Suggested Videos
Part 2 - Install Bootstrap for Angular 6 | Text | Slides
Part 3 - Angular 6 routing tutorial | Text | Slides
Part 4 - Angular reactive forms tutorial | Text | Slides
In this video we will discuss FormControl and FormGroup classes
The following are some of the useful properties provided by the AbstractControl class
FormGroup instance tracks the value and state of all the form controls in it's group
To see the form model we created using FormGroup and FormControl classes, log the employeeForm to the console.
onSubmit(): void {
console.log(this.employeeForm);
}
On Save button click, you should see the following form model in the browser console.
To access the FormGroup properties use, employeeForm property in the component class. When you press DOT on the employeeForm property you can see all the available properties and methods.
To access a FormControl in a FormGroup, we can use one of the following 2 ways.
Note: This same code works, both in the template and component class.
Please include the following HTML, just after the <form> tag, in the template, and you can see the property values change as you interact with the form controls on the form.
In addition to these properties, AbstractControl also provides the following methods. In our upcoming videos we will use these properties and methods for form validation and working with data.
Part 2 - Install Bootstrap for Angular 6 | Text | Slides
Part 3 - Angular 6 routing tutorial | Text | Slides
Part 4 - Angular reactive forms tutorial | Text | Slides
In this video we will discuss FormControl and FormGroup classes
- When working with reactive forms we create instances of FormControl and FormGroup classes to create a form model.
- To bind an HTML <form> tag in the template to the FromGroup instance in the component class, we use formGroup directive
- To bind an HTML <input> element in the template to the FormControl instance in the component class, we use formControlName directive
- formGroup and formControlName directives are provided by the ReactiveFormsModule
- Both FormControl and FormGroup classes inherit from AbstractControl base class
- The AbstractControl class has properties that help us track both FormControl and FormGroup value and state
The following are some of the useful properties provided by the AbstractControl class
- value
- errors
- valid
- invalid
- dirty
- pristine
- touched
- untouched
FormGroup instance tracks the value and state of all the form controls in it's group
To see the form model we created using FormGroup and FormControl classes, log the employeeForm to the console.
onSubmit(): void {
console.log(this.employeeForm);
}
On Save button click, you should see the following form model in the browser console.

To access the FormGroup properties use, employeeForm property in the component class. When you press DOT on the employeeForm property you can see all the available properties and methods.

To access a FormControl in a FormGroup, we can use one of the following 2 ways.
employeeForm.controls.fullName.value
employeeForm.get('fullName').value
employeeForm.get('fullName').value
Note: This same code works, both in the template and component class.
Please include the following HTML, just after the <form> tag, in the template, and you can see the property values change as you interact with the form controls on the form.
<table border="1">
<tr>
<th style="padding: 10px">FormGroup</th>
<th style="padding: 10px">FormControl (fullName)</th>
</tr>
<tr>
<td style="padding: 10px">
touched : {{ employeeForm.touched }}
<br/> dirty : {{ employeeForm.dirty }}
<br/> valid : {{ employeeForm.valid }}
<br/> Form Values : {{employeeForm.value |
json}}
</td>
<td style="padding: 10px">
touched : {{ employeeForm.get('fullName').touched
}}
<br/> dirty : {{
employeeForm.get('fullName').dirty }}
<br/> valid : {{
employeeForm.get('fullName').valid }}
<br/> FullName Value :
{{employeeForm.get('fullName').value}}
</td>
</tr>
</table>
In addition to these properties, AbstractControl also provides the following methods. In our upcoming videos we will use these properties and methods for form validation and working with data.
- setValidators()
- clearValidators()
- updateValueAndValidity()
- setValue()
- patchValue()
- Reset()
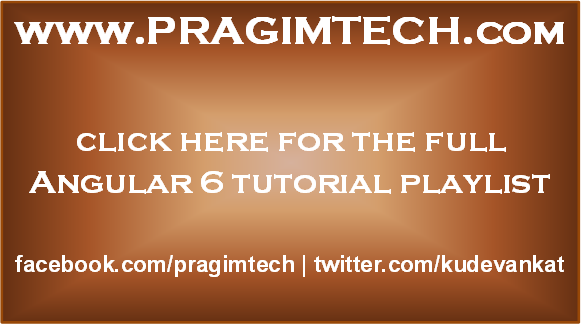
Hi Venkat the above code given the following error employeeForm.get('fullName').touched =>" Object is possibly 'null' "
ReplyDeleteerror showing help me with this
try below code
ReplyDeleteTouched: {{ employeeForm.get('fullName')?.touched }}
Dirty: {{ employeeForm.get('fullName')?.dirty }}
Valid: {{ employeeForm.get('fullName')?.valid }}
Full Name Value: {{ employeeForm.get('fullName')?.value }}