Suggested Videos
Part 62 - Angular content projection | Text | Slides
Part 63 - Online fake REST API | Text | Slides
Part 64 - Angular client server architecture | Text | Slides
In this video we will discuss how to call a server side service using Angular HttpClient service. We will specifically discuss, issuing a GET request to retrieve data from the server.
Step 1 : Import Angular HttpClientModule : Before we can use HttpClient service, we need to import Angular HttpClientModule. In most angular applications we do this in the root module AppModule (app.module.ts)
Include the HttpClientModule in the imports array of @NgModule() decorator of AppModule class
Step 2 : Import and inject HttpClient service : We want to use HttpClient service in our EmployeeService (employee.service.ts)
Notice
However, there is an exception to this. If the observable service is being consumed by a Resolver, the resolver service will subscribe to the Observable, we do not have to explicitly subscribe. The resolver will automatically subscribe to the observable service. On the other hand, if the getEmployees() method of the EmployeeService is consumed by a Component or another service, then that component or service must explicitly subscribe to the Observable, otherwise it will not be called.
Make sure the JSON server is running. If it is not running the list route does not display anything. Use the following command to start the JSON server
json-server --watch db.json
At the moment, we are not handling errors. What happens if the request fails on the server, or if a poor network connection prevents the request from even reaching the server. In this case, HttpClient service returns an error object instead of a successful response. We will discuss error handling in our next video.
Part 62 - Angular content projection | Text | Slides
Part 63 - Online fake REST API | Text | Slides
Part 64 - Angular client server architecture | Text | Slides
In this video we will discuss how to call a server side service using Angular HttpClient service. We will specifically discuss, issuing a GET request to retrieve data from the server.
Step 1 : Import Angular HttpClientModule : Before we can use HttpClient service, we need to import Angular HttpClientModule. In most angular applications we do this in the root module AppModule (app.module.ts)
import { HttpClientModule } from '@angular/common/http';
Include the HttpClientModule in the imports array of @NgModule() decorator of AppModule class
Step 2 : Import and inject HttpClient service : We want to use HttpClient service in our EmployeeService (employee.service.ts)
// Import HttpClient service
import
{ HttpClient } from '@angular/common/http';
// Inject the service using the service
class constructor
@Injectable()
export
class EmployeeService {
constructor(private
httpClient: HttpClient) {
}
getEmployees(): Observable<Employee[]> {
return this.httpClient.get<Employee[]>('http://localhost:3000/employees');
}
}
Notice
- We are using the HttpClient service get() method to issue a GET HTTP request.
- In addition to get() method, we also have post(), put(), patch(), and delete() methods to perform the respective HTTP operations.
- To the get() method we pass the URI of the server side service we want to call.
- Also notice we are using the get<T>() method, generic parameter to specify the type of data we are expecting. In our case, we are expecting an Employee[] array back.
- If we were using the old Http service, we would have to use .json() method on the response to get JSON data back.
- With the new HttpClient service, we no longer have to do that. JSON is now the default response.
However, there is an exception to this. If the observable service is being consumed by a Resolver, the resolver service will subscribe to the Observable, we do not have to explicitly subscribe. The resolver will automatically subscribe to the observable service. On the other hand, if the getEmployees() method of the EmployeeService is consumed by a Component or another service, then that component or service must explicitly subscribe to the Observable, otherwise it will not be called.
Make sure the JSON server is running. If it is not running the list route does not display anything. Use the following command to start the JSON server
json-server --watch db.json
At the moment, we are not handling errors. What happens if the request fails on the server, or if a poor network connection prevents the request from even reaching the server. In this case, HttpClient service returns an error object instead of a successful response. We will discuss error handling in our next video.
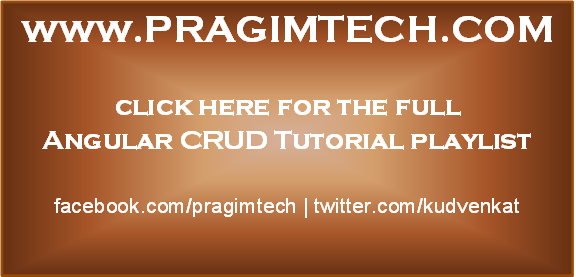
Hi Venkat,
ReplyDeleteGetting below while connect db.json
GET http://localhost:3000/employee net::ERR_CONNECTION_REFUSED