Suggested Videos
Part 10 - Strings in JavaScript
Part 11 - Substrings in JavaScript
Part 12 - JavaScript substring example
JavaScript code is executed in a linear fashion from the first line to the last line. If for some reason you want to interrupt this flow and execute certain statements, only, if certain condition is met, then we use conditional statements.
JavaScript has the following conditional statements
if
if else
if else if else
switch
ternary operator - shortcut for an if...else statement
If example
var userInput = Number(prompt("Please enter a number", ""));
If else if example
Part 10 - Strings in JavaScript
Part 11 - Substrings in JavaScript
Part 12 - JavaScript substring example
JavaScript code is executed in a linear fashion from the first line to the last line. If for some reason you want to interrupt this flow and execute certain statements, only, if certain condition is met, then we use conditional statements.
JavaScript has the following conditional statements
if
if else
if else if else
switch
ternary operator - shortcut for an if...else statement
If example
var userInput = Number(prompt("Please enter a number", ""));
if (userInput == 1)
{
alert("You number
is One");
}
if (userInput == 2)
{
alert("You number
is Two");
}
if (userInput == 3)
{
alert("Your number
is Three");
}
if (userInput != 1 && userInput
!= 2 && userInput != 3)
{
alert("Your number
is not between 1 and 3");
}
var userInput = Number(prompt("Please enter a number", ""));
if (userInput == 1)
{
alert("You number
is One");
}
else if (userInput == 2)
{
alert("You number
is Two");
}
else if (userInput == 3)
{
alert("Your number
is Three");
}
else
{
alert("Your number
is not between 1 and 3");
}
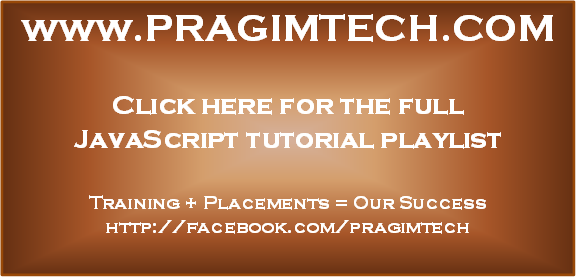
No comments:
Post a Comment
It would be great if you can help share these free resources