Suggested Videos
Part 64 - ASP.NET Core LogLevel configuration | Text | Slides
Part 65 - ASP.NET Core Identity tutorial from scratch | Text | Slides
Part 66 - Register new user using asp.net core identity | Text | Slides
In this video we will discuss, how to
This is continuation to Part 66. Please watch Part 66 from ASP.NET Core tutorial before proceeding.
UserManager<IdentityUser> class contains the required methods to manage users in the underlying data store. For example, this class has methods like CreateAsync, DeleteAsync, UpdateAsync to create, delete and update users.
SignInManager<IdentityUser> class contains the required methods for users signin. For example, this class has methods like SignInAsync, SignOutAsync to signin and signout a user.
At this point if you run the project and provide a valid email address and password, the user account should be created in AspNetUsers table in the underlying SQL server database. You could view this data from SQL Server Object Explorer in Visual Studio
Part 64 - ASP.NET Core LogLevel configuration | Text | Slides
Part 65 - ASP.NET Core Identity tutorial from scratch | Text | Slides
Part 66 - Register new user using asp.net core identity | Text | Slides
In this video we will discuss, how to
- Create a new user, using UserManager service provided by asp.net core identity.
- Sign-in a user using SignInManager service provided by asp.net core identity.
This is continuation to Part 66. Please watch Part 66 from ASP.NET Core tutorial before proceeding.

UserManager<IdentityUser> class contains the required methods to manage users in the underlying data store. For example, this class has methods like CreateAsync, DeleteAsync, UpdateAsync to create, delete and update users.
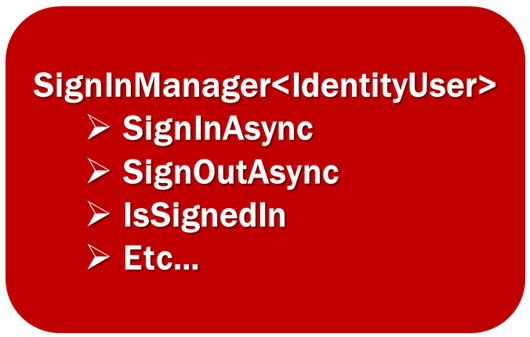
SignInManager<IdentityUser> class contains the required methods for users signin. For example, this class has methods like SignInAsync, SignOutAsync to signin and signout a user.
- Both UserManager and SignInManager services are injected into the AccountController using constructor injection
- Both these services accept a generic parameter. We use the generic parameter to specify the User class that these services should work with.
- At the moment, we are using the built-in IdentityUser class as the argument for the generic parameter.
- The generic parameter on these 2 services is an extension point.
- This means, we can create our own custom user with any additional data that we want to capture about the user and then plug-in this custom class as an argument for the generic parameter instead of the built-in IdentityUser class.
- We will discuss how to do this in our upcoming videos.
using EmployeeManagement.ViewModels;
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
namespace EmployeeManagement.Controllers
{
public class AccountController : Controller
{
private readonly UserManager<IdentityUser> userManager;
private readonly SignInManager<IdentityUser> signInManager;
public AccountController(UserManager<IdentityUser> userManager,
SignInManager<IdentityUser>
signInManager)
{
this.userManager = userManager;
this.signInManager = signInManager;
}
[HttpGet]
public IActionResult Register()
{
return View();
}
[HttpPost]
public async
Task<IActionResult> Register(RegisterViewModel model)
{
if (ModelState.IsValid)
{
// Copy
data from RegisterViewModel to IdentityUser
var user = new
IdentityUser
{
UserName = model.Email,
Email = model.Email
};
// Store
user data in AspNetUsers database table
var result = await
userManager.CreateAsync(user, model.Password);
// If user is
successfully created, sign-in the user using
//
SignInManager and redirect to index action of HomeController
if (result.Succeeded)
{
await signInManager.SignInAsync(user,
isPersistent: false);
return RedirectToAction("index", "home");
}
// If
there are any errors, add them to the ModelState object
// which
will be displayed by the validation summary tag helper
foreach (var error in result.Errors)
{
ModelState.AddModelError(string.Empty, error.Description);
}
}
return View(model);
}
}
}
At this point if you run the project and provide a valid email address and password, the user account should be created in AspNetUsers table in the underlying SQL server database. You could view this data from SQL Server Object Explorer in Visual Studio

Thank you for your input. I have a question turns out that I am using identity for user identification. I register my users and log in the first time but when I want to make a new login I get the message Invalid login Attempt. Then finding out I found that it is not allowed as a username because the @ is included, but I want to keep my username as email. Another solution I found but it does not seem the most correct is changing in the database the EmailConfirmed column to true to true. on the other hand probe put in my starup file the following code options.SignIn.RequireConfirmedEmail = true; But neither The problem is that the PasswordSignInAsync method shows as a result Not Allowed can you help me with any solution?
ReplyDeleteHow to configure the identity in config services for dot net core 3.0
ReplyDeleteHi sir! thx u for such a great tutorial, could u tell me where can i get a source code?
ReplyDeleteAs far as I know you need to create it by yourself.. :)
ReplyDelete