Suggested Videos
Part 35 - How to set an item selected when dropdownlist is loaded
Part 36 - Difference between Html.TextBox and Html.TextBoxFor
Part 37 - Generating a radiobuttonlist control in mvc using HTML helpers
In this video we will discuss implementing a checkbox list in asp.net mvc. We will be using table "tblCity" for this demo.
We should be generating a checkbox for each city from the table tblCity.
Sql script to create table tblCity
CREATE TABLE tblCity
(
ID INT IDENTITY PRIMARY KEY,
Name NVARCHAR(100) NOT NULL,
IsSelected BIT NOT NULL
)
Insert into tblCity values ('London', 0)
Insert into tblCity values ('New York', 0)
Insert into tblCity values ('Sydney', 1)
Insert into tblCity values ('Mumbai', 0)
Insert into tblCity values ('Cambridge', 0)
Let's add ADO.NET data model, to retrieve data from database
1. Right click on the "Models" folder > Add > Add New Item
2. From "Add New Item" dialog box, select "ADO.NET Entity Data Model"
3. Set Name=SampleDataModel.edmx and click "Add"
4. On "Entity Data Model" wizard, select "Generate from database" and click "Next"
5. Check "Save entity connection settings in Web.Config as:" checkbox
6. Type "SampleDBContext" as the connection string name and click "Next"
7. On "Choose Your Database Objects" screen, expand "Tables" and select "tblCity" table
8. Type "Models" in "Model Namespace" textbox and click "Finish"
9. Change the entity name from "tblCity" to "City"
Right click on the "Controllers" folder, and add a "HomeController". Include the following 2 namespaces in "HomeController"
using MVCDemo.Models;
using System.Text;
Copy and paste the following code.
[HttpGet]
public ActionResult Index()
{
SampleDBContext db = new SampleDBContext();
return View(db.Cities);
}
[HttpPost]
public string Index(IEnumerable<City> cities)
{
if (cities.Count(x => x.IsSelected) == 0)
{
return "You have not selected any City";
}
else
{
StringBuilder sb = new StringBuilder();
sb.Append("You selected - ");
foreach (City city in cities)
{
if (city.IsSelected)
{
sb.Append(city.Name + ", ");
}
}
sb.Remove(sb.ToString().LastIndexOf(","), 1);
return sb.ToString();
}
}
Right click on the "Views" folder, and a "Home" folder. Right click on the "Home" folder and "EditorTemplates" folder.
Right click on "EditorTemplates" folder > Add > View. In the "Add View" dialog box, set
View Name = City
View Engine = Razor
and click "Add".
Copy and paste the following code in "City.cshtml"
@model MVCDemo.Models.City
@{
ViewBag.Title = "City";
}
@Html.HiddenFor(x => x.ID)
@Html.HiddenFor(x => x.Name)
@Html.CheckBoxFor(x => x.IsSelected)
@Html.DisplayFor(x => x.Name)
Please Note: Put the templates in "Shared" folder, if you want the "Templates", to be available for all the views.
Right click on the "Index" action method in "HomeController", and select "Add View" from the contex menu. Set
View Name = Index
View Engine = Razor and click "Add"
Copy and paste the following code in "Index.cshtml"
@model IEnumerable<MVCDemo.Models.City>
@{
ViewBag.Title = "Index";
}
<div style="font-family:Arial">
<h2>Index</h2>
@using (Html.BeginForm())
{
@Html.EditorForModel()
<br />
<input type="submit" value="Submit" />
}
</div>
Part 35 - How to set an item selected when dropdownlist is loaded
Part 36 - Difference between Html.TextBox and Html.TextBoxFor
Part 37 - Generating a radiobuttonlist control in mvc using HTML helpers
In this video we will discuss implementing a checkbox list in asp.net mvc. We will be using table "tblCity" for this demo.
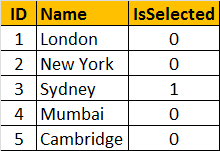
We should be generating a checkbox for each city from the table tblCity.
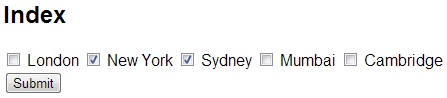
Sql script to create table tblCity
CREATE TABLE tblCity
(
ID INT IDENTITY PRIMARY KEY,
Name NVARCHAR(100) NOT NULL,
IsSelected BIT NOT NULL
)
Insert into tblCity values ('London', 0)
Insert into tblCity values ('New York', 0)
Insert into tblCity values ('Sydney', 1)
Insert into tblCity values ('Mumbai', 0)
Insert into tblCity values ('Cambridge', 0)
Let's add ADO.NET data model, to retrieve data from database
1. Right click on the "Models" folder > Add > Add New Item
2. From "Add New Item" dialog box, select "ADO.NET Entity Data Model"
3. Set Name=SampleDataModel.edmx and click "Add"
4. On "Entity Data Model" wizard, select "Generate from database" and click "Next"
5. Check "Save entity connection settings in Web.Config as:" checkbox
6. Type "SampleDBContext" as the connection string name and click "Next"
7. On "Choose Your Database Objects" screen, expand "Tables" and select "tblCity" table
8. Type "Models" in "Model Namespace" textbox and click "Finish"
9. Change the entity name from "tblCity" to "City"
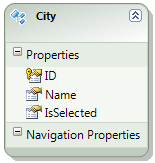
Right click on the "Controllers" folder, and add a "HomeController". Include the following 2 namespaces in "HomeController"
using MVCDemo.Models;
using System.Text;
Copy and paste the following code.
[HttpGet]
public ActionResult Index()
{
SampleDBContext db = new SampleDBContext();
return View(db.Cities);
}
[HttpPost]
public string Index(IEnumerable<City> cities)
{
if (cities.Count(x => x.IsSelected) == 0)
{
return "You have not selected any City";
}
else
{
StringBuilder sb = new StringBuilder();
sb.Append("You selected - ");
foreach (City city in cities)
{
if (city.IsSelected)
{
sb.Append(city.Name + ", ");
}
}
sb.Remove(sb.ToString().LastIndexOf(","), 1);
return sb.ToString();
}
}
Right click on the "Views" folder, and a "Home" folder. Right click on the "Home" folder and "EditorTemplates" folder.
Right click on "EditorTemplates" folder > Add > View. In the "Add View" dialog box, set
View Name = City
View Engine = Razor
and click "Add".
Copy and paste the following code in "City.cshtml"
@model MVCDemo.Models.City
@{
ViewBag.Title = "City";
}
@Html.HiddenFor(x => x.ID)
@Html.HiddenFor(x => x.Name)
@Html.CheckBoxFor(x => x.IsSelected)
@Html.DisplayFor(x => x.Name)
Please Note: Put the templates in "Shared" folder, if you want the "Templates", to be available for all the views.
Right click on the "Index" action method in "HomeController", and select "Add View" from the contex menu. Set
View Name = Index
View Engine = Razor and click "Add"
Copy and paste the following code in "Index.cshtml"
@model IEnumerable<MVCDemo.Models.City>
@{
ViewBag.Title = "Index";
}
<div style="font-family:Arial">
<h2>Index</h2>
@using (Html.BeginForm())
{
@Html.EditorForModel()
<br />
<input type="submit" value="Submit" />
}
</div>
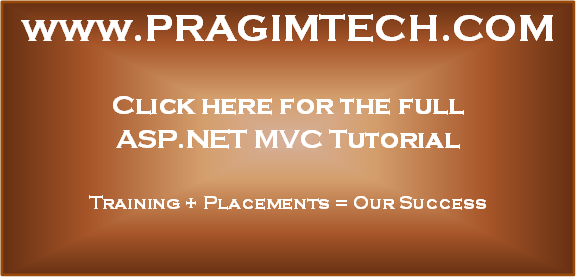
How to get the selected data on another page .
ReplyDeletevarious way to send selected data to another page, 1- Using FormCollection. 2- Use data model object.
DeleteHow to use this helper in Create page?and how to display what i select item?
ReplyDeletehow to pass collection of model class from view to controller without using editor templatpe?
ReplyDeleteYou can loop with for in your view. Use IList instead of IEnumerable
DeleteGetting an error like con not convert implicitly the type bool? to bool.
ReplyDeletetype isselected.value for convert implicity typeto bool
ReplyDeletehow to enter the checkboxlist value to database using entityframework
ReplyDeletei am very confused
ReplyDelete(1) what happens when we check the checkbox?
(2) how isSelected is updated ?
(3) when the form is posted dowe receive all checkboxes or only the selected ones?
(4)In foreach loop we are checking
if (city.isSelected)
{
sb.Append(city.Name + ", ");
}
how isSeleced is updated??????
When we check the check boxes and click on submit, the form is posted back to the server and the Index action method decorated with Http post attribute gets executed. It receives the list of checked check boxes and hence the name of the cities associated with them. Int the output stream it returns the names.
DeleteFrom where the Cities Property comes in this code and all other parts :-
ReplyDelete[HttpGet]
public ActionResult Index()
{
SampleDBContext db = new SampleDBContext();
return View(db.Cities);
}
Instance of data context class connects with the model containing the 'City' class generated from the tblCity database table and it has got all the table coloumns as properties of the 'City' data model class
Deletei get out 1234 with submit button . after all the code ?
ReplyDeletedo not get checkbox , is there is any solution for that
hii venkat i wanna functionality like , if i submitted the from using Submit button okay , but if some one does back the page using browser then i wanna first time displayed checkboxes.
ReplyDeleteHi Venkat,
ReplyDeleteCould you please let me know how to post the data back to data base from check box, I know you showed us to to display what checked boxes user check on UI, what if we want to save that data how do we do that, I would really appreciate if you could have something information for me.
Thanks
Ali
I am getting error in this line of code "@Html.CheckBoxFor(x=>x.IsSelected)".
ReplyDeleteGetting red square line under “x.IsSelected”.
i.e
"Cannot implicitly convert type 'bool?' to 'bool'. An explicit conversion exists (are you missing a cast?)
Cannot convert lambda expression to intended delegate type because some of the return types in the block are not implicitly convertible to the delegate return type"
Red squire line
Check your table entries, IsSelected column should not have null entries.
DeleteHow we handle this if the column has null entries?
DeleteI got output as 12345. template is not loaded, I tried
ReplyDelete@Html.EditorForModel("City") with parameter. its not working. help me in this.
Check the view name in EditorsTemplates. It must equals to model view.
Deleteyour folder name should be like this "EditorTemplates" it will solve the issue
Deleteif you are getting output like 12345, means your editortepmlate folder name is not correct. Please update it with EditorTemplates
ReplyDeleteIf you are getting that issue bcz when you are adding a view to index page don't select dropdownlist (SampleDbContext) your issues will be resolved.
Deletehow to implement in a class because there is multiple properties
ReplyDeletehow to save the values in the checkbox to database
ReplyDelete