Suggested Videos
Part 33 - Creating feature routing module in angular | Text | Slides
Part 34 - Creating shared module in angular | Text | Slides
Part 35 - Grouping routes and creating component less route in angular | Text | Slides
In this video we will discuss lazy loading angular modules.
At the moment the sample application that we have been working with has 2 modules.
As you add more features to your application you will have more feature modules like ReportsModule, AdminModule etc. As you add more feature modules, the overall application size will continue to grow. At some point you'll reach a tipping point where the application takes a very long time to load.
Unless, you are using lazy loading, all the modules are eagerly loaded. This means, all the modules in your angular application and their associated components, directives, pipes and services must be downloaded from the server, when the user first visits the application. Depending on the number of modules in your application and the internet speed, this could take a significant amount of time and may very badly affect the end user experience.
To address this problem, we use asynchronous routing, which loads feature modules lazily, on demand. This can significantly reduce the initial load time of your application.
At the moment, the 2 modules (AppModule & EmployeeModule) in our application are eagerly loaded.
We want to lazily load EmployeeModule. To lazy load a module, it has to meet 2 requirements.
At the moment, our application does not meet the second requirement. EmployeeModule that we want to lazy load is referenced in the AppModule. So please delete the EmployeeModule references in the AppModule (app.module.ts)
At this point, if we navigate to any of the EmployeeModule routes (i.e /employees or employees/create or employees/edit/2), we see the PageNotFoundComponent template displayed.
To fix this, we have to lazy load the EmployeeModule. To achieve this, include the following route in app-routing.module.ts file. This new route, lazily loads the EmployeeModule. Make sure the below route is before the wild card route in the AppRoutingModule. Otherwise we would not be able to get to any of the EmployeeModule routes.
At this point, if we navigate to any of the EmployeeModule routes (i.e /employees or employees/create or employees/edit/2), we see either an empty page or the PageNotFoundComponent template displayed.
But if we include an extra /employees in the path as shown below, then all the EmployeeModule routes work as expected
To fix this, in employee-routing.module.ts file, change the following routes. We do not need the parent route - 'employees'
After you remove the 'employees' parent route, the routes should look as shown below.
With the above changes, the Employee feature module should be lazily loaded. You can confirm this by looking at the Network tab on the Browser Developer Tools.
Part 33 - Creating feature routing module in angular | Text | Slides
Part 34 - Creating shared module in angular | Text | Slides
Part 35 - Grouping routes and creating component less route in angular | Text | Slides
In this video we will discuss lazy loading angular modules.
At the moment the sample application that we have been working with has 2 modules.
- Root application module - AppModule
- Feature module - EmployeeModule
As you add more features to your application you will have more feature modules like ReportsModule, AdminModule etc. As you add more feature modules, the overall application size will continue to grow. At some point you'll reach a tipping point where the application takes a very long time to load.
Unless, you are using lazy loading, all the modules are eagerly loaded. This means, all the modules in your angular application and their associated components, directives, pipes and services must be downloaded from the server, when the user first visits the application. Depending on the number of modules in your application and the internet speed, this could take a significant amount of time and may very badly affect the end user experience.
To address this problem, we use asynchronous routing, which loads feature modules lazily, on demand. This can significantly reduce the initial load time of your application.
At the moment, the 2 modules (AppModule & EmployeeModule) in our application are eagerly loaded.
We want to lazily load EmployeeModule. To lazy load a module, it has to meet 2 requirements.
- All the routes in the angular module that you want to lazy load should have the same route prefix
- The module should not be referenced in any other module. If it is referenced, the module loader will eagerly load it instead of lazily loading it.
At the moment, our application does not meet the second requirement. EmployeeModule that we want to lazy load is referenced in the AppModule. So please delete the EmployeeModule references in the AppModule (app.module.ts)
At this point, if we navigate to any of the EmployeeModule routes (i.e /employees or employees/create or employees/edit/2), we see the PageNotFoundComponent template displayed.
To fix this, we have to lazy load the EmployeeModule. To achieve this, include the following route in app-routing.module.ts file. This new route, lazily loads the EmployeeModule. Make sure the below route is before the wild card route in the AppRoutingModule. Otherwise we would not be able to get to any of the EmployeeModule routes.
{ path: 'employees', loadChildren: './employee/employee.module#EmployeeModule' }
At this point, if we navigate to any of the EmployeeModule routes (i.e /employees or employees/create or employees/edit/2), we see either an empty page or the PageNotFoundComponent template displayed.
But if we include an extra /employees in the path as shown below, then all the EmployeeModule routes work as expected
/employees/employees |
/employees/employees/create |
/employees/employees/edit/2 |
To fix this, in employee-routing.module.ts file, change the following routes. We do not need the parent route - 'employees'
const appRoutes: Routes = [
{
path: 'employees',
children: [
{ path: '', component: ListEmployeesComponent },
{ path: 'create', component: CreateEmployeeComponent },
{ path: 'edit/:id', component: CreateEmployeeComponent },
]
}
];
{
path: 'employees',
children: [
{ path: '', component: ListEmployeesComponent },
{ path: 'create', component: CreateEmployeeComponent },
{ path: 'edit/:id', component: CreateEmployeeComponent },
]
}
];
After you remove the 'employees' parent route, the routes should look as shown below.
const appRoutes: Routes = [
{ path: '', component: ListEmployeesComponent },
{ path: 'create', component: CreateEmployeeComponent },
{ path: 'edit/:id', component: CreateEmployeeComponent },
];
{ path: '', component: ListEmployeesComponent },
{ path: 'create', component: CreateEmployeeComponent },
{ path: 'edit/:id', component: CreateEmployeeComponent },
];
With the above changes, the Employee feature module should be lazily loaded. You can confirm this by looking at the Network tab on the Browser Developer Tools.
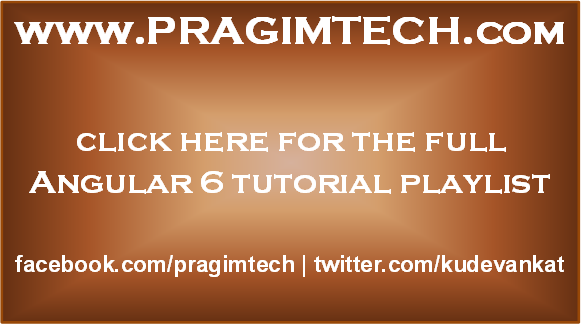
Very nice explaination and very help full all tutorial s , thanks sir.
ReplyDeleteloadChildren has new syntax now
ReplyDelete{path: 'employees', loadChildren: () => import('./employee/employee.module').then(m => m.EmployeeModule) }
loadChildren has new syntax now.
ReplyDelete{path: 'employees', loadChildren: () => import('./employee/employee.module').then(m => m.EmployeeModule) }