Suggested Videos
Part 23 - Angularjs routing tutorial
Part 24 - Angular layout template
Part 25 - Angularjs partial templates
In this video we will discuss configuring routes and creating controllers. This is continuation to Part 25. Please watch Part 25 from AngularJS tutorial.
Right click on the Scripts folder and add a new JavaScript file. Name it script.js. Copy and paste the following code.
2 Changes to index.html
1. Add a reference to the script.js file in the layout template i.e index.html.
2. Set ng-app="Demo" on the root html element
At this point, depending on the URL, the respective partial template will be injected into the layout template in the location where we have ng-view directive. For example if you have index.html#/home, then home.html is injected into index.html. Similarly if you are on index.html#/courses, then course.html is injected into index.html.
Next video : How to remove hash from URL
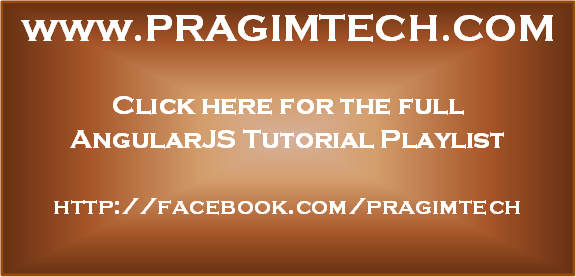
Part 23 - Angularjs routing tutorial
Part 24 - Angular layout template
Part 25 - Angularjs partial templates
In this video we will discuss configuring routes and creating controllers. This is continuation to Part 25. Please watch Part 25 from AngularJS tutorial.
Right click on the Scripts folder and add a new JavaScript file. Name it script.js. Copy and paste the following code.
///
<reference path="angular.min.js" />
var app = angular
.module("Demo", ["ngRoute"])
.config(function ($routeProvider) {
$routeProvider
.when("/home", {
templateUrl: "Templates/home.html",
controller: "homeController"
})
.when("/courses", {
templateUrl: "Templates/courses.html",
controller: "coursesController"
})
.when("/students", {
templateUrl: "Templates/students.html",
controller: "studentsController"
})
})
.controller("homeController",
function
($scope) {
$scope.message = "Home Page";
})
.controller("coursesController", function ($scope) {
$scope.courses = ["C#", "VB.NET", "ASP.NET", "SQL Server"];
})
.controller("studentsController", function ($scope, $http) {
$http.get("StudentService.asmx/GetAllStudents")
.then(function (response) {
$scope.students = response.data;
})
})
2 Changes to index.html
1. Add a reference to the script.js file in the layout template i.e index.html.
<script src="Scripts/script.js"></script>
2. Set ng-app="Demo" on the root html element
<html xmlns="http://www.w3.org/1999/xhtml" ng-app="Demo">
At this point, depending on the URL, the respective partial template will be injected into the layout template in the location where we have ng-view directive. For example if you have index.html#/home, then home.html is injected into index.html. Similarly if you are on index.html#/courses, then course.html is injected into index.html.
Next video : How to remove hash from URL
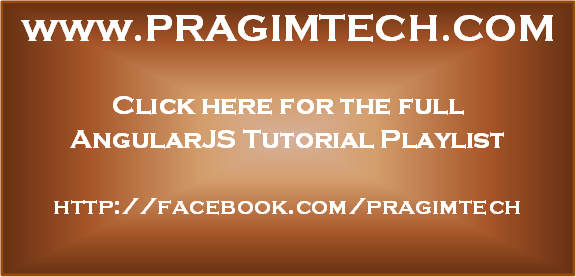
Great Course for AngularJs
ReplyDeleteSimple and easy way approach for learning trending technologies like Angularjs... Thank you for providing us a learning tutorails...
ReplyDeleteHad one query. Due to routeProvider it is not required to define controller in html?
ReplyDeleteHello, great videos - really helpful, but I have a problem, I use VS2015 professional with resharper 10 (if I remember correctly for it) and on the links section I get (with resharper on) id cannot be resolved ( Home for example) and then, when I turn off the resharper I do not get the message anymore, bu I cannot see the partial template either... :< Can someone please help me with this, because these are cool tutorials. :) Great work man!
ReplyDeletei have done this example form video 23-25 after execution navigation are not working then template is not loading. can any one help in this.
ReplyDeleteGuys try to add cdn link. problem got solved!.....
Deletegreat site for the angular learners
ReplyDeletehello sir,
ReplyDeleteAngularJs/index.html#!/
AngularJs/index.html#!/#%2Fgreen
this kind of error happen in URL when I click to link.
please help me
Hi,
DeleteI also faced the same error.
Did followed this to get rid of this issue,
- removed # from leftmenu as below..
"
Home
Courses
Students
"
- added base tag in tag of index page.
- Enabled 'html5Mode' using $locationProvider service in script.js
.config(function ($routeProvider, $locationProvider) {
$routeProvider
.when("/home", {
templateUrl: "Templates/Home.htm",
controller: "homeController"
})
.when("/courses", {
templateUrl: "Templates/Courses.htm",
controller: "coursesController"
})
.when("/students", {
templateUrl: "Templates/Students.htm",
controller: "studentsController"
})
$locationProvider.html5Mode({ enabled: true
});
})
try this if it works for you as well.
Thanks,
Thanks Bhoopendra. Your Fix worked for me.
DeleteHi All,
ReplyDeletePlesae user angular-route.min.js from stable version which is currently 1.2.32 version as latest version is showing compile time errors in eclipse in the Dyanamic Web Application.
Where to add Controllers Name in index.html?
ReplyDeleteThe links were not working for me so I came to know that angular 1.6.1 core and route files have some problem. so here is my JS code to that fixed the links.
ReplyDelete///
var app = angular.module('Demo', ['ngRoute'])
.config(function ($routeProvider, $locationProvider)
{
$locationProvider.hashPrefix('');//this helped fix a bug with angular 1.6.1 http://stackoverflow.com/questions/41211875/angularjs-1-6-0-latest-now-routes-not-working the routing links were not working but this $locationProvider variable change fixed it.
$routeProvider
.when('/home', {
templateUrl: 'Templates/home.html',
controller: 'homeController'
})
.when('/courses', {
templateUrl: 'Templates/courses.html',
controller: 'coursesController'
})
.when('/students', {
templateUrl: 'Templates/students.html',
controller: 'studentsController'
});
})
.controller('homeController', function ($scope)
{
$scope.message = 'Home Page';
})
.controller('coursesController', function ($scope)
{
$scope.courses = ['C#', 'VB.NET', 'SQL Server', 'ASP.NET'];
})
.controller('studentsController', function ($scope, $http)
{
$http.get('Part25StudentService.asmx/GetAllStudents')
.then(function (response)
{
$scope.students = response.data;
});
});
Thanks AJ, I don't understand it but that got it to work! These videos have been awesome, but I will definitely need more ngRoute examples / practice to get my head around this.
DeleteThis solution worked. Thanks
DeleteHi Aj,
DeleteI placed the same code what you are said above, but is not working for me. May i know what is the reason?
After reading the post in AJ s comment. Change in Angular 1-6. Either go with AJ's solution - or - make the href in anchor tags '#!/home' instead or '#/home'
ReplyDeleteThank you , '#!/home' works for me
Deletethank you it works for me href="#!/home" for a_tag is correct
DeleteThank you , '#!/home' works for me, but don't understand why ?
DeleteAm did not getting the list of the student when i click the student link the other two links mean home and courses can anyone help me...???
ReplyDeletehow to remove # tag without using asp bcz this tutorial using asp
ReplyDeletebut i want without asp
hello sir,
ReplyDeleteAngularJs/index.html#!/
AngularJs/index.html#!/#%2Fstudents
I am getting this error please help me i reffered video no 26 route configuration
my problem is solved, i simply added cdn link and problem solved but why we use cdn if we have local script file and if i use local script file it doesn't work. please anybody tell me the reason...
ReplyDeletevar myApp = angular.module("myModule", ["ngRoute"]).config(function ($routeProvider) {
ReplyDelete$routeProvider.when("/home", { templateURL: "Templates/home.html", controller: "homeController" })
.when("/courses", { templateURL: "Templates/courses.html",controller:"coursesCountroller" })
.when("/students", { templateURL: "Templates/students.html", controller: "studentsController" })
.controller("homeController", function ($scope) {
$scope.message = "Home Page!!";
})
.controller("coursesController", function ($scope) {
$scope.courses = ["ASP.NET","C#","VB.NET","SQL Server"];
})
.controller("studentsController", function ($scope, $http)
{
$http.get("StudentService.asmx/StudentData").then(function (response) {
$scope.students = response.data;
})
})
});
The links are not getting loaded and I m getting this error:Error: $injector:modulerr
Module Error
Failed to instantiate module myModule due to:
TypeError: $routeProvider.when(...).controller is not a function
Hi in 1.7.x version we are getting this error. Instead refer 1.2.x for both angular.min.js amd angular-route.min.js. Then the navigation works for Home, Courses, Students
ReplyDeleteHi Venkat Sir,
ReplyDeleteI have implemented the above example only with 2 pages Home and courses as I don't have VS and SQL. I'm getting below error message when I'm clicking on Home or Courses link.
GET http://127.0.0.1:5500/Templates/home.html 404 (Not Found)
(anonymous) @ angular.js:13562
sendReq @ angular.js:13288
serverRequest @ angular.js:13029
processQueue @ angular.js:17945
(anonymous) @ angular.js:17993
$digest @ angular.js:19112
$apply @ angular.js:19500
(anonymous) @ angular.js:15301
defaultHandlerWrapper @ angular.js:3824
eventHandler @ angular.js:3812
angular.js:15567 Error: [$templateRequest:tpload] Failed to load template: Templates/home.html (HTTP status: 404 Not Found)
https://errors.angularjs.org/1.7.8/$templateRequest/tpload?p0=Templates%2Fhome.html&p1=404&p2=Not%20Found
at angular.js:138
at handleError (angular.js:21397)
at processQueue (angular.js:17945)
at angular.js:17993
at Scope.$digest (angular.js:19112)
at Scope.$apply (angular.js:19500)
at done (angular.js:13343)
at completeRequest (angular.js:13600)
at XMLHttpRequest.requestLoaded (angular.js:13505)
(anonymous) @ angular.js:15567
(anonymous) @ angular.js:11846
handleError @ angular.js:21401
processQueue @ angular.js:17945
(anonymous) @ angular.js:17993
$digest @ angular.js:19112
$apply @ angular.js:19500
done @ angular.js:13343
completeRequest @ angular.js:13600
requestLoaded @ angular.js:13505
load (async)
(anonymous) @ angular.js:13488
sendReq @ angular.js:13288
serverRequest @ angular.js:13029
processQueue @ angular.js:17945
(anonymous) @ angular.js:17993
$digest @ angular.js:19112
$apply @ angular.js:19500
(anonymous) @ angular.js:15301
defaultHandlerWrapper @ angular.js:3824
eventHandler @ angular.js:3812
Do we really need Visual Studio and web.config file to get this example work.
Please advise.
Hello friends,
ReplyDeleteI'm very late to ask anything on this tutorial and sorry about that. I followed twice this 3 videos but still unable to get the result as I should get it. first of all, json(StudentService.asmx) working absolutely fine. when I'm testing 3 html files separately its not working, I mean it is not displaying the either course data nor student data. The same way index.html file is also not displaying anything when click on home, course, student links.
Any help would be much appreciated.