Suggested Videos
Part 1 - Installing asp.net mvc
Part 2 - Which asp.net mvc version is my mvc application using
Part 3 - Creating your first asp.net mvc application
In this video we will discuss about controllers. Please watch Part 3 of MVC tutorial before proceeding. In Part 3, we discussed that, the URL - http://localhost/MVCDemo/Home/Index will invoke Index() function of HomeController class. So, the question is, where is this mapping defined. The mapping is defined in Global.asax. Notice that in Global.asax we have RegisterRoutes() method.
RouteConfig.RegisterRoutes(RouteTable.Routes);
Right click on this method, and select "Go to Definition". Notice the implementation of RegisterRoutes() method in RouteConfig class. This method has got a default route.
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional }
);
}
The following URL does not have id. This is not a problem because id is optional in the default route.
http://localhost/MVCDemo/Home/Index
Now pass id in the URL as shown below and press enter. Nothing happens.
http://localhost/MVCDemo/Home/Index/10
Change the Index() function in HomeController as shown below.
public string Index(string id)
{
return "The value of Id = " + id;
}
Enter the following URL and press enter. We get the output as expected.
http://localhost/MVCDemo/Home/Index/10
In the following URL, 10 is the value for id parameter and we also have a query string "name".
http://localhost/MVCDemo/home/index/10?name=Pragim
Change the Index() function in HomeController as shown below, to read both the parameter values.
public string Index(string id, string name)
{
return "The value of Id = " + id + " and Name = " + name;
}
Just like web forms, you can also use "Request.QueryString"
public string Index(string id)
{
return "The value of Id = " + id + " and Name = " + Request.QueryString["name"];
}
Part 1 - Installing asp.net mvc
Part 2 - Which asp.net mvc version is my mvc application using
Part 3 - Creating your first asp.net mvc application
In this video we will discuss about controllers. Please watch Part 3 of MVC tutorial before proceeding. In Part 3, we discussed that, the URL - http://localhost/MVCDemo/Home/Index will invoke Index() function of HomeController class. So, the question is, where is this mapping defined. The mapping is defined in Global.asax. Notice that in Global.asax we have RegisterRoutes() method.
RouteConfig.RegisterRoutes(RouteTable.Routes);
Right click on this method, and select "Go to Definition". Notice the implementation of RegisterRoutes() method in RouteConfig class. This method has got a default route.
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional }
);
}
The following URL does not have id. This is not a problem because id is optional in the default route.
http://localhost/MVCDemo/Home/Index
Now pass id in the URL as shown below and press enter. Nothing happens.
http://localhost/MVCDemo/Home/Index/10
Change the Index() function in HomeController as shown below.
public string Index(string id)
{
return "The value of Id = " + id;
}
Enter the following URL and press enter. We get the output as expected.
http://localhost/MVCDemo/Home/Index/10
In the following URL, 10 is the value for id parameter and we also have a query string "name".
http://localhost/MVCDemo/home/index/10?name=Pragim
Change the Index() function in HomeController as shown below, to read both the parameter values.
public string Index(string id, string name)
{
return "The value of Id = " + id + " and Name = " + name;
}
Just like web forms, you can also use "Request.QueryString"
public string Index(string id)
{
return "The value of Id = " + id + " and Name = " + Request.QueryString["name"];
}
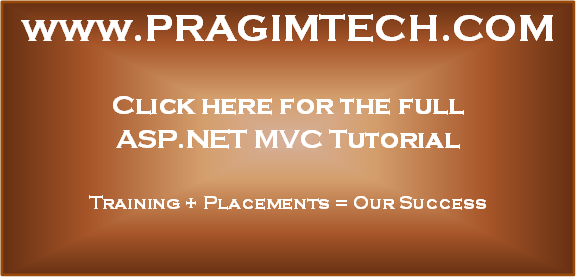
hi,
ReplyDeleteYou said by default it will look for "Home" Controller which is provided in defaults values. But you named it as "HomeController" in your demo. How it can know that "HomeController" is "Home"?
That is by convention in MVC. All controllers will have Controller suffix. This suffix is not required in the URL. So, if you want to invoke Home controller, you specify /Home and not /HomeController. Hope this clarifies your question.
DeleteController is Auto Included in the Name so when we enter /Home MVC search for HOME controller am i right @venkat
Delete#jawadahmed
home is a child class of parent class controller so index fuction is the method of home class whose inherit to controller class
Deletehello
ReplyDeleteI have already tried your first Mvc application.but the fact is that localhost//MVCDEMO/ is showing resource not found.I cant figure it out plz help.
Hi Anudeep ,Just you remove project name from url
Deletelocalhost/controlname/actionmethod(localhost/home/index)
try this it will work
Hi,
ReplyDeleteIn this chapter, we have written Index method of Home Controller as below
public string Index(string id, string name)
{
return "ID = " + id + "Name = " + name ;
}
We made only "id" parameter as optional in Global.ascx but not "name" parameter right. Even though, we are aren't passing any value to "name" parameter, how its taking empty string as its default value.
string is nullable type, but int is not if you change data type of name parameter you will see exception
DeleteHi Pragim
ReplyDeleteIf I, pass HomeController in url insted of Home, it give an error, why?
Hi you don't have to pass HomeController if you want to point to 'Home' action.....
DeleteRouting Engine will take care of appending Controller on ur behalf(as a browser client). Its a 2 step process that happens after you specify the url in the browser & hit enter.
First is appending 'Controller' to the controller information(in ur case 'Home') in the url and Second is search for the HomeController within the MVC application that's executing...
Why we take return type string instead of ActionResult and how will i get value in view by using ActionResult return type ?
ReplyDeleteYou can use viewdata or viewbag to get the value of that variable in the view.
Deletehi sir,
ReplyDeletecan u tell me about routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
Hello,
ReplyDeleteAs you mentioned the URL should not include the project name then how should we access multiple projects on same system using loclhost/controller/action name.
Why we r passing id and when it needs. I don't understand actually why we r passing id here.. what is advantage of it.
ReplyDeleteWe wont use pass id specifically. What it means is we can pass variables into action method through URL of the request
DeleteIt is not required to pass id. We can send variables to action method through URL also like query strings in asp.net.
DeleteThat piece of code does't work proper..please clarify
ReplyDeletepublic string Index(string id, string name)
{
return "The value of Id = " + id + " and Name = " + name;
}
its work fine.I think ur controller name might be wrong.plz check your controller....
DeleteOtherwise plz post error message
How to pass three paramaeters in to url?
Deletepublic string Index(string id,string name,double salary)
{
return "Your id : =" + id+"
"+
"Your name is :"+name + "
" +
"Your salary is :"+salary;
}
url::http://localhost:23105/Home/Index/10?NaMe=suresh&&salary=1000
ReplyDeleteI am getting the below trace error even though i have written a trace element in the web.config file.
Trace Error
Description: Trace.axd is not enabled in the configuration file for this application. Note: Trace is never enabled when
It seems that you have not configured web.config file correctly.
DeleteOpen web.config file.
Under tag add below tag
.
.
.
Hello
ReplyDeleteI having to some exception to convert xml data file into web and i want to import that file in .xlsx format please post this solution as soon as possible.
thanks