Suggested Videos
Part 2 - Which asp.net mvc version is my mvc application using
Part 3 - Creating your first asp.net mvc application
Part 4 - Controllers in an mvc application
In this video we will discuss views. Please watch Part 4 of MVC tutorial before proceeding. Let's change the Index() method in HomeController to return a list of country names. Since country names are strings, return List<string>. Get rid of id and name parameters.
public List<string> Index()
{
return new List<string>()
{
"India",
"US",
"UK",
"Canada"
};
}
Run the application and notice that the output is not as expected.
System.Collections.Generic.List`1[System.String]
To correct this problem, let's add a view to our project.
1. Right click on the Index() function
2. Click on "Add View"
3. Notice that, the view name in "Add View" dialog box matches the name of the controller action method
4. Select "Razor" as the view engine
5. Leave the rest of the defaults as is, and click "Add" button
Make the following modifications to the Index() function of the HomeController class, so that, the HomeController returns a view instead of List<string>.
// Change the return type from List<string> to ActionResult
public ActionResult Index()
{
// Store the list of Countries in ViewBag.
ViewBag.Countries = new List<string>()
{
"India",
"US",
"UK",
"Canada"
};
// Finally return a view
return View();
}
We will discuss ViewBag & ViewData, and the differences between them in our next video session. For now, understand that, ViewBag & ViewData is a mechanism to pass data from the controller to the view.
Please Note: To pass data from controller to a view, It's always a good practice to use strongly typed view models instead of using ViewBag & ViewData. We will discuss view models in a later video session.
Now, copy and paste the following code in "Index.cshtml" view
@{
ViewBag.Title = "Countries List";
}
<h2>Countries List</h2>
<ul>
@foreach (string strCountry in ViewBag.Countries)
{
<li>@strCountry</li>
}
</ul>
Please Note: We use "@" symbol to switch between html and c# code. We will discuss razor views and their syntax in a later video session.
Part 2 - Which asp.net mvc version is my mvc application using
Part 3 - Creating your first asp.net mvc application
Part 4 - Controllers in an mvc application
In this video we will discuss views. Please watch Part 4 of MVC tutorial before proceeding. Let's change the Index() method in HomeController to return a list of country names. Since country names are strings, return List<string>. Get rid of id and name parameters.
public List<string> Index()
{
return new List<string>()
{
"India",
"US",
"UK",
"Canada"
};
}
Run the application and notice that the output is not as expected.
System.Collections.Generic.List`1[System.String]
To correct this problem, let's add a view to our project.
1. Right click on the Index() function
2. Click on "Add View"
3. Notice that, the view name in "Add View" dialog box matches the name of the controller action method
4. Select "Razor" as the view engine
5. Leave the rest of the defaults as is, and click "Add" button
Make the following modifications to the Index() function of the HomeController class, so that, the HomeController returns a view instead of List<string>.
// Change the return type from List<string> to ActionResult
public ActionResult Index()
{
// Store the list of Countries in ViewBag.
ViewBag.Countries = new List<string>()
{
"India",
"US",
"UK",
"Canada"
};
// Finally return a view
return View();
}
We will discuss ViewBag & ViewData, and the differences between them in our next video session. For now, understand that, ViewBag & ViewData is a mechanism to pass data from the controller to the view.
Please Note: To pass data from controller to a view, It's always a good practice to use strongly typed view models instead of using ViewBag & ViewData. We will discuss view models in a later video session.
Now, copy and paste the following code in "Index.cshtml" view
@{
ViewBag.Title = "Countries List";
}
<h2>Countries List</h2>
<ul>
@foreach (string strCountry in ViewBag.Countries)
{
<li>@strCountry</li>
}
</ul>
Please Note: We use "@" symbol to switch between html and c# code. We will discuss razor views and their syntax in a later video session.
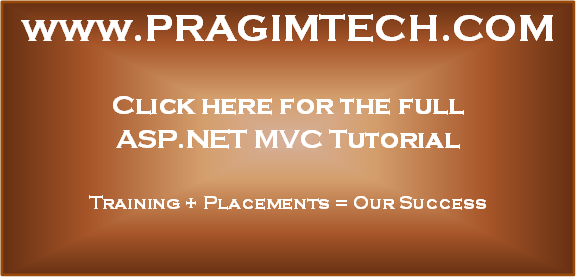
Thank U so Much Sir.....
ReplyDeleteRealy Super Teaching Sir....
By Seeing u r Vidoes only I Get Full Hope my Self.....
Thank you, Really its helpful, Simple Clear.. Stay UP
ReplyDeleteHusham
want a lear picture of login page in MVC 4.0
ReplyDeleteReally nice explanation for Beginners and Dummies....!
ReplyDeleteYes indeed He is a blessed teacher.. Everyone can understand His teachings..
DeleteHi,
ReplyDeletewhy we didn't needed a view to show values when return type was string?
Because MVC view knows the string type and it will parse and display directly when it comes to list we have to parse explicitly as html don't know about list.
Deletethats we are using Viewbag to do that job. hope it clears your doubt.
viewbag nd dataview just like a control like as lable,textbox...we all knw control strore string type of value.. when we need to convert then we convert that values. but ore value is string then we do'nt need to explicit convt..i hope... ur doubt would be clear
Deletehello sir
ReplyDeleteam facing a error at this line
Line 7: @foreach(string strCountry in ViewBag.Countries)
error is "Cannot convert type 'char' to 'string'"
plz solve it
Good Morning Sir,
ReplyDeleteThis is PARUSHURAM.
Dot Net All Videos Good.
ASP.NET MVC,AngularJS,Bootstrap Good Tutorial .
Please Start Xamarin With C# (Android) Tutorial classes.
Thanks Sir.
I love your teaching method and now I'm able to develop any application in mvc..
ReplyDeleteThank you so much
If you were not there Programming wouldn't be possible for me.
ReplyDeleteThanks alot Sir..!
pls make video on login using ad authentication login
ReplyDelete