Suggested Videos
Part 16 - Angular reactive form custom validator with parameter | Text | Slides
Part 17 - How to make angular custom validator reusable | Text | Slides
Part 18 - Angular reactive forms cross field validation | Text | Slides
In this video we will discuss FormArray in Angular
To build an Angular Reactive Form we use three fundamental building blocks
We discussed FormControl and FormGroup in our previous videos in this series. In this video we will discuss FormArray in Angular.
A FormArray as the name implies is an array. It can contain an array of
In the example below, we have a FormArray with
To programmatically find the number of elements in a FormArray use the length property
To iterate over a FormArray you can use a for loop. Use instanceof operator to determine if the control that you are currently dealing with is a FormControl, FormGroup or FormArray.
There are 2 ways to create a FormArray in Angular. Using the new keyword or FormBuilder class.
Create a FormArray, using the new keyword
Create a FormArray, using the array() method of the FormBuilder class
Although, we can use a FormArray to store unlike items, we generally use it to store like items. For example, an array of
formArray.value returns
We usually use the following properties to determine the state of a FormControl or a FormGroup. These status properties are also available on a FormArray. For example, if one of the controls in a FormArray is touched, the entire array becomes touched. Similarly, if one of the controls is invalid, the entire array becomes invalid.
We can also use a FormGroup to create a group of FormControls. Notice, in the example below, we are using the group() method of the FormBuilder class to create a FormGroup.
What is the difference between a FormGroup and a FormArray?
Well, in many aspects they are similar. However, one major difference is that a FormArray data is serialized as an array where as a FormGroup is serialized as an object.
To see this, log the FormGroup and FormArray instance to the browser console. In the case of FormArray, controls property contains an array of form controls. Where as, in the case of FormGroup, controls property contains an object with key/value pairs, where key is the name of the form control and value is an instance of FormControl.
The fact that FormArray tracks FormControls as part of an array is very useful, when we want to generate FormControls and FormGroups dynamically. For example, let's say you are filling an employment form, and you want to add multiple skills. We cannot have a fixed number of skill related fields on the form as they are dependant on the employee experience. This is one example where we need to generate form controls dynamically, and a FormArray is a perfect choice for implementing this.
We will discuss generating employee skill related fields dynamically in our next video.
Part 16 - Angular reactive form custom validator with parameter | Text | Slides
Part 17 - How to make angular custom validator reusable | Text | Slides
Part 18 - Angular reactive forms cross field validation | Text | Slides
In this video we will discuss FormArray in Angular
To build an Angular Reactive Form we use three fundamental building blocks
- FormControl
- FormGroup
- FormArray
We discussed FormControl and FormGroup in our previous videos in this series. In this video we will discuss FormArray in Angular.
A FormArray as the name implies is an array. It can contain an array of
- FormControls
- FormGroups
- Nested FormArrays
In the example below, we have a FormArray with
- one FormControl
- one FormGroup
- one FormArray
const formArray = new FormArray([
new FormControl('John', Validators.required),
new FormGroup({
country: new FormControl('', Validators.required)
}),
new FormArray([])
]);
To programmatically find the number of elements in a FormArray use the length property
formArray.length
To iterate over a FormArray you can use a for loop. Use instanceof operator to determine if the control that you are currently dealing with is a FormControl, FormGroup or FormArray.
for (const control of formArray.controls) {
if (control instanceof FormControl) {
console.log('control is FormControl');
}
if (control instanceof FormGroup) {
console.log('control is FormGroup');
}
if (control instanceof FormArray) {
console.log('control is FormArray');
}
}
There are 2 ways to create a FormArray in Angular. Using the new keyword or FormBuilder class.
Create a FormArray, using the new keyword
const formArray = new FormArray([
new FormControl('John', Validators.required),
new FormControl('IT', Validators.required),
]);
Create a FormArray, using the array() method of the FormBuilder class
const formArray = this.fb.array([
new FormControl('John', Validators.required),
new FormControl('IT', Validators.required),
]);
Although, we can use a FormArray to store unlike items, we generally use it to store like items. For example, an array of
- FormControls
- FormGroups
- or Nested FormArrays
const formArray = this.fb.array([
new FormControl('John', Validators.required),
new FormControl('IT', Validators.required),
new FormControl('', Validators.required),
]);
formArray.value returns
["John", "IT", ""]
We usually use the following properties to determine the state of a FormControl or a FormGroup. These status properties are also available on a FormArray. For example, if one of the controls in a FormArray is touched, the entire array becomes touched. Similarly, if one of the controls is invalid, the entire array becomes invalid.
- touched
- untouched
- dirty
- pristine
- valid
- invalid
Method | Purpose |
---|---|
push | Inserts the control at the end of the array |
insert | Inserts the control at the specified index in the array |
removeAt | Removes the control at the specified index in the array |
setControl | Replace an existing control at the specified index in the array |
at | Return the control at the specified index in the array |
We can also use a FormGroup to create a group of FormControls. Notice, in the example below, we are using the group() method of the FormBuilder class to create a FormGroup.
const formGroup = this.fb.group([
new FormControl('John', Validators.required),
new FormControl('IT', Validators.required),
new FormControl('', Validators.required),
]);
What is the difference between a FormGroup and a FormArray?
Well, in many aspects they are similar. However, one major difference is that a FormArray data is serialized as an array where as a FormGroup is serialized as an object.
To see this, log the FormGroup and FormArray instance to the browser console. In the case of FormArray, controls property contains an array of form controls. Where as, in the case of FormGroup, controls property contains an object with key/value pairs, where key is the name of the form control and value is an instance of FormControl.
console.log(formArray.value);
Output: [FormControl, FormControl, FormControl]
Output: [FormControl, FormControl, FormControl]
console.log(formGroup.value);
Output: {0: FormControl, 1: FormControl, 2: FormControl}
Output: {0: FormControl, 1: FormControl, 2: FormControl}
The fact that FormArray tracks FormControls as part of an array is very useful, when we want to generate FormControls and FormGroups dynamically. For example, let's say you are filling an employment form, and you want to add multiple skills. We cannot have a fixed number of skill related fields on the form as they are dependant on the employee experience. This is one example where we need to generate form controls dynamically, and a FormArray is a perfect choice for implementing this.
We will discuss generating employee skill related fields dynamically in our next video.
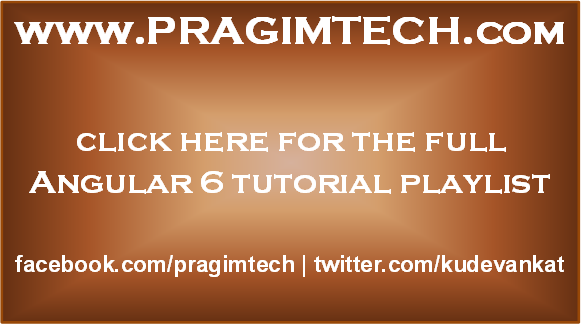
No comments:
Post a Comment
It would be great if you can help share these free resources