Suggested Videos
Part 1 - What is Entity Framework
Part 2 - Entity Framework Model First Approach
Entity Framework supports
1. Database first or schema first approach - Discussed in Part 1
2. Model first appraoch - Discussed in Part 2
3. Code first approach - In this video
Code-first approach allows us to create our custom classes first and based on those custom classes entity framework can generate database automatically for us. Let's understand this with an example. We will be modifying the example we worked with in Part 2.
Step 1: Delete EmployeeModel.edmx && EmployeeModel.edmx.sql files from the solution explorer.
Step 2: Add a class file to the project. Name it Employee.cs. Copy and paste the following code.
Step 3: Add a class file to the project. Name it Department.cs. Copy and paste the following code.
Step 4: Add a class file to the project. Name it EmployeeDBContext.cs. Copy and paste the following code.
Step 5: Add a class file to the project. Name it EmployeeRepository.cs. Copy and paste the following code.
Step 6: Add the database connection string in web.config file.
Please Note: If ProviderName is not specified the following runtime error will be thrown.
The connection string 'EmployeeDBContext' in the application's configuration file does not contain the required providerName attribute."
Step 7: Configure Object Data Source control
a) Delete EntityDataSource control, that is already there in WebForm1.aspx.
b) Drag and Drop ObjectDataSource control.
c) Right click on ObjectDataSource control and select "Show Smart Tag" option from the context menu
d) Click on "Configure Data Source..." link
e) On "Choose a Business Object" screen, select "EmployeeRepository" and click "Next"
f) On "Define Data Methods" screen, select GetDepartments() method and click "Finish"
Step 8: Configure GridView control
a) Right click on GridView control and select "Show Smart Tag" option from the context menu
b) Select "ObjectDataSource1" from "Choose Data Source" dropdownlist
c) Click "No" to "Refresh Fields and Keys for GridView1" when prompted
Step 9: Rebuild the solution.
Step 10: Delete the already existing database from SQL Server Management Studio.
Step 11: Run the application by pressing CTRL + F5. Notice that we don't have any data displayed on WebForm1. This is because we don't have any data in the Departments and Employees tables. At this point we have the following created automatically.
a) Sample database
b) Departments table
c) Employees table
Step 12: Use the SQL script to populate the tables with data.
Step 13: Refresh the Web Form and we should see the data we expect.
Part 1 - What is Entity Framework
Part 2 - Entity Framework Model First Approach
Entity Framework supports
1. Database first or schema first approach - Discussed in Part 1
2. Model first appraoch - Discussed in Part 2
3. Code first approach - In this video
Code-first approach allows us to create our custom classes first and based on those custom classes entity framework can generate database automatically for us. Let's understand this with an example. We will be modifying the example we worked with in Part 2.
Step 1: Delete EmployeeModel.edmx && EmployeeModel.edmx.sql files from the solution explorer.
Step 2: Add a class file to the project. Name it Employee.cs. Copy and paste the following code.
public class Employee
{
// Scalar Properties
public int Id
{ get; set;
}
public string FirstName
{ get; set;
}
public string LastName
{ get; set;
}
public string Gender
{ get; set;
}
public int Salary
{ get; set;
}
// Navigation Property
public Department Department { get; set; }
}
Step 3: Add a class file to the project. Name it Department.cs. Copy and paste the following code.
public class Department
{
// Scalar Properties
public int Id
{ get; set;
}
public string Name
{ get; set;
}
public string Location
{ get; set;
}
// Navigation Property
public List<Employee> Employees
{ get; set;
}
}
Step 4: Add a class file to the project. Name it EmployeeDBContext.cs. Copy and paste the following code.
// EmployeeDBContext class must
inherit from DbContext
// present in System.Data.Entity
namespace
public class EmployeeDBContext : DbContext
{
public DbSet<Department> Departments
{ get; set;
}
public DbSet<Employee> Employees
{ get; set;
}
}
Step 5: Add a class file to the project. Name it EmployeeRepository.cs. Copy and paste the following code.
public class EmployeeRepository
{
public List<Department> GetDepartments()
{
EmployeeDBContext employeeDBContext = new EmployeeDBContext();
return employeeDBContext.Departments.Include("Employees").ToList();
}
}
Step 6: Add the database connection string in web.config file.
<connectionStrings>
<add name="EmployeeDBContext"
connectionString="server=.;
database=Sample; integrated security=true;"
providerName="System.Data.SqlClient"/>
</connectionStrings>
Please Note: If ProviderName is not specified the following runtime error will be thrown.
The connection string 'EmployeeDBContext' in the application's configuration file does not contain the required providerName attribute."
Step 7: Configure Object Data Source control
a) Delete EntityDataSource control, that is already there in WebForm1.aspx.
b) Drag and Drop ObjectDataSource control.
c) Right click on ObjectDataSource control and select "Show Smart Tag" option from the context menu
d) Click on "Configure Data Source..." link
e) On "Choose a Business Object" screen, select "EmployeeRepository" and click "Next"
f) On "Define Data Methods" screen, select GetDepartments() method and click "Finish"
Step 8: Configure GridView control
a) Right click on GridView control and select "Show Smart Tag" option from the context menu
b) Select "ObjectDataSource1" from "Choose Data Source" dropdownlist
c) Click "No" to "Refresh Fields and Keys for GridView1" when prompted
Step 9: Rebuild the solution.
Step 10: Delete the already existing database from SQL Server Management Studio.
Step 11: Run the application by pressing CTRL + F5. Notice that we don't have any data displayed on WebForm1. This is because we don't have any data in the Departments and Employees tables. At this point we have the following created automatically.
a) Sample database
b) Departments table
c) Employees table
Step 12: Use the SQL script to populate the tables with data.
Insert into Departments values ('IT', 'New York')
Insert into Departments values ('HR', 'London')
Insert into Departments values ('Payroll', 'Sydney')
Insert into Employees values ('Mark', 'Hastings', 'Male', 60000, 1)
Insert into Employees values ('Steve', 'Pound', 'Male', 45000, 3)
Insert into Employees values ('Ben', 'Hoskins', 'Male', 70000, 1)
Insert into Employees values ('Philip', 'Hastings', 'Male', 45000, 2)
Insert into Employees values ('Mary', 'Lambeth', 'Female', 30000, 2)
Insert into Employees values ('Valarie', 'Vikings', 'Female', 35000, 3)
Insert into Employees values ('John', 'Stanmore', 'Male', 80000, 1)
Step 13: Refresh the Web Form and we should see the data we expect.
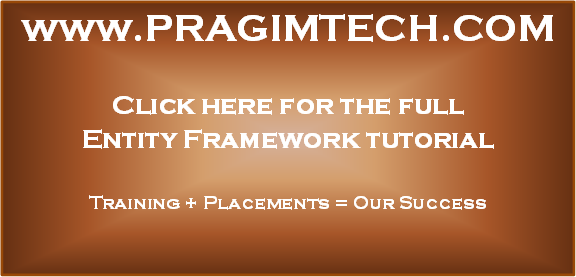
Venkat Sir,
ReplyDeleteWould you please discuss how to call Stored Procedures when we use Code First Approach or Model First Approach ?
And Would you please discuss Unit Of Work in Repository Pattern?
Thanks
Hi
ReplyDeleteI am not getting the EntityRepository.cs fill what do sir
i have done all things correct
Hey class should be public
Deletesir I am facing an Exception in repository class as"
ReplyDeleteAn exception of type 'System.Data.Entity.ModelConfiguration.ModelValidationException' occurred in EntityFramework.dll but was not handled in user code
Additional information: One or more validation errors were detected during model generation:
CodeFirstApproach_EFDemo.Departments: : EntityType 'Departments' has no key defined. Define the key for this EntityType.
department: EntityType: EntitySet 'department' is based on type 'Departments' that has no keys defined.
using System.ComponentModel.DataAnnotations.Schema;
Deletenamespace ConsoleEntityFramework.Models
{
public class Employee
{
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public string Gender { get; set; }
public int Salary { get; set; }
public int DepartmentId { get; set; }
[ForeignKey("DepartmentId")]
public Department Department { get; set; }
}
}
respected sir,
ReplyDeletei am new to both development and deployment .
i have developed a simple mvc5 application which is using entity framework to access the database.
it was working fine in my localhost .
i have published the application in godaddy .
now the app in godaddy is allowing me to insert the data into database but when i am trying to retrieve the data i am getting error .
my connection string in web.config is as follows
the error message is as follows
The connection string 'DBContext' in the application's configuration file does not contain the required providerName attribute."
please guide me through .
my mail id is mulladitya@gmail.com
Have problem with null values in code approach to database mapping, if the vaule in column is null in database i have problem to fill datagrid, can u give solution?
ReplyDeletethx
I am using VS2012, so far it's working good but in ObjectDataSource1 I am unable to find EmployeeRepository business object not sure why ?? Plz give any suggestion to solve my problem.. Thx
ReplyDeleteRebuild once and try
DeleteDear Venkat ,
ReplyDeleteI'm very happy in this course and thank you for that.and I need your help>i have error (Both DataSource and DataSourceID are defined on 'GridView2'. Remove one definition).
I have been trying to get this step to work and keep failing. I completed all the steps and the code matches, but when I run the program all I get is the "My ASP.NET Application" splash page. Also, the database structure does not get created. I had no issues with videos 1 and 2.
ReplyDeleteHi Venkat,
ReplyDeleteI am getting below Error in EmployeeRepository Class
The type initializer for 'System.Data.Entity.Internal.AppConfig' threw an exception.
Please let me know what could be the issue.. I have tried but not able to fix.
Hi Guys....
ReplyDeleteThose who are not getting the Business Object(EmployeeRepository) to configure the DataSource(ObjectDataSource), Just go and check where you have placed the connectionStrings element in the web.config file.
According to asp.net runtime the element if present within the web.config file then it must be the first child of the root configuration element.
so put your element anywhere after the element. And then build and try to find it in the
Business Objects List...
You have done a very good video here - much better than Microsoft documentation! Very helpful, sir! A couple of questions:
ReplyDelete1) In your EmployeeRepository class, you have the GetDepartments() method, where the first line is: EmployeeDBContext employeeDBContext = new EmployeeDBContext(); This means that the context in created new on each call. Isn't this bad for performance? Would it not be better to show employeeDBContext as an instance variable of the class, set when the EmployeeRepository class is created and destroyed when the class is destroyed?
2) You show connection string in the web.config file. It does not indicate the physical location of the database; it simply uses the default '.'... This doesn't seem like something a real-world app would do. Isn't there some way to determine the location and set the connection string at run time? What connection string would you use to specify that the database should be created in C:\Users\UserName\Documents\ (where UserName is different for each installation of the app)?
An explicit value for the identity column in table 'Employees' can only be specified when a column list is used and IDENTITY_INSERT is ON.
ReplyDeletei am getting this error sir
i got this error -> CREATE DATABASE permission denied in database 'master'.
ReplyDeletefor those who can not find EmployeeRepository, please add
ReplyDeleteusing system.data.entity in classes EmployeeRepository and EmployeeDBContext