Suggested Videos
Part 12 - JavaScript substring example
Part 13 - Conditional statements in javascript
Part 14 - Switch statement in JavaScript
Ternary operator can be used as a shortcut for if...else... statement.
Here is the syntax for the ternary operator
Boolean Expression ? Statements to execute if true : Statements to execute if false
The following example checks if the number is even or odd. We are using if...else... statement.
Ternary operator example : In the above example if...else... statement can be replaced with the ternary operator as shown below.
Can we have multiple statements per case with a ternary operator
Yes, we can put the multiple statements in a bracket and separate them with a comma.
Example :
Multiple if..else if... statements can be replaced with ternary operator.
The following example displays the month name based on the month number that is provided using if...else if... statement
Ternary operator with multiple conditions example : In the following example, if...else if... statements are replaced with ternary operator
Part 12 - JavaScript substring example
Part 13 - Conditional statements in javascript
Part 14 - Switch statement in JavaScript
Ternary operator can be used as a shortcut for if...else... statement.
Here is the syntax for the ternary operator
Boolean Expression ? Statements to execute if true : Statements to execute if false
The following example checks if the number is even or odd. We are using if...else... statement.
var userInput = Number(prompt("Please enter a number"));
var message = "";
if (userInput % 2 == 0)
{
message = "Your
number is even";
}
else
{
message = "Your
number is odd";
}
alert(message);
Ternary operator example : In the above example if...else... statement can be replaced with the ternary operator as shown below.
var userInput = Number(prompt("Please enter a number"));
var message = userInput % 2 ==
0 ? "Your number is
even"
: "Your number is
odd";
alert(message);
Can we have multiple statements per case with a ternary operator
Yes, we can put the multiple statements in a bracket and separate them with a comma.
Example :
var userInput = Number(prompt("Please enter a number"));
userInput % 2 == 0
?
(alert("Your number is even"),
alert("Your number is "
+ userInput))
: (alert("Your number is odd"), alert("Your number is " + userInput));
Multiple if..else if... statements can be replaced with ternary operator.
The following example displays the month name based on the month number that is provided using if...else if... statement
var userInput = Number(prompt("Please enter a month number - 1, 2 or 3"));
var monthName = "";
if (userInput == 1)
{
monthName = "January";
}
else if (userInput == 2)
{
monthName = "February";
}
else if (userInput == 3)
{
monthName = "March";
}
else
{
monthName = "Unknown
month number"
}
alert(monthName);
Ternary operator with multiple conditions example : In the following example, if...else if... statements are replaced with ternary operator
var userInput = Number(prompt("Please enter a month number - 1, 2 or 3"));
var monthName = userInput == 1
? "January" : userInput
== 2
? "February"
: userInput == 3
? "March"
: "Unknown month number";
alert(monthName);
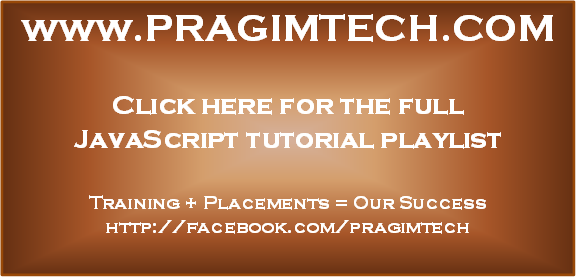
No comments:
Post a Comment
It would be great if you can help share these free resources