Suggested Videos
Part 1 - Installing asp.net mvc
Part 2 - Which asp.net mvc version is my mvc application using
In this video we will discuss about
1. Creating an asp.net mvc application
2. Understand how mvc request is processed as apposed to webform request
Creating an mvc application:
1. Open visual studio
2. Click File > New Project
3. Select "Web" from "Installed Templates" section
4. Select ASP.NET MVC 4 Web Application
5. Set Name="MVCDemo"
6. Click OK
7. Select "Empty" template. Select "Razor" as the ViewEngine. There are 2 built in view engines - Razor and ASPX. Razor is preferred by most mvc developers. We will discuss about Razor view engine in detail in a later video session.
8. At this point you should have an mvc application created.
Notice that in the solution explorer, you have several folders - Models, Views, Controllers etc. As the names suggest these folders are going to contain Models, Views, and Controllers. We will discuss about Models, Views, and Controllers in a later video session.
Now let's add a controller to our project
1. Right Click on "Controllers" folder
2. Select Add > Controller
3. Set Controller Name = HomeController
4. Leave rest of the defaults and click "Add"
We should have HomeController.cs added to "Controllers" folder.
At this point run the application by pressing CTRL+F5. Notice that you get an error as shown below.
To fix this error, we need to add a view with name, "Index". We will discuss about views in detail in a later video session. Let's fix it another way. The following is the function that is automatically added to HomeController class
public ActionResult Index()
{
return View();
}
Change the return type of Index() function from "ActionResult" to "string", and return string "Hello from MVC Application" instead of View().
public string Index()
{
return "Hello from MVC Application";
}
Run the application and notice that, the string is rendered on the screen. When you run the application, by default it is using built-in asp.net development server. Let's use IIS, to run the application instead of the built-in asp.net development server.
1. In the solution explorer, right click on the project and select "Properties"
2. Click on "Web" tab
3. Select "Use Local IIS Web Server" radio button
4. Notice that the Project Url is set to http://localhost/MVCDemo by default
5. Finally click on "Create Virtual Directory" button
Run the application, and notice that the URL is "http://localhost/MVCDemo/"
Now change the URL to "http://localhost/MVCDemo/Home/index"
In the URL "Home" is the name of the controller and "Index" is the method within HomeController class.
So the improtant point to understand is that the URL is mapped to a controller action method. Where as in web forms application, the URL is mapped to a physical file. For example, in a web application, if we have to display the same message.
1. We add a webform and in the Page_load() event include Response.Write("Hello from ASP.NET Web Forms Application");
2. We then access WebForm1.aspx as shown below
http://localhost/WebFormsApplication/WebForm1.aspx
3. The Page load event gets executed and the message string is displayed.
Part 1 - Installing asp.net mvc
Part 2 - Which asp.net mvc version is my mvc application using
In this video we will discuss about
1. Creating an asp.net mvc application
2. Understand how mvc request is processed as apposed to webform request
Creating an mvc application:
1. Open visual studio
2. Click File > New Project
3. Select "Web" from "Installed Templates" section
4. Select ASP.NET MVC 4 Web Application
5. Set Name="MVCDemo"
6. Click OK
7. Select "Empty" template. Select "Razor" as the ViewEngine. There are 2 built in view engines - Razor and ASPX. Razor is preferred by most mvc developers. We will discuss about Razor view engine in detail in a later video session.
8. At this point you should have an mvc application created.
Notice that in the solution explorer, you have several folders - Models, Views, Controllers etc. As the names suggest these folders are going to contain Models, Views, and Controllers. We will discuss about Models, Views, and Controllers in a later video session.
Now let's add a controller to our project
1. Right Click on "Controllers" folder
2. Select Add > Controller
3. Set Controller Name = HomeController
4. Leave rest of the defaults and click "Add"
We should have HomeController.cs added to "Controllers" folder.
At this point run the application by pressing CTRL+F5. Notice that you get an error as shown below.
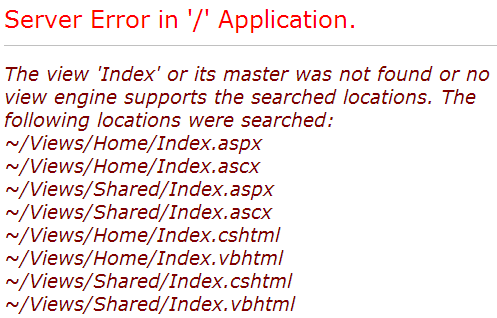
To fix this error, we need to add a view with name, "Index". We will discuss about views in detail in a later video session. Let's fix it another way. The following is the function that is automatically added to HomeController class
public ActionResult Index()
{
return View();
}
Change the return type of Index() function from "ActionResult" to "string", and return string "Hello from MVC Application" instead of View().
public string Index()
{
return "Hello from MVC Application";
}
Run the application and notice that, the string is rendered on the screen. When you run the application, by default it is using built-in asp.net development server. Let's use IIS, to run the application instead of the built-in asp.net development server.
1. In the solution explorer, right click on the project and select "Properties"
2. Click on "Web" tab
3. Select "Use Local IIS Web Server" radio button
4. Notice that the Project Url is set to http://localhost/MVCDemo by default
5. Finally click on "Create Virtual Directory" button
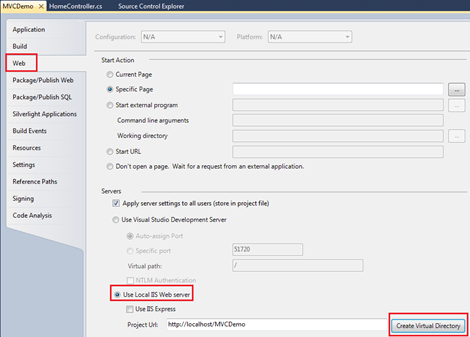
Run the application, and notice that the URL is "http://localhost/MVCDemo/"
Now change the URL to "http://localhost/MVCDemo/Home/index"
In the URL "Home" is the name of the controller and "Index" is the method within HomeController class.
So the improtant point to understand is that the URL is mapped to a controller action method. Where as in web forms application, the URL is mapped to a physical file. For example, in a web application, if we have to display the same message.
1. We add a webform and in the Page_load() event include Response.Write("Hello from ASP.NET Web Forms Application");
2. We then access WebForm1.aspx as shown below
http://localhost/WebFormsApplication/WebForm1.aspx
3. The Page load event gets executed and the message string is displayed.
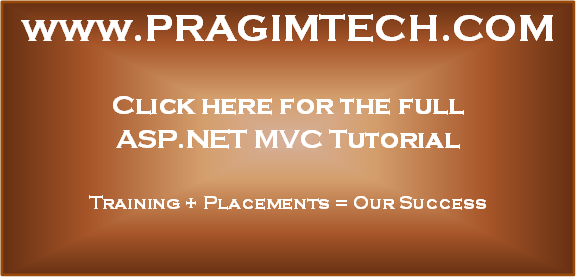
This comment has been removed by the author.
ReplyDeleteHi Venkat sir im executing MVCDEMO Application get below error
ReplyDeleteError: JavaScript runtime error: Unable to get property 'appendChild' of undefined or null reference
HI sir
ReplyDeleteIn properties -web i could not find "Use Local IIS Web Server" radio button. kindly do the needful.
If you are using Visual Studio 2013 there will not be the option for "Use Local IIS Web Server" radio button.
DeleteInstead, look under "Servers" section
Then, you should see a checkbox for "Apply server seetings to all users (store in project file) This should be checked by default. If it is then make sure the drop down list has "IIS Express" selected. This was my default.
Next, for "Project Url" make sure that matches what was said in the tutorial. ("http://localhost/MVCDemo/Home/index") IMPORTANT: if there is a port number listed in the Project Url such as mine ("http://localhost:62805") make sure you keep that when you change it. Mine ended up being "http://localhost:62805/MVCDemo" You will need to then click the "Create Virtual Directory Button and you will likely be prompted to create the virtual directory.
As a side note, if you are curious, like me, and want to know where it puts these settings at you will want to navigate to C:\Users\[USERNAME]\Documents\IISExpress\config and the settings are placed in the applicationhost.config file.
Worked for me in VS2015.Thank you!:)
DeletePlease help.... I did whatever u told...but this error is coming.... The Web server is configured to not list the contents of this directory.
ReplyDeleteDid you build the project?
ReplyDeleteFacing this error when i am trying to run Using IIS
ReplyDeleteError Code 0x80070021
Config Error This configuration section cannot be used at this path. This happens when the section is locked at a parent level. Locking is either by default (overrideModeDefault="Deny"), or set explicitly by a location tag with overrideMode="Deny" or the legacy allowOverride="false".
Config File \\?\E:\Dot NET\ADVANCE TOPICS\Vedkant MVC\MVCDemo\MVCDemo\web.config
please suggest what i write in web config file?
jai vikrat sir u r a superb guy . u are a best in the world .. thanks
ReplyDeleteHello sir, when i run this project showing an error.."unable to launch iis express web server port 80 is in use"
ReplyDeletehow to resolve it sir.
Sir I am Student When I Run this Prog As Per Instruction.
ReplyDeleteI Am Getting Error Like
cannot debug some of code in process w3wp.exe.
intellitrace code failed.
The system cannot find the file specified.
Managed(v4.5,v4.0) code succeeded...
Venkat sir Plz help me
hi venkat sir this is a guy from Andhra without your tutorials i am nothing your videos really helped me to grab my desired job
ReplyDelete-----thank you :)
Dear Sir, Thanks for your videos. It is very useful. You are so nice that you are sharing your knowledge with so many people.
ReplyDeleteI have a issue while following this - I am new to MVC platform. when I click cntrl F5 after creating the controller file. Is there any additions in View has to be done.
Hello Venkat Sir,
ReplyDeleteThank you for your videos
Can you please clarify As you are using Visual studio 2010 but i am using 2012 so when I am doing the steps in Properties Click on web tab and Select "Use Local IIS Web Server" radio button.Still the url is not changed to http://localhost/MVCDemo still its showing http://localhost:1526/ I am unable to get the full path of the controller and index "http://localhost/MVCDemo/Home/index" in my address bar. it is always showing http://localhost:portnumbers please help me out
I'm new to mvc, could i get any example to create a mvc application (Insert, update, delete)
ReplyDeleteHi venkat,
ReplyDeleteYour tutorial as well as video is awesome and unique way of teaching.
Kindly provide webserver tutorial which includes lifecycle of iis after running our asp.net application how iis starts trigerring from top to bottom.
thanks in adv
sir,when i do run visual studio,here error occured
ReplyDelete"HTTP Error 500.19 - Internal Server Error
The requested page cannot be accessed because the related configuration data for the page is invalid."
what is solution?
when i run this project showing an error.."unable to launch iis express web server port 80 is in use"
ReplyDeleteHi Venkat,
ReplyDeleteWhile Creating Virtual Directory is it compulsory to provide a port number? I did as you said but it shows the directory as " http://localhost:5804/MyFirstMVC " and while running it doesnt shows Controller name or index.Can you help me to figure it out?
sir, kindly tell me the default url in route config for all controllers
ReplyDeleteor which to change for error free code.