Suggested Videos
Part 35 - Grouping routes and creating component less route in angular | Text | Slides
Part 36 - Lazy loading in angular | Text | Slides
Part 37 - Preloading angular modules | Text | Slides
In this video we will discuss implementing custom preloading strategy in Angular.
To control when a lazy loaded module is downloaded onto the client machine, we use preloadingStrategy property. We discussed this in detail in Part 37 of Angular 6 tutorial.
Let us say in our Angular project, we have 2 lazy loaded modules
On the other hand, if we set preloadingStrategy property to NoPreloading, then none of the lazy loaded modules will be preloaded.
So with the following 2 built-in preloadingStrategy options, we can either preload, all lazy loaded modules or none of them.
Steps for creating Custom Preloading Strategy in Angular
Step 1 : To create a Custom Preloading Strategy, create a service that implements Angular's built-in PreloadingStrategy abstract class
To generate the service, use the following Angular CLI command
This command generates a file with name custom-preloading.service.ts. Modify the code in that file as shown below.
Step 2 : Import CustomPreloadingService and set it as the Preloading Strategy
In app-routing.module.ts, import CustomPreloadingService
Set CustomPreloadingService as the Preloading Strategy
Modify the 'employees' route, and set preload property to true or false. Set it to true if you want the EmployeeModule to be preloaded else false.
Part 35 - Grouping routes and creating component less route in angular | Text | Slides
Part 36 - Lazy loading in angular | Text | Slides
Part 37 - Preloading angular modules | Text | Slides
In this video we will discuss implementing custom preloading strategy in Angular.
To control when a lazy loaded module is downloaded onto the client machine, we use preloadingStrategy property. We discussed this in detail in Part 37 of Angular 6 tutorial.
Let us say in our Angular project, we have 2 lazy loaded modules
- EmployeeModule
- AdminModule
RouterModule.forRoot(appRoutes, {preloadingStrategy: PreloadAllModules})
On the other hand, if we set preloadingStrategy property to NoPreloading, then none of the lazy loaded modules will be preloaded.
RouterModule.forRoot(appRoutes, {preloadingStrategy: NoPreloading})
So with the following 2 built-in preloadingStrategy options, we can either preload, all lazy loaded modules or none of them.
- PreloadAllModules
- NoPreloading
Steps for creating Custom Preloading Strategy in Angular
Step 1 : To create a Custom Preloading Strategy, create a service that implements Angular's built-in PreloadingStrategy abstract class
To generate the service, use the following Angular CLI command
ng g s CustomPreloading
This command generates a file with name custom-preloading.service.ts. Modify the code in that file as shown below.
import
{ Injectable } from '@angular/core';
import
{ PreloadingStrategy, Route } from '@angular/router';
import
{ Observable, of } from 'rxjs';
@Injectable({
providedIn: 'root'
})
// Since we are creating a Custom
Preloading Strategy, this service
// class must implement
PreloadingStrategy abstract class
export
class CustomPreloadingService implements
PreloadingStrategy {
constructor() { }
// PreloadingStrategy abstract class has the
following preload()
// abstract method for which we need to provide
implementation
preload(route: Route, fn: () =>
Observable<any>): Observable<any> {
// If data property exists on the route of the lazy
loaded module
// and if that data property also has preload
property set to
// true, then return the fn() which preloads the
module
if (route.data && route.data['preload'])
{
return fn();
// If data property does not exist or preload
property is set to
// false, then return Observable of null, so the
module is not
// preloaded in the background
} else {
return of(null);
}
}
}
Step 2 : Import CustomPreloadingService and set it as the Preloading Strategy
In app-routing.module.ts, import CustomPreloadingService
import { CustomPreloadingService } from './custom-preloading.service';
Set CustomPreloadingService as the Preloading Strategy
RouterModule.forRoot(appRoutes, {
preloadingStrategy: CustomPreloadingService
})
preloadingStrategy: CustomPreloadingService
})
Modify the 'employees' route, and set preload property to true or false. Set it to true if you want the EmployeeModule to be preloaded else false.
const appRoutes: Routes =
[
{ path: 'home',
component: HomeComponent },
{ path: '', redirectTo: '/home',
pathMatch: 'full'
},
{
path: 'employees',
// set the preload property to true, using the
route data property
// If you do not want the module to be preloaded
set it to false
data: { preload: true },
loadChildren: './employee/employee.module#EmployeeModule'
},
{ path: '**',
component: PageNotFoundComponent }
];
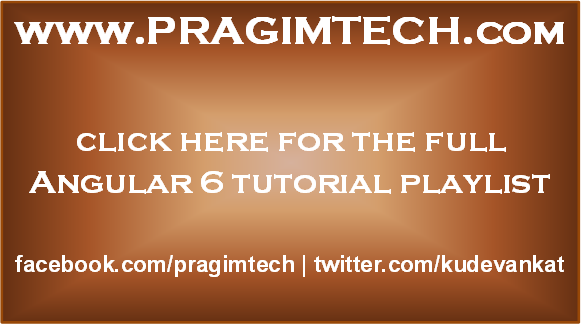
do you have the source code for this entire project?
ReplyDeleteThanks for such a great job. Sir can you cover the interceptor and Authentication and Authorization part of angular so it would great help for us.
ReplyDeletedo you have the source code for this entire project?
ReplyDeleteit's another great hand-on-hand learning course. I just compelted all of lesson 01-38. No big issue for me to follow your code and video. Thanks again!
ReplyDeleteThanks a lot sir this is the best tutorial for the angular2
ReplyDelete