are stored as name/value pairs in the ViewData dictionary.
Please Note: To pass data from controller to a view, It's always a good practice to use strongly typed view models instead of using ViewBag & ViewData. Strongly typed view models provide compile time error checking. We will discuss view models in a later video session.
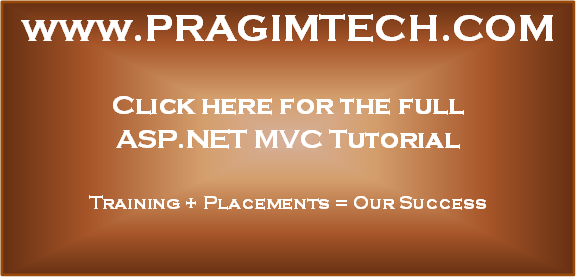
HIIII VENKAT...THANQ SO MUCH FOR POSTING VIDEOS ON MVC...
ReplyDeleteIT'S REALLY AWESOME. IT'S REALLY HELPFUL FOR US
Thank You so much for providing us such a nice tutorials.thanks again and again.
ReplyDeleteHi venkat i try to to some code but got error i am not sure why i am geting null point exception here is my code
ReplyDeletepublic ActionResult Index()
{
ViewBag.Message = new List() {"Ankit","Mark","Chris" };
ViewBag.Work = new List() { ".net", "Java", "C#" };
return View();
}
Name :
@foreach (string name in ViewBag.Message)
{@name }
Work :@foreach (string work in ViewBag.Work)
{@work}
also question how can we do display list data from Viewdata.
ViewBag.Message = new List string { "Ankit", "Mark", "Chris" };
DeleteViewBag.Work = new List string { ".net", "Java", "C#" };
public ActionResult Index()
Delete{
ViewBag.Message = new List { "Ankit", "Mark", "Chris" };
ViewBag.Work = new List{ ".net", "Java", "C#" };
return View();
}
Use the following Lines of Code:
DeleteViewBag.Message = new List {"Sameer","Kamran","Luqman" };
ViewBag.Tech = new List { ".Net", "SQL", "Microsoft" };
return View();
Thanks for the detailed presentation
DeleteTry the following ...
ReplyDeleteViewBag.Message = new List () { "Ankit", "Mark", "Chris" };
ViewBag.Work = new List () { ".net", "Java", "C#" };
I hope it will work fine..
ReplyDeletepublic ActionResult Index()
{
ViewBag.Message=new List{"Ankit","Mark","Chris"};
ViewBag.Work=new List{".net","Java","C#");
return View();
}
how send asp.net webform data to mvc controller?
ReplyDeletepublic ActionResult Index()
ReplyDelete{
List Countries = new List()
{
"Nepal",
"Usa",
"Australia",
"UK"
};
ViewBag.Countries = Countries;
return View();
}
public ActionResult Countries()
{
ViewData["Countries"] = new List(){
"Nepal",
"Russia",
"Japan"
};
return View();
}
Really Nice...
ReplyDeletepublic ActionResult Index()
{
ViewBag.Countries = new List()
{
"India",
"US",
"UK",
"Canada"
};
ViewData["Message"] = new List { "Sameer", "Kamran", "Luqman" };
ViewData["Tech"] = new List { ".Net", "SQL", "Microsoft" };
return View();
}
Simply awesome set of videos!
ReplyDeleteThis comment has been removed by the author.
ReplyDelete// Please try below, it should work!!!
ReplyDeletepublic ActionResult Index()
{
ViewBag.Message = new List() {"Ankit","Mark","Chris" };
ViewBag.Work = new List() { ".net", "Java", "C#" };
return View();
}
Name :
@foreach (string name in ViewBag.Message)
{@name }
Work :@foreach (string work in ViewBag.Work)
{@work}
Hello Sir
ReplyDeleteYou and your knowledge is awesome. I enjoy your tutorial.
How can to display two different list data from Viewdata
Controller Code
public ActionResult Index()
{
ViewBag.Message = new List() { "Ankit", "Mark", "Chris" };
ViewBag.Work = new List() { ".net", "Java", "C#" };
return View();
}
we have to pass list type as argument for new list.
View code :
@foreach (string item in ViewBag.Message)
{
@item
}
@foreach (string item in ViewBag.Work)
{
@item
}
Thank you Venkat sir....my heartfelt gratitude towards u
ReplyDeleteWhen i try to use below code it gives me an error cannot convert char to string. Can anyone help?
ReplyDelete@foreach (string strCity in ViewData["Cities"].ToString())
Hi Venkat,
ReplyDeleteActually in one of the interview, the Interviewer asked me a question on ViewBag and ViewData.
Interviewer: We can achieve the same functionality using ViewData, then what is the use of ViewBag. Why ViewBag was introduced.
I answered him the Basic difference and also ViewBag provides dynamic property i.e. when we put a . we get the property name so there is less chances of error.
But he questioned me again but what if we type the property name wrong again we will get error. So Basically both will give error if the key/property name is invalid.
So I was unable to answer this question. I searched a lot about this on Internet but didn't get proper answer. So may I know is there any specific reason why ViewBag was introduced inspite of ViewData performing the same funcitonality.
Thanks in advance _/\_
I think it cud be bcoz Viewbag does not require typecasting like viewdata
DeleteYes, it is dynamic object, we do not need to typecaste the object, while displaying data on view, when it comes using viewbag. But if we use viewdata, we have to typecaste it to its original type to retrieve it
ReplyDelete