Suggested Videos
Part 5 - Using XSLTTransform with xmldatasource control
Part 6 - AccessDataSource in asp.net
Part 7 - Formatting asp.net gridview control
Please watch Part 7 of the asp.net gridview tutorial before proceeding with this video.
This video is in response to one of my youtube subscriber's question. The subscriber's question is How can we have multiple cultures for a single page. For example, each row of the grid displays a record for a person belonging to a different country.
Let us understand this with an example. I have a table, tblEmployee as shown below.
When, this data is displayed in a gridview control, we want the country specific currency symbol to be displayed, next to their salary. The output should be as shown below.
HTML of WebForm1.aspx:
<div style="font-family:Arial">
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:DBConnectionString %>"
SelectCommand="SELECT * FROM [tblEmployee]"></asp:SqlDataSource>
<br />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
DataKeyNames="EmployeeId" DataSourceID="SqlDataSource1"
BackColor="#DEBA84" BorderColor="#DEBA84" BorderStyle="None" BorderWidth="1px"
CellPadding="3" CellSpacing="2" onrowdatabound="GridView1_RowDataBound">
<Columns>
<asp:BoundField DataField="EmployeeId" HeaderText="EmployeeId"
InsertVisible="False" ReadOnly="True" SortExpression="EmployeeId" />
<asp:BoundField DataField="FirstName" HeaderText="FirstName"
SortExpression="FirstName" />
<asp:BoundField DataField="AnnualSalary" HeaderText="AnnualSalary"
SortExpression="AnnualSalary"/>
<asp:BoundField DataField="Country" HeaderText="Country"
SortExpression="Country" />
</Columns>
<FooterStyle BackColor="#F7DFB5" ForeColor="#8C4510" />
<HeaderStyle BackColor="#A55129" Font-Bold="True" ForeColor="White" />
<PagerStyle ForeColor="#8C4510" HorizontalAlign="Center" />
<RowStyle BackColor="#FFF7E7" ForeColor="#8C4510" />
<SelectedRowStyle BackColor="#738A9C" Font-Bold="True" ForeColor="White" />
<SortedAscendingCellStyle BackColor="#FFF1D4" />
<SortedAscendingHeaderStyle BackColor="#B95C30" />
<SortedDescendingCellStyle BackColor="#F1E5CE" />
<SortedDescendingHeaderStyle BackColor="#93451F" />
</asp:GridView>
</div>
To achieve this, we can make use of RowDataBound event of GridView control. RowDataBound event is raised when a record in the datasource is bound to a row in gridview control. Please note that in RowDataBound event, we are first checking, if the row, that is being bound, is a datarow. This event is also raised for other row types like Header, Footer etc.
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
// Check if the row that is being bound, is a datarow
if (e.Row.RowType == DataControlRowType.DataRow)
{
if (e.Row.Cells[3].Text == "US")
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string formattedString = string.Format(new System.Globalization.CultureInfo("en-US"), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
else if (e.Row.Cells[3].Text == "UK")
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string formattedString = string.Format(new System.Globalization.CultureInfo("en-GB"), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
else if (e.Row.Cells[3].Text == "India")
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string formattedString = string.Format(new System.Globalization.CultureInfo("en-IN"), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
else if (e.Row.Cells[3].Text == "South Africa")
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string formattedString = string.Format(new System.Globalization.CultureInfo("en-ZA"), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
else if (e.Row.Cells[3].Text == "Malaysia")
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string formattedString = string.Format(new System.Globalization.CultureInfo("en-MY"), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
}
}
Use the code below, to get the list of all supported cultures in asp.net
foreach (CultureInfo ci in CultureInfo.GetCultures(CultureTypes.AllCultures))
{
Response.Write(ci.Name + " => " + ci.DisplayName + "<br/>");
}
The list of countries and their currencies can be found at the following wikipedia article
http://en.wikipedia.org/wiki/List_of_circulating_currencies
Part 5 - Using XSLTTransform with xmldatasource control
Part 6 - AccessDataSource in asp.net
Part 7 - Formatting asp.net gridview control
Please watch Part 7 of the asp.net gridview tutorial before proceeding with this video.
This video is in response to one of my youtube subscriber's question. The subscriber's question is How can we have multiple cultures for a single page. For example, each row of the grid displays a record for a person belonging to a different country.
Let us understand this with an example. I have a table, tblEmployee as shown below.

When, this data is displayed in a gridview control, we want the country specific currency symbol to be displayed, next to their salary. The output should be as shown below.

HTML of WebForm1.aspx:
<div style="font-family:Arial">
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:DBConnectionString %>"
SelectCommand="SELECT * FROM [tblEmployee]"></asp:SqlDataSource>
<br />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
DataKeyNames="EmployeeId" DataSourceID="SqlDataSource1"
BackColor="#DEBA84" BorderColor="#DEBA84" BorderStyle="None" BorderWidth="1px"
CellPadding="3" CellSpacing="2" onrowdatabound="GridView1_RowDataBound">
<Columns>
<asp:BoundField DataField="EmployeeId" HeaderText="EmployeeId"
InsertVisible="False" ReadOnly="True" SortExpression="EmployeeId" />
<asp:BoundField DataField="FirstName" HeaderText="FirstName"
SortExpression="FirstName" />
<asp:BoundField DataField="AnnualSalary" HeaderText="AnnualSalary"
SortExpression="AnnualSalary"/>
<asp:BoundField DataField="Country" HeaderText="Country"
SortExpression="Country" />
</Columns>
<FooterStyle BackColor="#F7DFB5" ForeColor="#8C4510" />
<HeaderStyle BackColor="#A55129" Font-Bold="True" ForeColor="White" />
<PagerStyle ForeColor="#8C4510" HorizontalAlign="Center" />
<RowStyle BackColor="#FFF7E7" ForeColor="#8C4510" />
<SelectedRowStyle BackColor="#738A9C" Font-Bold="True" ForeColor="White" />
<SortedAscendingCellStyle BackColor="#FFF1D4" />
<SortedAscendingHeaderStyle BackColor="#B95C30" />
<SortedDescendingCellStyle BackColor="#F1E5CE" />
<SortedDescendingHeaderStyle BackColor="#93451F" />
</asp:GridView>
</div>
To achieve this, we can make use of RowDataBound event of GridView control. RowDataBound event is raised when a record in the datasource is bound to a row in gridview control. Please note that in RowDataBound event, we are first checking, if the row, that is being bound, is a datarow. This event is also raised for other row types like Header, Footer etc.
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
// Check if the row that is being bound, is a datarow
if (e.Row.RowType == DataControlRowType.DataRow)
{
if (e.Row.Cells[3].Text == "US")
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string formattedString = string.Format(new System.Globalization.CultureInfo("en-US"), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
else if (e.Row.Cells[3].Text == "UK")
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string formattedString = string.Format(new System.Globalization.CultureInfo("en-GB"), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
else if (e.Row.Cells[3].Text == "India")
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string formattedString = string.Format(new System.Globalization.CultureInfo("en-IN"), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
else if (e.Row.Cells[3].Text == "South Africa")
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string formattedString = string.Format(new System.Globalization.CultureInfo("en-ZA"), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
else if (e.Row.Cells[3].Text == "Malaysia")
{
int salary = Convert.ToInt32(e.Row.Cells[2].Text);
string formattedString = string.Format(new System.Globalization.CultureInfo("en-MY"), "{0:c}", salary);
e.Row.Cells[2].Text = formattedString;
}
}
}
Use the code below, to get the list of all supported cultures in asp.net
foreach (CultureInfo ci in CultureInfo.GetCultures(CultureTypes.AllCultures))
{
Response.Write(ci.Name + " => " + ci.DisplayName + "<br/>");
}
The list of countries and their currencies can be found at the following wikipedia article
http://en.wikipedia.org/wiki/List_of_circulating_currencies
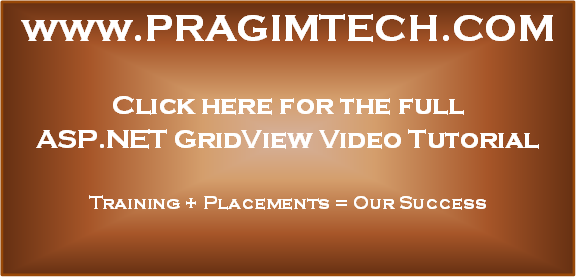
How you are able to display new rupee symbol here ?
ReplyDeleteIE has some problem displaying Indian rupee symbol, but it works fine with Chrome browser
ReplyDelete