Suggested Videos
Part 13 - Handling 404 error in razor pages project | Text | Slides
Part 14 - Editing data in asp.net core razor pages | Text | Slides
Part 15 - Updating data in asp.net core razor pages | Text | Slides
In this video we will discuss how to upload a file to the server using ASP.NET Core razor pages framework.
We discussed how to display existing employee data (Name, Email and Department) on Edit razor page as shown in the image below in our previous video. In this video we will discuss how to delete the existing employee photo and upload a new photo.
Edit.cshtml
Edit.cshtml.cs
IEmployeeRepository.cs
MockEmployeeRepository.cs
Part 13 - Handling 404 error in razor pages project | Text | Slides
Part 14 - Editing data in asp.net core razor pages | Text | Slides
Part 15 - Updating data in asp.net core razor pages | Text | Slides
In this video we will discuss how to upload a file to the server using ASP.NET Core razor pages framework.
We discussed how to display existing employee data (Name, Email and Department) on Edit razor page as shown in the image below in our previous video. In this video we will discuss how to delete the existing employee photo and upload a new photo.
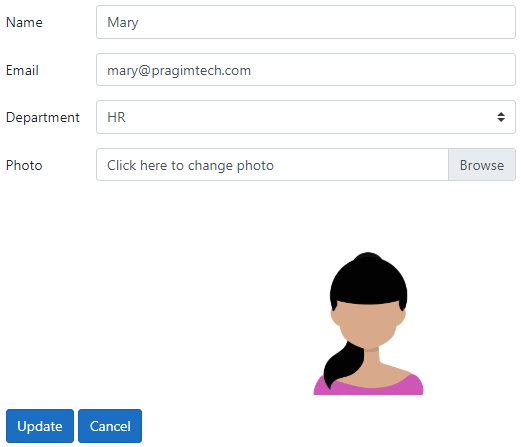
Edit.cshtml
@page "{id:min(1)}"
@model
RazorPagesTutorial.Pages.Employees.EditModel
@{
ViewData["Title"] = "Edit";
// If an
employee does not have a photo display noimage.png
var photoPath = "~/images/" + (Model.Employee.PhotoPath ?? "noimage.jpg");
}
<h1>Edit</h1>
<form method="post" class="mt-3" enctype="multipart/form-data">
<input hidden asp-for="Employee.Id" />
@*Store employee existing Photo
Path in a hidden field*@
<input hidden asp-for="Employee.PhotoPath" />
<div class="form-group row">
<label asp-for="Employee.Name" class="col-sm-2 col-form-label">
</label>
<div class="col-sm-10">
<input asp-for="Employee.Name" class="form-control" placeholder="Name">
</div>
</div>
<div class="form-group row">
<label asp-for="Employee.Email" class="col-sm-2 col-form-label"></label>
<div class="col-sm-10">
<input asp-for="Employee.Email" class="form-control" placeholder="Email">
</div>
</div>
<div class="form-group row">
<label asp-for="Employee.Department" class="col-sm-2 col-form-label"></label>
<div class="col-sm-10">
<select asp-for="Employee.Department" class="custom-select mr-sm-2"
asp-items="Html.GetEnumSelectList<Dept>()">
<option value="">Please Select</option>
</select>
</div>
</div>
<div class="form-group row">
<label asp-for="Photo" class="col-sm-2
col-form-label"></label>
<div class="col-sm-10">
<div class="custom-file">
@*Photo property type is
IFormFile, so ASP.NET Core
automatically creates a
FileUpload control *@
<input asp-for="Photo" class="custom-file-input
form-control">
<label class="custom-file-label">Click
here to change photo</label>
</div>
</div>
</div>
@*Display the existing employee
photo*@
<div class="form-group row col-sm-4 offset-4">
<img class="imageThumbnail" src="@photoPath" asp-append-version="true" />
</div>
<div class="form-group row">
<div class="col-sm-10">
<button type="submit" class="btn btn-primary">Update</button>
<a asp-page="/Employees/Index" class="btn btn-primary">Cancel</a>
</div>
</div>
@*This jquery code is required to
display the selected
file name in the file upload controls*@
@section Scripts {
<script>
$(document).ready(function () {
$('.custom-file-input').on("change", function () {
var fileName = $(this).val().split("\\").pop();
$(this).next('.custom-file-label').html(fileName);
});
});
</script>
}
</form>
Edit.cshtml.cs
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using RazorPagesTutorial.Models;
using RazorPagesTutorial.Services;
using System;
using System.IO;
namespace RazorPagesTutorial.Pages.Employees
{
public class EditModel : PageModel
{
private readonly IEmployeeRepository employeeRepository;
private readonly IWebHostEnvironment webHostEnvironment;
public EditModel(IEmployeeRepository employeeRepository,
IWebHostEnvironment
webHostEnvironment)
{
this.employeeRepository = employeeRepository;
// IWebHostEnvironment
service allows us to get the
// absolute
path of WWWRoot folder
this.webHostEnvironment = webHostEnvironment;
}
public Employee Employee { get; set; }
// We use this
property to store and process
// the newly
uploaded photo
[BindProperty]
public IFormFile Photo { get; set; }
public IActionResult OnGet(int id)
{
Employee =
employeeRepository.GetEmployee(id);
if (Employee == null)
{
return RedirectToPage("/NotFound");
}
return Page();
}
public IActionResult OnPost(Employee employee)
{
if (Photo != null)
{
// If a
new photo is uploaded, the existing photo must be
//
deleted. So check if there is an existing photo and delete
if (employee.PhotoPath != null)
{
string filePath =
Path.Combine(webHostEnvironment.WebRootPath,
"images",
employee.PhotoPath);
System.IO.File.Delete(filePath);
}
// Save
the new photo in wwwroot/images folder and update
//
PhotoPath property of the employee object
employee.PhotoPath =
ProcessUploadedFile();
}
Employee =
employeeRepository.Update(employee);
return RedirectToPage("Index");
}
private string ProcessUploadedFile()
{
string uniqueFileName = null;
if (Photo != null)
{
string uploadsFolder = Path.Combine(webHostEnvironment.WebRootPath, "images");
uniqueFileName =
Guid.NewGuid().ToString() + "_" + Photo.FileName;
string filePath = Path.Combine(uploadsFolder, uniqueFileName);
using (var
fileStream = new
FileStream(filePath, FileMode.Create))
{
Photo.CopyTo(fileStream);
}
}
return uniqueFileName;
}
}
}
IEmployeeRepository.cs
using RazorPagesTutorial.Models;
using System.Collections.Generic;
namespace RazorPagesTutorial.Services
{
public interface IEmployeeRepository
{
IEnumerable<Employee>
GetAllEmployees();
Employee GetEmployee(int id);
Employee Update(Employee
updatedEmployee);
}
}
MockEmployeeRepository.cs
using RazorPagesTutorial.Models;
using System.Collections.Generic;
using System.Linq;
namespace RazorPagesTutorial.Services
{
public class MockEmployeeRepository :
IEmployeeRepository
{
private List<Employee> _employeeList;
public MockEmployeeRepository()
{
_employeeList = new List<Employee>()
{
new Employee() { Id = 1, Name = "Mary", Department = Dept.HR,
Email = "mary@pragimtech.com",
PhotoPath="mary.png" },
new Employee() { Id = 2, Name = "John", Department = Dept.IT,
Email = "john@pragimtech.com",
PhotoPath="john.png" },
new Employee() { Id = 3, Name = "Sara", Department = Dept.IT,
Email = "sara@pragimtech.com",
PhotoPath="sara.png" },
new Employee() { Id = 4, Name = "David", Department = Dept.Payroll,
Email = "david@pragimtech.com" },
};
}
public IEnumerable<Employee> GetAllEmployees()
{
return _employeeList;
}
public Employee GetEmployee(int id)
{
return _employeeList.FirstOrDefault(e => e.Id == id);
}
public Employee Update(Employee updatedEmployee)
{
Employee employee =
_employeeList.FirstOrDefault(e
=> e.Id == updatedEmployee.Id);
if (employee != null)
{
employee.Name =
updatedEmployee.Name;
employee.Email =
updatedEmployee.Email;
employee.Department = updatedEmployee.Department;
employee.PhotoPath =
updatedEmployee.PhotoPath;
}
return employee;
}
}
}

No comments:
Post a Comment
It would be great if you can help share these free resources