Suggested Videos
Part 5 - Angular form control and form group | Text | Slides
Part 6 - Angular nested form groups | Text | Slides
Part 7 - Angular setvalue and patchvalue methods | Text | Slides
In this video we will discuss an easier way of creating reactive forms using the FormBuilder class
In Angular, there are 2 ways to create reactive forms
The FormBuilder class provides syntactic sugar that shortens creating instances of a FormControl, FormGroup, or FormArray. It reduces the amount of code we have to write to build complex reactive forms. The FormBuilder service has three methods:
Step 1 : Import FormBuilder
The FormBuilder class is provided as a service, so first let's import the service
Step 2 : Inject the FormBuilder service
Once the FormBuilder service is imported, inject it into the component using the constructor
Step 3 : Use the FormBuilder
FormBuilder reduces the amount of boilerplate code we have to write to build complex reactive forms.
Part 5 - Angular form control and form group | Text | Slides
Part 6 - Angular nested form groups | Text | Slides
Part 7 - Angular setvalue and patchvalue methods | Text | Slides
In this video we will discuss an easier way of creating reactive forms using the FormBuilder class
In Angular, there are 2 ways to create reactive forms
- Explicitly creating instances of FormGroup and FormControl classes using the new keyword. We discussed this in Part 4 and Part 6 of Angular 6 tutorial.
- Using the FormBuilder class
The FormBuilder class provides syntactic sugar that shortens creating instances of a FormControl, FormGroup, or FormArray. It reduces the amount of code we have to write to build complex reactive forms. The FormBuilder service has three methods:
- control() - Construct a new FormControl instance
- group() - Construct a new FormGroup instance
- array() - Construct a new FormArray instance
Step 1 : Import FormBuilder
The FormBuilder class is provided as a service, so first let's import the service
import { FormBuilder } from '@angular/forms';
Step 2 : Inject the FormBuilder service
Once the FormBuilder service is imported, inject it into the component using the constructor
constructor(private fb: FormBuilder) { }
Step 3 : Use the FormBuilder
- Notice in the example below, we are using the FormBuilder group() method to create a FormGroup instance.
- To the method we pass an object that contains a collection of child controls.
- For each child control we specify a key and value.
- Key is the name of the form control and the value is an array.
- The first element of the array is used to specify an initial value for the form control.
- The second and third elements of the array are used to specify synchronous and asynchronous validators for the form control. We will discuss these when we discuss form validation in our upcoming videos.
- For now, we have defined just the initial value using the first element of the array.
- We have specified an empty string as the default value for all the controls except proficiency radio buttons.
- For proficiency we have a default value of beginner. So the respective radio button is selected when the form loads.
this.employeeForm = this.fb.group({
fullName: [''],
email: [''],
skills: this.fb.group({
skillName: [''],
experienceInYears: [''],
proficiency: ['beginner']
}),
});
FormBuilder reduces the amount of boilerplate code we have to write to build complex reactive forms.
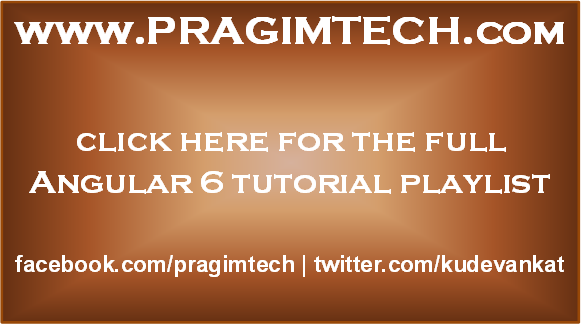
Would it be possible to get a code download for each section as completed? I am having different results and can't find my error.
ReplyDelete