Suggested Videos
Part 11 - Route constraints in asp.net core | Text | Slides
Part 12 - ASP.NET core custom route constraint | Text | Slides
Part 13 - Handling 404 error in razor pages project | Text | Slides
In this video we will discuss implementing Edit razor page.
Navigate to Edit razor page
When the Edit button on the employees list razor page (/Employees/Index.cshtml) is clicked, we want to redirect the request to Edit razor page (/Employees/Edit.cshtml)
Edit razor page
Add a new razor page to the Employees folder. Name it Edit.cshtml. From design standpoint, Edit razor page should look as shown below. Use a textbox to edit emplyee NAME and EMAIL and a select list for DEPARTMENT.
Edit.cshtml.cs
Edit.cshtml
If you are new to tag helpers we discussed them in detail from Parts 35 to 40 in our ASP.NET Core tutorial for beginners course.
Changes in _ViewImports.cshtml
Include the following using declaration.
Part 11 - Route constraints in asp.net core | Text | Slides
Part 12 - ASP.NET core custom route constraint | Text | Slides
Part 13 - Handling 404 error in razor pages project | Text | Slides
In this video we will discuss implementing Edit razor page.
Navigate to Edit razor page
When the Edit button on the employees list razor page (/Employees/Index.cshtml) is clicked, we want to redirect the request to Edit razor page (/Employees/Edit.cshtml)

<a asp-page="/Employees/Edit" asp-route-ID="@employee.Id"
class="btn btn-primary m-1">Edit</a>
Edit razor page
Add a new razor page to the Employees folder. Name it Edit.cshtml. From design standpoint, Edit razor page should look as shown below. Use a textbox to edit emplyee NAME and EMAIL and a select list for DEPARTMENT.
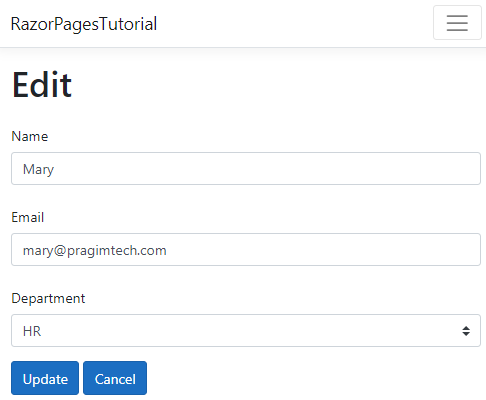
Edit.cshtml.cs
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using RazorPagesTutorial.Models;
using RazorPagesTutorial.Services;
namespace RazorPagesTutorial.Pages.Employees
{
public class EditModel : PageModel
{
private readonly IEmployeeRepository employeeRepository;
public EditModel(IEmployeeRepository employeeRepository)
{
this.employeeRepository = employeeRepository;
}
// This is the
property the display template will use to
// display
existing Employee data
public Employee Employee { get; private set; }
// Populate
Employee property
public IActionResult OnGet(int id)
{
Employee =
employeeRepository.GetEmployee(id);
if (Employee == null)
{
return RedirectToPage("/NotFound");
}
return Page();
}
}
}
Edit.cshtml
If you are new to tag helpers we discussed them in detail from Parts 35 to 40 in our ASP.NET Core tutorial for beginners course.
@page "{id:min(1)}"
@model
RazorPagesTutorial.Pages.Employees.EditModel
@{
ViewData["Title"] = "Edit";
}
<h1>Edit</h1>
<form method="post" class="mt-3">
@*Use hidden input elements to
store employee id which
we need when we submit the form*@
<input hidden asp-for="Employee.Id" />
@*asp-for tag helper takes care of
displaying the existing
data in the respective input elements*@
<div class="form-group row">
<label asp-for="Employee.Name" class="col-sm-2 col-form-label">
</label>
<div class="col-sm-10">
<input asp-for="Employee.Name" class="form-control" placeholder="Name">
</div>
</div>
<div class="form-group row">
<label asp-for="Employee.Email" class="col-sm-2 col-form-label"></label>
<div class="col-sm-10">
<input asp-for="Employee.Email" class="form-control" placeholder="Email">
</div>
</div>
<div class="form-group row">
<label asp-for="Employee.Department" class="col-sm-2 col-form-label"></label>
<div class="col-sm-10">
<select asp-for="Employee.Department" class="custom-select mr-sm-2"
asp-items="Html.GetEnumSelectList<Dept>()">
<option value="">Please Select</option>
</select>
</div>
</div>
<div class="form-group row">
<div class="col-sm-10">
<button type="submit" class="btn btn-primary">Update</button>
<a asp-page="/Employees/Index" class="btn btn-primary">Cancel</a>
</div>
</div>
</form>
Changes in _ViewImports.cshtml
Include the following using declaration.
@using RazorPagesTutorial.Models

No comments:
Post a Comment
It would be great if you can help share these free resources