Suggested Videos
Part 32 - Creating feature module in angular | Text | Slides
Part 33 - Creating feature routing module in angular | Text | Slides
Part 34 - Creating shared module in angular | Text | Slides
In this video we will discuss
Consider the following routes in employee-routing.module.ts file
In our upcoming videos we will discuss lazy loading angular module. One of the requirements to be able to lazy load an angular module is the following.
At the moment, the above 3 routes does not have a common route prefix. To achieve this, modify the above route configuration as shown below.
Code explanation :
Part 32 - Creating feature module in angular | Text | Slides
Part 33 - Creating feature routing module in angular | Text | Slides
Part 34 - Creating shared module in angular | Text | Slides
In this video we will discuss
- Grouping routes
- Creating a component less route
Consider the following routes in employee-routing.module.ts file
const appRoutes: Routes =
[
{ path: 'list',
component: ListEmployeesComponent },
{ path: 'create',
component: CreateEmployeeComponent },
{ path: 'edit/:id',
component: CreateEmployeeComponent },
];
In our upcoming videos we will discuss lazy loading angular module. One of the requirements to be able to lazy load an angular module is the following.
All the routes in an angular module that you want to lazy load should have the same route prefix.
At the moment, the above 3 routes does not have a common route prefix. To achieve this, modify the above route configuration as shown below.
const appRoutes: Routes =
[
{
path: 'employees',
children: [
{ path: '', component:
ListEmployeesComponent },
{ path: 'create',
component: CreateEmployeeComponent },
{ path: 'edit/:id',
component: CreateEmployeeComponent },
]
}
];
Code explanation :
- Notice we have a parent route with path employees
- The parent 'employees' route has 3 child routes
- All the 3 child routes will be pre-fixed with the parent route path - employees
- Notice the parent route(employees) does not have a component associated with it. That is why this route is called a component less route.
Route Path | Description |
---|---|
/employees | Displays the list of all employees |
/employees/create | Allows to create a new employee |
/employees/edit/1 | Allows to create a edit an existing employee |
Notice now, all the routes in this module have the same route prefix. In our upcoming videos we will discuss lazy loading an angular module.
Update the routes in the navigation menu in app.component.html
<li>
<a routerLinkActive="active"
routerLink="employees">List</a>
</li>
<li>
<a routerLinkActive="active"
routerLink="employees/create">Create</a>
</li>
In list-employees.component.ts, modify the code to redirect to the new EDIT route (employees/edit/id)
editButtonClick(employeeId: number) {
this._router.navigate(['/employees/edit',
employeeId]);
}
In create-employee.component.ts, modify the code to redirect to the new 'employees' route
onSubmit(): void {
this.mapFormValuesToEmployeeModel();
if (this.employee.id) {
this.employeeService.updateEmployee(this.employee).subscribe(
() => this.router.navigate(['employees']),
(err: any) => console.log(err)
);
} else {
this.employeeService.addEmployee(this.employee).subscribe(
() => this.router.navigate(['employees']),
(err: any) => console.log(err)
);
}
}
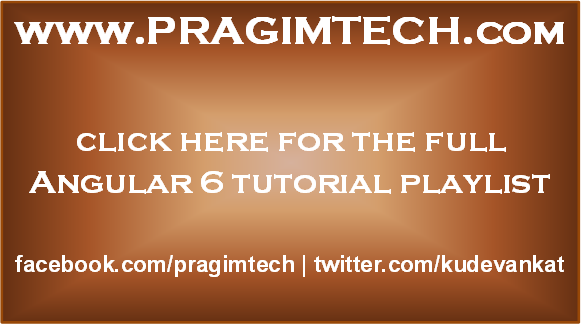
No comments:
Post a Comment
It would be great if you can help share these free resources