Suggested Videos
Part 14 - Dynamically adding or removing form control validators in reactive form | Text | Slides
Part 15 - Angular reactive form custom validator | Text | Slides
Part 16 - Angular reactive form custom validator with parameter | Text | Slides
In this video we will discuss how to make a custom validator reusable in Angular.
The built-in validators in angular like the following, are reusable. This means we can use them with any form control on any angular form.
To be able to use one of the built-in angular validator, all we have to do is import the Validators class from '@angular/forms' package.
Once the Validators class is imported, use the validator functions on the form control that you want to validate.
All the buil-in validator functions are marked as static functions in the Validators class. This allows us to use the validator functions, without the need to create an instance of the Validators class.
Along the same lines let's make our emailDomain custom validator function reusable by including it as a static function in a separate class.
We want to make this validator function available to all form controls on all forms. So, create a shared folder. In the shared folder, create a file with name custom.validators.ts and include the following code.
Using the reusable Custom Validators : First, Import the CustomValidators class. Just like how we import the built-in Validators class, import the CustomValidators class from custom.validators.ts file.
Tie the validator function to a form control that you want to validate.
Part 14 - Dynamically adding or removing form control validators in reactive form | Text | Slides
Part 15 - Angular reactive form custom validator | Text | Slides
Part 16 - Angular reactive form custom validator with parameter | Text | Slides
In this video we will discuss how to make a custom validator reusable in Angular.
The built-in validators in angular like the following, are reusable. This means we can use them with any form control on any angular form.
- required
- min
- max
- minlength
- maxlength
- pattern
To be able to use one of the built-in angular validator, all we have to do is import the Validators class from '@angular/forms' package.
import { Validators } from '@angular/forms';
Once the Validators class is imported, use the validator functions on the form control that you want to validate.
fullName: ['', [Validators.required, Validators.minLength(2)]]
All the buil-in validator functions are marked as static functions in the Validators class. This allows us to use the validator functions, without the need to create an instance of the Validators class.
Along the same lines let's make our emailDomain custom validator function reusable by including it as a static function in a separate class.
We want to make this validator function available to all form controls on all forms. So, create a shared folder. In the shared folder, create a file with name custom.validators.ts and include the following code.
import
{ AbstractControl } from '@angular/forms';
export
class CustomValidators {
static emailDomain(domainName: string) {
return (control: AbstractControl): { [key:
string]: any } | null => {
const
email: string = control.value;
const
domain = email.substring(email.lastIndexOf('@')
+ 1);
if
(email === '' ||
domain.toLowerCase() === domainName.toLowerCase()) {
return
null;
} else
{
return
{ 'emailDomain': true
};
}
};
}
}
Using the reusable Custom Validators : First, Import the CustomValidators class. Just like how we import the built-in Validators class, import the CustomValidators class from custom.validators.ts file.
import { CustomValidators } from '../shared/custom.validators';
Tie the validator function to a form control that you want to validate.
email: ['', [CustomValidators.emailDomain('dell.com')]]
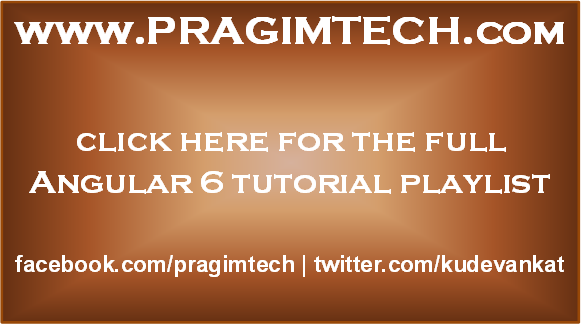
No comments:
Post a Comment
It would be great if you can help share these free resources