Suggested Videos
Part 4 - XMLDataSource in asp.net
Part 5 - Using XSLTTransform with xmldatasource control
Part 6 - AccessDataSource in asp.net
We will be using tblEmployee table for this demo. Use the script below to create employees table and populate it with sample data.
CREATE TABLE [tblEmployee]
(
[EmployeeId] [int] IDENTITY PRIMARY KEY,
[FirstName] [nvarchar](50),
[LastName] [nvarchar](50),
[DateOfBirth] [datetime],
[AnnualSalary] [int],
[Gender] [nvarchar](50),
[DepartmentName] [nvarchar](50)
)
Insert into tblEmployee values
('John','Johnson','08/10/1982',55000,'Male','IT')
Insert into tblEmployee values
('Pam','Roberts','11/11/1979',62000,'Female','HR')
Insert into tblEmployee values
('David','John','01/15/1980',45000,'Male','IT')
Insert into tblEmployee values
('Rose','Mary','08/18/1967',78000,'Female','Payroll')
Insert into tblEmployee values
('Mark','Mell','12/10/1974',69000,'Male','HR')
Use "SqlDataSource" control to retrieve data from the database and bind it to gridview control. The HTML of the webform, is shown below.
<div style="font-family: Arial">
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:DBConnectionString %>"
SelectCommand="SELECT * FROM [tblEmployee]"></asp:SqlDataSource>
<br />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
DataKeyNames="EmployeeId" DataSourceID="SqlDataSource1">
<Columns>
<asp:BoundField DataField="EmployeeId" HeaderText="EmployeeId"
InsertVisible="False" ReadOnly="True" SortExpression="EmployeeId" />
<asp:BoundField DataField="FirstName" HeaderText="FirstName"
SortExpression="FirstName" />
<asp:BoundField DataField="LastName" HeaderText="LastName"
SortExpression="LastName" />
<asp:BoundField DataField="DateOfBirth" HeaderText="DateOfBirth"
SortExpression="DateOfBirth" />
<asp:BoundField DataField="AnnualSalary" HeaderText="AnnualSalary"
SortExpression="AnnualSalary" />
<asp:BoundField DataField="Gender" HeaderText="Gender"
SortExpression="Gender" />
<asp:BoundField DataField="DepartmentName" HeaderText="DepartmentName"
SortExpression="DepartmentName" />
</Columns>
</asp:GridView>
</div>
Notice that, by default, all the columns from the table are displayed in the gridview control. For example, I don't want to display EmployeeId column in the gridview control. There are 3 ways to achieve this
1. You can modify "SqlDataSource" control "SELECT" query, to include only the columns that you need.
2. Remove the "BoundField" column from gridview control that displays, EmployeeId
3. Set visibility property to false on the "BoundField" column from gridview control that displays, EmployeeId
Notice the column headers in the gridview control. They are same as the column names in the table. For example, we want a space between First and Name in FirstName. Similary, we want spaces for LastName, DateOfBirth, AnnualSalary and DepartmentName.
FirstName = First Name
LastName = Last Name
DateOfBirth = Date Of Birth
AnnualSalary = Annual Salary
DepartmentName = Department Name
To achieve this, change "HeaderText" property of the respective column, in the gridview control.
When you run the application, DateOfBirth column displays both Date and Time. I want only the "date" to be displayed. We can very easily achieve this using DataFormatString property as shown below. We have set DataFormatString="{0:d}", which will display short date pattern. Setting DataFormatString="{0:D}", will display long date pattern.
<asp:BoundField DataField="DateOfBirth" DataFormatString="{0:d}"
HeaderText="DateOfBirth" SortExpression="DateOfBirth" />
For the complete list of "Standard Date and Time Format Strings", please visit MSDN link below.
http://msdn.microsoft.com/en-gb/library/az4se3k1.aspx
For the annual salary column, I want to display currency symbol. For example, depending on your application requirement, you may want to display, either United States dollar ($), Great British Pound(£) or Indian Rupee Symbol. To achieve this use currency format string "0:c" as shown below. If you want to display 2 decimal places using "0:c2"
<asp:BoundField DataField="AnnualSalary" DataFormatString="{0:c}"
HeaderText="AnnualSalary" SortExpression="AnnualSalary" />
For the complete list of "Standard Numeric Format Strings", please visit MSDN link below.
http://msdn.microsoft.com/en-gb/library/dwhawy9k.aspx
Currency symbol will be displayed, based on the default culture setting. For example,
If your default culture setting is "en-US", United States Dollar symbol will be displayed
If your default culture setting is "en-GB", Great British Pound symbol will be displayed
If your default culture setting is "en-IN", Indian Rupee symbol will be displayed
Culture can be specified in web.config as shown below. This setting will be applicable for all pages in the asp.net web application.
<system.web>
<globalization culture="en-US"/>
</system.web>
It is possible to override culture setting on a page-by-page basis. To set the culture declaratively in the aspx page, use "Culture" attribute of the "Page" directive as shown below.
<%@ Page Culture="en-GB"
To override the culture programmatically
System.Threading.Thread.CurrentThread.CurrentCulture = new System.Globalization.CultureInfo("en-GB");
Part 4 - XMLDataSource in asp.net
Part 5 - Using XSLTTransform with xmldatasource control
Part 6 - AccessDataSource in asp.net
We will be using tblEmployee table for this demo. Use the script below to create employees table and populate it with sample data.
CREATE TABLE [tblEmployee]
(
[EmployeeId] [int] IDENTITY PRIMARY KEY,
[FirstName] [nvarchar](50),
[LastName] [nvarchar](50),
[DateOfBirth] [datetime],
[AnnualSalary] [int],
[Gender] [nvarchar](50),
[DepartmentName] [nvarchar](50)
)
Insert into tblEmployee values
('John','Johnson','08/10/1982',55000,'Male','IT')
Insert into tblEmployee values
('Pam','Roberts','11/11/1979',62000,'Female','HR')
Insert into tblEmployee values
('David','John','01/15/1980',45000,'Male','IT')
Insert into tblEmployee values
('Rose','Mary','08/18/1967',78000,'Female','Payroll')
Insert into tblEmployee values
('Mark','Mell','12/10/1974',69000,'Male','HR')
Use "SqlDataSource" control to retrieve data from the database and bind it to gridview control. The HTML of the webform, is shown below.
<div style="font-family: Arial">
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:DBConnectionString %>"
SelectCommand="SELECT * FROM [tblEmployee]"></asp:SqlDataSource>
<br />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
DataKeyNames="EmployeeId" DataSourceID="SqlDataSource1">
<Columns>
<asp:BoundField DataField="EmployeeId" HeaderText="EmployeeId"
InsertVisible="False" ReadOnly="True" SortExpression="EmployeeId" />
<asp:BoundField DataField="FirstName" HeaderText="FirstName"
SortExpression="FirstName" />
<asp:BoundField DataField="LastName" HeaderText="LastName"
SortExpression="LastName" />
<asp:BoundField DataField="DateOfBirth" HeaderText="DateOfBirth"
SortExpression="DateOfBirth" />
<asp:BoundField DataField="AnnualSalary" HeaderText="AnnualSalary"
SortExpression="AnnualSalary" />
<asp:BoundField DataField="Gender" HeaderText="Gender"
SortExpression="Gender" />
<asp:BoundField DataField="DepartmentName" HeaderText="DepartmentName"
SortExpression="DepartmentName" />
</Columns>
</asp:GridView>
</div>
Notice that, by default, all the columns from the table are displayed in the gridview control. For example, I don't want to display EmployeeId column in the gridview control. There are 3 ways to achieve this
1. You can modify "SqlDataSource" control "SELECT" query, to include only the columns that you need.
2. Remove the "BoundField" column from gridview control that displays, EmployeeId
3. Set visibility property to false on the "BoundField" column from gridview control that displays, EmployeeId
Notice the column headers in the gridview control. They are same as the column names in the table. For example, we want a space between First and Name in FirstName. Similary, we want spaces for LastName, DateOfBirth, AnnualSalary and DepartmentName.
FirstName = First Name
LastName = Last Name
DateOfBirth = Date Of Birth
AnnualSalary = Annual Salary
DepartmentName = Department Name
To achieve this, change "HeaderText" property of the respective column, in the gridview control.
When you run the application, DateOfBirth column displays both Date and Time. I want only the "date" to be displayed. We can very easily achieve this using DataFormatString property as shown below. We have set DataFormatString="{0:d}", which will display short date pattern. Setting DataFormatString="{0:D}", will display long date pattern.
<asp:BoundField DataField="DateOfBirth" DataFormatString="{0:d}"
HeaderText="DateOfBirth" SortExpression="DateOfBirth" />
For the complete list of "Standard Date and Time Format Strings", please visit MSDN link below.
http://msdn.microsoft.com/en-gb/library/az4se3k1.aspx
For the annual salary column, I want to display currency symbol. For example, depending on your application requirement, you may want to display, either United States dollar ($), Great British Pound(£) or Indian Rupee Symbol. To achieve this use currency format string "0:c" as shown below. If you want to display 2 decimal places using "0:c2"
<asp:BoundField DataField="AnnualSalary" DataFormatString="{0:c}"
HeaderText="AnnualSalary" SortExpression="AnnualSalary" />
For the complete list of "Standard Numeric Format Strings", please visit MSDN link below.
http://msdn.microsoft.com/en-gb/library/dwhawy9k.aspx
Currency symbol will be displayed, based on the default culture setting. For example,
If your default culture setting is "en-US", United States Dollar symbol will be displayed
If your default culture setting is "en-GB", Great British Pound symbol will be displayed
If your default culture setting is "en-IN", Indian Rupee symbol will be displayed
Culture can be specified in web.config as shown below. This setting will be applicable for all pages in the asp.net web application.
<system.web>
<globalization culture="en-US"/>
</system.web>
It is possible to override culture setting on a page-by-page basis. To set the culture declaratively in the aspx page, use "Culture" attribute of the "Page" directive as shown below.
<%@ Page Culture="en-GB"
To override the culture programmatically
System.Threading.Thread.CurrentThread.CurrentCulture = new System.Globalization.CultureInfo("en-GB");
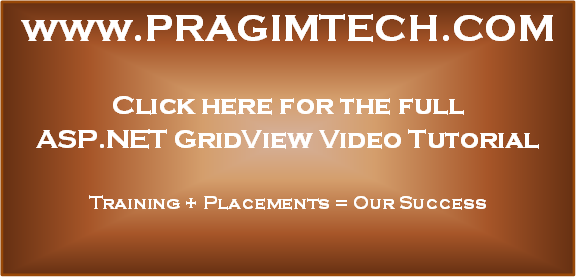
Hi Venkat,
ReplyDeleteHow can we have multiple cultures for a single page.For e.g. Each row of the grid displays a record for a person belonging to a different country ???
Thanks,
Rohan
Hi Rohan, this is a very good question. In fact, this is a frequently asked interview question as well. Thanks for your question. If you want to change any thing on a row-by-row basis, then RowDataBound() event of the gridview control can be used. If this does not make sense now, please don't worry, I will record and upload a video very shortly.
Deletehii sir how to get new currency symbol my system is showing old currency symbol , that question ask by an interviewer . please tell me
ReplyDelete