Suggested Videos
Part 32 - How does a controller find a view
Part 33 - Html helpers
Part 34 - Generating a dropdownlist control in mvc using HTML helpers
In this video we will discuss, how to set an item selected when an asp.net mvc dropdownlist options are loaded from a database table. Please watch Part 34 before proceeding.
To have the "IT" department selected, when the departments are loaded from tblDepartment table, use the following overloaded constructor of "SelectList" class. Notice that we are passing a value of "1" for "selectedValue" parameter.
ViewBag.Departments = new SelectList(db.Departments, "Id", "Name", "1");
If you run the application at this point, "IT" department will be selected, when the dropdownlist is rendered. The downside of hard-coding the "selectedValue" in code is that, application code needs to be modified, if we want "HR" department to be selected instead of "IT". So every time there is a change in requirement, we need to change the application code.
Let's now discuss, the steps required to drive the selection of an item in the dropdownlist using a column in tblDepartment table.
Step 1: Add "IsSelected" bit column to tblDepartment table
ALTER TABLE tblDepartment
ADD IsSelected BIT
Step 2: At this point, this column will be null for all the rows in tblDepartment table. If we want IT department to be selected by default when the dropdownlist is loaded, set "IsSelected=1" for the "IT" department row.
Update tblDepartment Set IsSelected = 1 Where Id = 2
Step 3: Refresh ADO.NET Entity Data Model
Step 4: Finally, make the following changes to the "Index()" action method in "HomeController" class.
public ActionResult Index()
{
SampleDBContext db = new SampleDBContext();
List<SelectListItem> selectListItems = new List<SelectListItem>();
foreach (Department department in db.Departments)
{
SelectListItem selectListItem = new SelectListItem
{
Text = department.Name,
Value = department.Id.ToString(),
Selected = department.IsSelected.HasValue ? department.IsSelected.Value : false
};
selectListItems.Add(selectListItem);
}
ViewBag.Departments = selectListItems;
return View();
}
Run the application and notice that, "IT" department is selected, when the dropdownlist is loaded.
If you now want "HR" department to be selected, instead of "IT", set "IsSelected=1" for "HR" department and "IsSelected=0" for "IT" department.
Update tblDepartment Set IsSelected = 1 Where Id = 2
Update tblDepartment Set IsSelected = 0 Where Id = 1
Part 32 - How does a controller find a view
Part 33 - Html helpers
Part 34 - Generating a dropdownlist control in mvc using HTML helpers
In this video we will discuss, how to set an item selected when an asp.net mvc dropdownlist options are loaded from a database table. Please watch Part 34 before proceeding.
To have the "IT" department selected, when the departments are loaded from tblDepartment table, use the following overloaded constructor of "SelectList" class. Notice that we are passing a value of "1" for "selectedValue" parameter.
ViewBag.Departments = new SelectList(db.Departments, "Id", "Name", "1");
If you run the application at this point, "IT" department will be selected, when the dropdownlist is rendered. The downside of hard-coding the "selectedValue" in code is that, application code needs to be modified, if we want "HR" department to be selected instead of "IT". So every time there is a change in requirement, we need to change the application code.
Let's now discuss, the steps required to drive the selection of an item in the dropdownlist using a column in tblDepartment table.
Step 1: Add "IsSelected" bit column to tblDepartment table
ALTER TABLE tblDepartment
ADD IsSelected BIT
Step 2: At this point, this column will be null for all the rows in tblDepartment table. If we want IT department to be selected by default when the dropdownlist is loaded, set "IsSelected=1" for the "IT" department row.
Update tblDepartment Set IsSelected = 1 Where Id = 2
Step 3: Refresh ADO.NET Entity Data Model
Step 4: Finally, make the following changes to the "Index()" action method in "HomeController" class.
public ActionResult Index()
{
SampleDBContext db = new SampleDBContext();
List<SelectListItem> selectListItems = new List<SelectListItem>();
foreach (Department department in db.Departments)
{
SelectListItem selectListItem = new SelectListItem
{
Text = department.Name,
Value = department.Id.ToString(),
Selected = department.IsSelected.HasValue ? department.IsSelected.Value : false
};
selectListItems.Add(selectListItem);
}
ViewBag.Departments = selectListItems;
return View();
}
Run the application and notice that, "IT" department is selected, when the dropdownlist is loaded.
If you now want "HR" department to be selected, instead of "IT", set "IsSelected=1" for "HR" department and "IsSelected=0" for "IT" department.
Update tblDepartment Set IsSelected = 1 Where Id = 2
Update tblDepartment Set IsSelected = 0 Where Id = 1
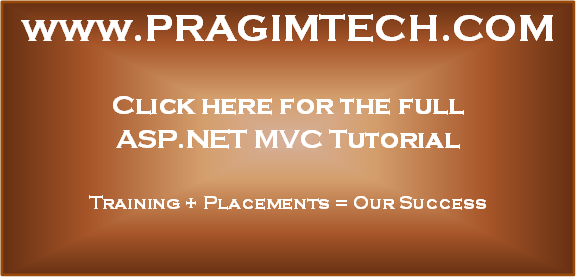
SelectedlistItem hasValue and value property showing error
ReplyDeleteadd public bool? IsSelected{get;set;} in Departemnt class
Deleteplz plz solve tis issue
ReplyDeleteIf you want only the distinct data how do you do that?
ReplyDeleteSelectedlistItem hasValue and value property showing error how to set property of the hashvalue
ReplyDeleteYou can define
ReplyDeletepublic partial class Department
{
public int dept_id { get; set; }
public string dept_name { get; set; }
public int? isSelected { get; set; }
}
in Department.cs