Suggested Videos
Part 22 - Angular anchorscroll with database data
Part 23 - Angularjs routing tutorial
Part 24 - Angular layout template
In this video we will discuss creating the partial templates i.e home.html, courses.html and students.html. This is continuation to Part 24. Please watch Part 24 from AngularJS tutorial before proceeding.
One important thing to keep in mind is that, since we have all the surrounding HTML (i.e html, head, body etc) in the layout view (index.html), there is no need to include that same surrounding HTML again in the partial templates.
All our partial templates are going to be in Templates folder. So first add Templates folder to the project's root folder.
home.html : Right click on the Templates folder and add a new HTML file. Name it home.html. Copy and paste the following. The homeController will set the message property on the $scope object. We will discuss creating the homeController in a later video.
courses.html : The coursesController will set the courses property on the $scope object. We will discuss creating the coursesController in a later video.
students.html : The students data is going to come from a database table. So here are the steps
Step 1 : Create the database table (tblStudents) and populate it with test data.
Step 2 : Include the following configuration in web.config file. Notice we have a connection string to the database. We also have enabled HttpGet protocol for ASP.NET web services.
Step 3 : Add a class file to the project. Name it Student.cs. Copy and paste the following code.
Step 4 : Add an ASMX web service to the project. Name it StudentService.asmx. Copy and paste the following code.
Step 5 : Right click on the Templates folder and add a new HTML file. Name it students.html. Copy and paste the following. The studentsController will set the students property on the $scope object. We will discuss creating the studentsController in a later video.
In our next video we will discuss configuring angularjs routes and creating the controllers i.e homeController, coursesController and studentsController.
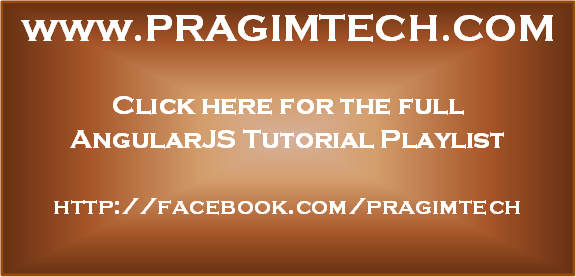
Part 22 - Angular anchorscroll with database data
Part 23 - Angularjs routing tutorial
Part 24 - Angular layout template
In this video we will discuss creating the partial templates i.e home.html, courses.html and students.html. This is continuation to Part 24. Please watch Part 24 from AngularJS tutorial before proceeding.
One important thing to keep in mind is that, since we have all the surrounding HTML (i.e html, head, body etc) in the layout view (index.html), there is no need to include that same surrounding HTML again in the partial templates.
All our partial templates are going to be in Templates folder. So first add Templates folder to the project's root folder.
home.html : Right click on the Templates folder and add a new HTML file. Name it home.html. Copy and paste the following. The homeController will set the message property on the $scope object. We will discuss creating the homeController in a later video.
<h1>{{message}}</h1>
<div>
PRAGIM Established in 2000 by 3 S/W
engineers offers very cost effective training. PRAGIM Speciality in training
arena unlike other training institutions
</div>
<ul>
<li>Training delivered by real time software experts having
more than 10 years of experience.</li>
<li>Realtime projects discussion relating to the possible
interview questions.</li>
<li>Trainees can attend training and use lab untill you get a
job.</li>
<li>Resume preperation and mock up interviews.</li>
<li>100% placement assistance.</li>
<li>Lab facility.</li>
</ul>
courses.html : The coursesController will set the courses property on the $scope object. We will discuss creating the coursesController in a later video.
<h1>Courses we offer</h1>
<ul>
<li ng-repeat="course in
courses">
{{course}}
</li>
</ul>
students.html : The students data is going to come from a database table. So here are the steps
Step 1 : Create the database table (tblStudents) and populate it with test data.
Create table tblStudents
(
Id int primary
key identity,
Name nvarchar(50),
Gender nvarchar(10),
City nvarchar(20)
)
Go
Insert into tblStudents values ('Mark', 'Male', 'London')
Insert into tblStudents values ('John', 'Male', 'Chennai')
Insert into tblStudents values ('Sara', 'Female', 'Sydney')
Insert into tblStudents values ('Tom', 'Male', 'New York')
Insert into tblStudents values ('Pam', 'Male', 'Los Angeles')
Insert into tblStudents values ('Catherine', 'Female', 'Chicago')
Insert into tblStudents values ('Mary', 'Female', 'Houston')
Insert into tblStudents values ('Mike', 'Male', 'Phoenix')
Insert into tblStudents values ('Rosie', 'Female', 'Manchester')
Insert into tblStudents values ('Sasha', 'Female', 'Hyderabad')
Go
Step 2 : Include the following configuration in web.config file. Notice we have a connection string to the database. We also have enabled HttpGet protocol for ASP.NET web services.
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<connectionStrings>
<add name="DBCS"
connectionString="server=(local);database=SampleDB; integrated security=true"
providerName="System.Data.SqlClient" />
</connectionStrings>
<system.web>
<webServices>
<protocols>
<add name="HttpGet" />
</protocols>
</webServices>
</system.web>
</configuration>
Step 3 : Add a class file to the project. Name it Student.cs. Copy and paste the following code.
namespace Demo
{
public class Student
{
public int id { get; set; }
public string name { get; set; }
public string gender { get; set; }
public string city { get; set; }
}
}
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Script.Serialization;
using System.Web.Services;
namespace Demo
{
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class StudentService : System.Web.Services.WebService
{
[WebMethod]
public void GetAllStudents()
{
List<Student> listStudents = new List<Student>();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("Select * from
tblStudents", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Student student = new Student();
student.id = Convert.ToInt32(rdr["Id"]);
student.name = rdr["Name"].ToString();
student.gender = rdr["Gender"].ToString();
student.city = rdr["City"].ToString();
listStudents.Add(student);
}
}
JavaScriptSerializer js = new JavaScriptSerializer();
Context.Response.Write(js.Serialize(listStudents));
}
}
}
Step 5 : Right click on the Templates folder and add a new HTML file. Name it students.html. Copy and paste the following. The studentsController will set the students property on the $scope object. We will discuss creating the studentsController in a later video.
<h1>List of Students</h1>
<ul>
<li ng-repeat="student in
students">
{{student.name}}
</li>
</ul>
In our next video we will discuss configuring angularjs routes and creating the controllers i.e homeController, coursesController and studentsController.
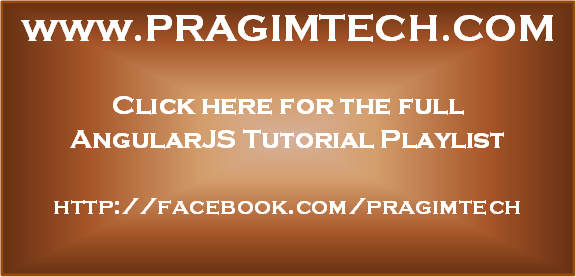
what is partial template?
ReplyDeleteWhy is there no link to go to the next video slide
ReplyDeleteClick on 'new post' bottom of the page
DeletePlease explain ng-view directory that how we call our partial view using ng-view. how ng-view know which page call at what time i am very confused please expain
ReplyDeletewhy it takes time to load data?
ReplyDeletedata not loaded quickely using web service