Suggested Videos
Part 11 - Substrings in JavaScript
Part 12 - JavaScript substring example
Part 13 - Conditional statements in javascript
When should we use switch statement
To improve the readability of a program multiple if else if statements can be replaced with a switch statement.
Notice that in the following code we have several if else if statements
The above code can be rewritten using a switch statement and it greatly improves the readability
In general we need to have break statement after each case statement to ensure the program breaks out of the switch statement after executing statements present in a specific case.
What happens if there is no break in a switch statement
If there is no break statement the execution falls automatically to next case until a break statement is encountered or end of the program is reached.
In the example below, since we don't have a break statement for case 1, when we enter 1 as the number we would get 2 alerts. First alert from case 1 and the second alert from case 2.
When would you combine multiple case statements together
If you want the same piece of code to be executed for multiple cases you can combine them together as shown below. Case statement with no code in-between creates a single case for multiple values. A case without any code will automatically fall through to the next case.
In this example case 1 and case 2 will fall through and execute code for case 3
Part 11 - Substrings in JavaScript
Part 12 - JavaScript substring example
Part 13 - Conditional statements in javascript
When should we use switch statement
To improve the readability of a program multiple if else if statements can be replaced with a switch statement.
Notice that in the following code we have several if else if statements
var userInput = Number(prompt("Please enter a number", ""));
if (userInput == 1)
{
alert("You number
is One");
}
else if (userInput == 2)
{
alert("You number
is Two");
}
else if (userInput == 3)
{
alert("Your number
is Three");
}
else
{
alert("Your number
is not between 1 and 3");
}
The above code can be rewritten using a switch statement and it greatly improves the readability
var userInput = Number(prompt("Please enter a number", ""));
switch (userInput)
{
case 1:
alert("You number
is One");
break;
case 2:
alert("You number
is Two");
break;
case 3:
alert("You number
is Three");
break;
default:
alert("You number
is not between 1 and 3");
break;
}
In general we need to have break statement after each case statement to ensure the program breaks out of the switch statement after executing statements present in a specific case.
What happens if there is no break in a switch statement
If there is no break statement the execution falls automatically to next case until a break statement is encountered or end of the program is reached.
In the example below, since we don't have a break statement for case 1, when we enter 1 as the number we would get 2 alerts. First alert from case 1 and the second alert from case 2.
var userInput = Number(prompt("Please enter a number", ""));
switch (userInput)
{
case 1:
alert("You number
is One");
case 2:
alert("You number
is Two");
break;
case 3:
alert("You number
is Three");
break;
default:
alert("You number
is not between 1 and 3");
break;
}
When would you combine multiple case statements together
If you want the same piece of code to be executed for multiple cases you can combine them together as shown below. Case statement with no code in-between creates a single case for multiple values. A case without any code will automatically fall through to the next case.
In this example case 1 and case 2 will fall through and execute code for case 3
var userInput = Number(prompt("Please enter a number", ""));
switch (userInput)
{
case 1:
case 2:
case 3:
alert("You number
is " + userInput);
break;
default:
alert("You number
is not between 1 and 3");
break;
}
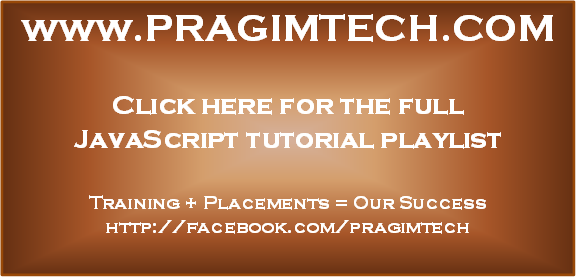
No comments:
Post a Comment
It would be great if you can help share these free resources