Suggested Videos
Part 34 - Creating shared module in angular | Text | Slides
Part 35 - Grouping routes and creating component less route in angular | Text | Slides
Part 36 - Lazy loading in angular | Text | Slides
In this video we will discuss
Module loading strategies in Angular
Eager loading in Angular : With Eager Loading all the modules must be downloaded onto the client machine before the application starts.
Well, yes, the lazy loaded modules can be preloaded in the background after the initial start up bundle is downloaded. Here is how it works.
Preloading in Angular : Preloading is the same as lazy loading but happens slightly differently.
Step 1 : Import PreloadAllModules type from @angular/router package
Step 2 : Set preloadingStrategy to PreloadAllModules.
We do this in the configuration object that we pass as a second parameter to the forRoot() method of the RouterModule class.
The value for preloadingStrategy property can be one of the following
Part 34 - Creating shared module in angular | Text | Slides
Part 35 - Grouping routes and creating component less route in angular | Text | Slides
Part 36 - Lazy loading in angular | Text | Slides
In this video we will discuss
- Different module loading strategies in Angular and the difference between them
- What is Preloading strategy and how to configure it
Module loading strategies in Angular
- Eager Loading
- Lazy Loading
- Preloading
Eager loading in Angular : With Eager Loading all the modules must be downloaded onto the client machine before the application starts.

- If we do not do anything special, by default, the angular modules are eagerly loaded
- The root application module (AppModule) is always eagerly loaded
- Eager loading works fine for small applications because a small application usually has, just a few modules. So eagerly downloading those few modules should not take a long time and hence it is not a performance bottle neck in a small application. But Eager loading all modules before the application starts is not right for a medium or large angular applications. In most cases, in a real world angular application we use a combination of these module loading strategies.
- Only the first request to the application takes a long time, but the subsequent requests from that same client will be faster. This is because, with eager loading, all the modules must be loaded before the application starts. So depending on the number of modules and the internet connection speed, the first request may take a long time, but the subsequent requests from that same client will be faster, because all the modules are already downloaded on to the client machine.
- There is nothing special that we have to do, for an Angular module to be eager loaded. It just needs to be referenced in the application using imports metadata of @NgModule decorator.

- To lazy load a module, it should not be referenced in any other module. If it is referenced, the module loader will eagerly load it instead of lazily loading it. We discussed lazy loading in detail in our previous video.
- The main benefit of Lazy loading is, it can significantly reduce the initial application load time. With lazy loading configured, our application does not load every module on startup. Only the root module and any other essential modules that the user expects to see when the application first starts are loaded. In our example here, only the root module and employee module are loaded when the application starts. The rest of the modules i.e ReportsModule and AdminModule are configured to be lazy loaded so they are not loaded when the application starts. Because of this reduced download size, the application initial load time is reduced to a great extent.
- However there is a downside for lazy loading. When a route in a lazy loaded module is first requested, the user has to wait for that module to be downloaded. We do not have this problem with eager loading, because all the modules are already downloaded, so the end user gets to see the component associated with the route without any wait time.
Well, yes, the lazy loaded modules can be preloaded in the background after the initial start up bundle is downloaded. Here is how it works.
Preloading in Angular : Preloading is the same as lazy loading but happens slightly differently.

- First, the module to bootstrap the application and eager loaded modules are downloaded.
- At this point, we have the application up and running and the user is interacting with the application.
- While the application has nothing else to download, it downloads angular modules configured to preload in the background.
- So, by the time the user navigates to a route in a lazy loaded module, it is already pre-loaded, so the user does not have to wait, and sees the component associated with that route right away.
- So with preloading modules, we have the best of both the worlds i.e Eager Loading and Lazy Loading.
- Preloading is also often called Eager Lazy Loading
Step 1 : Import PreloadAllModules type from @angular/router package
import { PreloadAllModules } from '@angular/router';
Step 2 : Set preloadingStrategy to PreloadAllModules.
We do this in the configuration object that we pass as a second parameter to the forRoot() method of the RouterModule class.
@NgModule({
imports: [
RouterModule.forRoot(appRoutes, { preloadingStrategy: PreloadAllModules })
],
exports: [ RouterModule ]
})
export class AppRoutingModule { }
imports: [
RouterModule.forRoot(appRoutes, { preloadingStrategy: PreloadAllModules })
],
exports: [ RouterModule ]
})
export class AppRoutingModule { }
The value for preloadingStrategy property can be one of the following
Value | Description |
---|---|
NoPreloading | This is the default and does not preload any modules |
PreloadAllModules | Preloads all modules as quickly as possible in the background |
Custom Preload Strategy | We can also specify our own custom preloading strategy. We will discuss why and how to implement custom preloading strategy in our next video. |
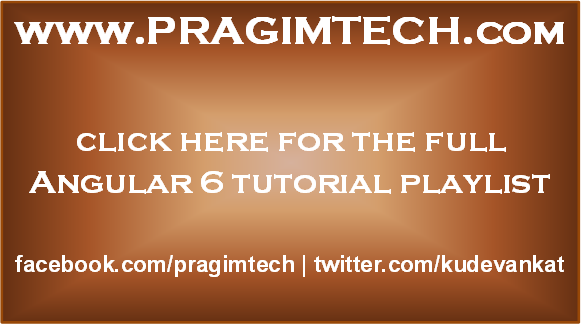
Hi.. My question is: Its a better idea load AppModule and first route(like my index route) eager and others routes lazily?
ReplyDelete