Suggested Videos
Part 34 - Generating a dropdownlist control in mvc using HTML helpers
Part 35 - How to set an item selected when dropdownlist is loaded
Part 36 - Difference between Html.TextBox and Html.TextBoxFor
In this video, we will discuss, generating a radiobuttonlist in mvc using Html.RadioButtonFor helper. Please watch Part 36 before proceeding.
Right click on the "Models" folder and add a class file with "name=Company.cs". Copy and paste the following code.
public class Company
{
public string SelectedDepartment { get; set; }
public List<Department> Departments
{
get
{
SampleDBContext db = new SampleDBContext();
return db.Departments.ToList();
}
}
}
Copy and paste the following 2 "Index" action methods in HomeController class.
[HttpGet]
public ActionResult Index()
{
Company company = new Company();
return View(company);
}
[HttpPost]
public string Index(Company company)
{
if (string.IsNullOrEmpty(company.SelectedDepartment))
{
return "You did not select any department";
}
else
{
return "You selected department with ID = " + company.SelectedDepartment;
}
}
Right click on the "Index" action method in "HomeController" and add a view with "name=Index". Copy and paste the following code.
@model MVCDemo.Models.Company
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
@using (Html.BeginForm())
{
foreach (var department in Model.Departments)
{
@Html.RadioButtonFor(m => m.SelectedDepartment, department.Id) @department.Name
}
<br />
<br />
<input type="submit" value="Submit" />
}
Run the application and click on "Submit" without selecting any department. Notice that, you get a message stating you have not selected any department. On the other hand, select a department and click "Submit". The selected department ID must be displayed.
Part 34 - Generating a dropdownlist control in mvc using HTML helpers
Part 35 - How to set an item selected when dropdownlist is loaded
Part 36 - Difference between Html.TextBox and Html.TextBoxFor
In this video, we will discuss, generating a radiobuttonlist in mvc using Html.RadioButtonFor helper. Please watch Part 36 before proceeding.
Right click on the "Models" folder and add a class file with "name=Company.cs". Copy and paste the following code.
public class Company
{
public string SelectedDepartment { get; set; }
public List<Department> Departments
{
get
{
SampleDBContext db = new SampleDBContext();
return db.Departments.ToList();
}
}
}
Copy and paste the following 2 "Index" action methods in HomeController class.
[HttpGet]
public ActionResult Index()
{
Company company = new Company();
return View(company);
}
[HttpPost]
public string Index(Company company)
{
if (string.IsNullOrEmpty(company.SelectedDepartment))
{
return "You did not select any department";
}
else
{
return "You selected department with ID = " + company.SelectedDepartment;
}
}
Right click on the "Index" action method in "HomeController" and add a view with "name=Index". Copy and paste the following code.
@model MVCDemo.Models.Company
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
@using (Html.BeginForm())
{
foreach (var department in Model.Departments)
{
@Html.RadioButtonFor(m => m.SelectedDepartment, department.Id) @department.Name
}
<br />
<br />
<input type="submit" value="Submit" />
}
Run the application and click on "Submit" without selecting any department. Notice that, you get a message stating you have not selected any department. On the other hand, select a department and click "Submit". The selected department ID must be displayed.
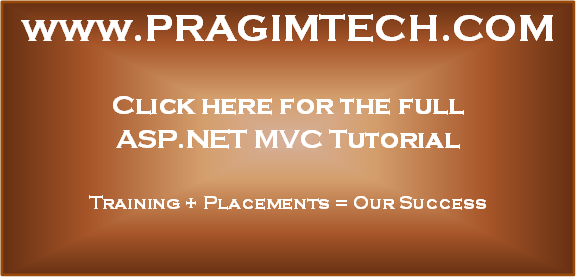
hi Please let me know anyone ,how can we display the selected values in the same page(it means ,i have to display radiobuttons and also the selected value below)
ReplyDeleteyou can make an ajax call to partial view which can display the value.
ReplyDeleteNice Tutorial,
ReplyDeleteBy doing so we are getting all the input radio control ids value same that is SelectedDepartment of all 3 radio button, but in a html id should be unique because otherwise w3validation throws warning , so how to achieve this i want 3 radio button name will be same but id should be unique in whole html instance , please help to fix it , thanks
FROM WHERE THE HELL "SelectedDepartment" property gets the value where it was given the value VERY VERY Confused please reply..... [sorry for my english]
ReplyDeleteWhen I click on the Submit button, the app fails with "No parameterless constructor defined for this object.". It looks like the company object is not being returned when the post Index method is invoked. If I replace "public string Index(Company company)" with "public string Index(object obj)" and then within the method, code "Company company = obj as company;", then I can see that "obj" is null. Has anyone else gotten this? I could use some help with this.
ReplyDeletei face same problem , you should add parameterless constructor to Company Class like below :
Deletepublic Company(){
}