Suggested Videos
Part 13 - Conditional statements in javascript
Part 14 - Switch statement in JavaScript
Part 15 - Ternary operator in JavaScript
Here are the basic loops in JavaScript
while
do...while
for
In this video we will discuss while loop in JavaScript with an example. The following example, prints all even numbers from 0 till the target number that the end user has provided.
How does the while loop work
1. While loop checks the condition first
2. If the condition is true, statements with in the loop are executed
3. This process is repeated as long as the condition evaluates to true.
Failure to update the variable that participates in the condition will create a never ending or infinite loop. In the example below, notice that we have commented the line that updates start variable. As a result of this start will always be ZERO and will always be less than the target number. This mean the condition will never become false, and we have an infinite while loop.
JavaScript infinite while loop example
Let us now understand the use of break statement in a while loop with an example. We want a while loop that would to the following
1. If the target number that is provided by the end user is less than 100, then print all even numbers from ZERO until the target number
2. If the target number that is provided by the end user is greater than 100, then print all even numbers from ZERO until 100.
So, this means the loop should break once it has printed even numbers until 100.
JavaScript while loop break out example
What is the use of break statement
If break statement is used in a switch statement, it causes the execution to break out of that switch statement. In a similar fashion, if used in a loop, the loop ends.
JavasSript while loop continue example
continue statement tells the JavaScript interpreter to skip remaining code that is present after this statement and start the next iteration of the loop. Let us understand this with an example.
Example: The following example prints all odd numbers between 1 and 10.
Part 13 - Conditional statements in javascript
Part 14 - Switch statement in JavaScript
Part 15 - Ternary operator in JavaScript
Here are the basic loops in JavaScript
while
do...while
for
In this video we will discuss while loop in JavaScript with an example. The following example, prints all even numbers from 0 till the target number that the end user has provided.
var targetNumber = Number(prompt("Please enter your target number", ""));
var start = 0;
while (start <= targetNumber)
{
document.write(start + "<br/>");
start = start + 2;
}
How does the while loop work
1. While loop checks the condition first
2. If the condition is true, statements with in the loop are executed
3. This process is repeated as long as the condition evaluates to true.
Failure to update the variable that participates in the condition will create a never ending or infinite loop. In the example below, notice that we have commented the line that updates start variable. As a result of this start will always be ZERO and will always be less than the target number. This mean the condition will never become false, and we have an infinite while loop.
JavaScript infinite while loop example
var targetNumber = Number(prompt("Please enter your target number", ""));
var start = 0;
while (start <= targetNumber)
{
document.write(start + "<br/>");
// start = start + 2;
}
Let us now understand the use of break statement in a while loop with an example. We want a while loop that would to the following
1. If the target number that is provided by the end user is less than 100, then print all even numbers from ZERO until the target number
2. If the target number that is provided by the end user is greater than 100, then print all even numbers from ZERO until 100.
So, this means the loop should break once it has printed even numbers until 100.
JavaScript while loop break out example
var targetNumber = Number(prompt("Please enter your target number", ""));
var start = 0;
while (start <= targetNumber)
{
document.write(start + "<br/>");
start = start + 2;
if (start > 100) {
break;
}
}
What is the use of break statement
If break statement is used in a switch statement, it causes the execution to break out of that switch statement. In a similar fashion, if used in a loop, the loop ends.
JavasSript while loop continue example
continue statement tells the JavaScript interpreter to skip remaining code that is present after this statement and start the next iteration of the loop. Let us understand this with an example.
Example: The following example prints all odd numbers between 1 and 10.
var start = 0;
while (start < 10)
{
start = start + 1;
if (start % 2 == 0)
{
continue;
}
document.write(start + "<br/>");
}
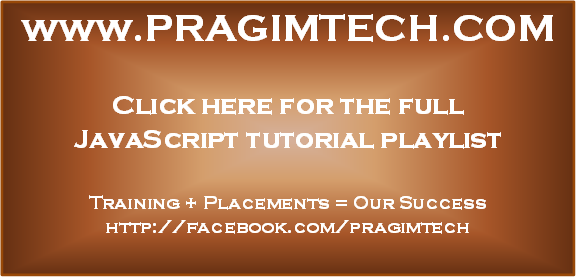
No comments:
Post a Comment
It would be great if you can help share these free resources