Suggested Videos
Part 2 - Data source controls in asp.net
Part 3 - Object datasource in asp.net
Part 4 - XMLDataSource in asp.net
In Part 4 of the asp.net gridview tutorial, we discussed about binding an xml file to a gridview control using xmldatasource control. XmlDataSource control did not work as expected, when the xml file, contained child xml nodes. We had to manually rewrite the entire xml file using attributes instead. However, manually rewriting the xml file is laborious and error prone, especially if the file is very large.
We can use an XSLT transform file, to dynamically convert the child xml nodes to attributes. Countries xml with child nodes is shown below. Create an asp.net web application. Add a folder with name=Data to the project. Right click on the "Data" folder and add an XML file with name=Countries.xml.
Copy and paste the following XML in Countries.xml
<Countries>
<Country>
<Id>101</Id>
<Name>India</Name>
<Continent>Asia</Continent>
</Country>
<Country>
<Id>102</Id>
<Name>China</Name>
<Continent>Asia</Continent>
</Country>
<Country>
<Id>103</Id>
<Name>Frnace</Name>
<Continent>Europe</Continent>
</Country>
<Country>
<Id>104</Id>
<Name>United Kingdom</Name>
<Continent>Europe</Continent>
</Country>
<Country>
<Id>105</Id>
<Name>United State of America</Name>
<Continent>North America</Continent>
</Country>
</Countries>
We want to transform the above xml using XSLT. The output should be as shown below.
<Countries>
<Country Id="101" Name="India" Continent="Asia"/>
<Country Id="102" Name="China" Continent="Asia"/>
<Country Id="103" Name="Frnace" Continent="Europe"/>
<Country Id="104" Name="United Kingdom" Continent="Europe"/>
<Country Id="105" Name="United State of America" Continent="North America"/>
</Countries>
To achieve this, right click on "Data" folder and add an XSLT file with name=CountriesXSLT.xslt. Copy and paste the following code in CountriesXSLT.xslt file.
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="Countries">
<Countries>
<xsl:apply-templates/>
</Countries>
</xsl:template>
<xsl:template match="Country">
<Country>
<xsl:for-each select="*">
<xsl:attribute name="{name()}">
<xsl:value-of select="text()"/>
</xsl:attribute>
</xsl:for-each>
</Country>
</xsl:template>
</xsl:stylesheet>
Drag "XmlDataSource" control from the toolbox, onto WebForm1.aspx. Click on the smart tag button of the "XmlDataSource" control, and then click on "Configure Data Source". Click on "Browse" button next to "Data file" textbox and select "Countries.xml" from "Data" folder. Now, click on "Browse" button next to "Transform file" textbox and select "CountriesXSLT.xslt" from "Data" folder. Finally click OK.
Now, drag and drop a gridview control on WebForm1.aspx. Click on smart tag button on the gridview control, and select "XmlDataSource1" from "Choose Data Source" dropdownlist. Notice that, the gridview control displays xml data as expected.
If you want to display the same xml data in a gridview control without using xslt transform, then load the xml data into a dataset and then bind it to the gridview control.
DataSet ds = new DataSet();
ds.ReadXml(Server.MapPath("~/Data/Countries.xml"));
GridView1.DataSource = ds;
GridView1.DataBind();
Part 2 - Data source controls in asp.net
Part 3 - Object datasource in asp.net
Part 4 - XMLDataSource in asp.net
In Part 4 of the asp.net gridview tutorial, we discussed about binding an xml file to a gridview control using xmldatasource control. XmlDataSource control did not work as expected, when the xml file, contained child xml nodes. We had to manually rewrite the entire xml file using attributes instead. However, manually rewriting the xml file is laborious and error prone, especially if the file is very large.
We can use an XSLT transform file, to dynamically convert the child xml nodes to attributes. Countries xml with child nodes is shown below. Create an asp.net web application. Add a folder with name=Data to the project. Right click on the "Data" folder and add an XML file with name=Countries.xml.
Copy and paste the following XML in Countries.xml
<Countries>
<Country>
<Id>101</Id>
<Name>India</Name>
<Continent>Asia</Continent>
</Country>
<Country>
<Id>102</Id>
<Name>China</Name>
<Continent>Asia</Continent>
</Country>
<Country>
<Id>103</Id>
<Name>Frnace</Name>
<Continent>Europe</Continent>
</Country>
<Country>
<Id>104</Id>
<Name>United Kingdom</Name>
<Continent>Europe</Continent>
</Country>
<Country>
<Id>105</Id>
<Name>United State of America</Name>
<Continent>North America</Continent>
</Country>
</Countries>
We want to transform the above xml using XSLT. The output should be as shown below.
<Countries>
<Country Id="101" Name="India" Continent="Asia"/>
<Country Id="102" Name="China" Continent="Asia"/>
<Country Id="103" Name="Frnace" Continent="Europe"/>
<Country Id="104" Name="United Kingdom" Continent="Europe"/>
<Country Id="105" Name="United State of America" Continent="North America"/>
</Countries>
To achieve this, right click on "Data" folder and add an XSLT file with name=CountriesXSLT.xslt. Copy and paste the following code in CountriesXSLT.xslt file.
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="Countries">
<Countries>
<xsl:apply-templates/>
</Countries>
</xsl:template>
<xsl:template match="Country">
<Country>
<xsl:for-each select="*">
<xsl:attribute name="{name()}">
<xsl:value-of select="text()"/>
</xsl:attribute>
</xsl:for-each>
</Country>
</xsl:template>
</xsl:stylesheet>
Drag "XmlDataSource" control from the toolbox, onto WebForm1.aspx. Click on the smart tag button of the "XmlDataSource" control, and then click on "Configure Data Source". Click on "Browse" button next to "Data file" textbox and select "Countries.xml" from "Data" folder. Now, click on "Browse" button next to "Transform file" textbox and select "CountriesXSLT.xslt" from "Data" folder. Finally click OK.
Now, drag and drop a gridview control on WebForm1.aspx. Click on smart tag button on the gridview control, and select "XmlDataSource1" from "Choose Data Source" dropdownlist. Notice that, the gridview control displays xml data as expected.
If you want to display the same xml data in a gridview control without using xslt transform, then load the xml data into a dataset and then bind it to the gridview control.
DataSet ds = new DataSet();
ds.ReadXml(Server.MapPath("~/Data/Countries.xml"));
GridView1.DataSource = ds;
GridView1.DataBind();
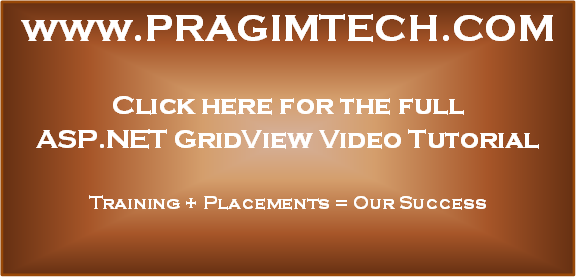
No comments:
Post a Comment
It would be great if you can help share these free resources