Suggested Videos
Part 31 - Create operation in angular | Text | Slides
Part 32 - Angular switch case example | Text | Slides
Part 33 - Pass data from parent to child component in angular | Text | Slides
In this video we will discuss how to detect and react when component input property value changes. This is continuation to Part 33. Please watch Part 33 from Angular CRUD tutorial before proceeding.
To detect and react when an input property value changes, we have 2 options. We can either use
If you are new to Angular Life Cycle hooks, please check out Part 24 from Angular 2 tutorial.
To understand these 2 approaches, let's modify ListEmployeesComponent as shown below. Notice instead of displaying all the employees at once, we have "View Next Employee" button which cycles through the list of employees, displaying one employee at a time.
To achieve this modify the code in list-employees.component.html file as shown below.
Modify the code in list-employees.component.ts as shown below.
At this point view the page in the browser. Notice only one employee is displayed. Click "View Next Employee" button to see the next employee. It is the child component (DisplayEmployeeComponent) that displays the employee details. For the child component to be able to display the employee details, the parent component is passing the employee object to the child component using the child component input property.
So every time we click the "View Next Employee" button, the INPUT property value changes. When this happens we want to detect the change and react. Let us say for example, we want to log to the console the previously displayed employee name and the currently displayed employee name.
We can achieve this either by using ngOnChanges life cycle hook or Property Setter. Let's look at both the ways.
Detecting and reacting to Input property changes using ngOnChanges life cycle hook
Next Video : Detecting and reacting to Input property changes using Property Setter
Part 31 - Create operation in angular | Text | Slides
Part 32 - Angular switch case example | Text | Slides
Part 33 - Pass data from parent to child component in angular | Text | Slides
In this video we will discuss how to detect and react when component input property value changes. This is continuation to Part 33. Please watch Part 33 from Angular CRUD tutorial before proceeding.
To detect and react when an input property value changes, we have 2 options. We can either use
- Property Setter
- ngOnChanges Life Cycle Hook
If you are new to Angular Life Cycle hooks, please check out Part 24 from Angular 2 tutorial.
To understand these 2 approaches, let's modify ListEmployeesComponent as shown below. Notice instead of displaying all the employees at once, we have "View Next Employee" button which cycles through the list of employees, displaying one employee at a time.

To achieve this modify the code in list-employees.component.html file as shown below.
<button
(click)="nextEmployee()"
class="btn
btn-primary">
View Next Employee
</button>
<br/><br/>
<app-employee-display
[employee]="employeeToDisplay">
</app-employee-display>
Modify the code in list-employees.component.ts as shown below.
import
{ Component, OnInit } from '@angular/core';
import
{ Employee } from '../models/employee.model';
import
{ EmployeeService } from './employee.service';
@Component({
templateUrl: './list-employees.component.html',
styleUrls: ['./list-employees.component.css']
})
export
class ListEmployeesComponent implements
OnInit {
employees: Employee[];
employeeToDisplay: Employee;
private arrayIndex = 1;
private arrayIndex = 1;
constructor(private
_employeeService: EmployeeService) { }
ngOnInit() {
this.employees = this._employeeService.getEmployees();
this.employeeToDisplay = this.employees[0];
}
nextEmployee(): void {
if (this.employeeToDisplay.id
<= 2) {
this.employeeToDisplay = this.employees[this.arrayIndex];
this.arrayIndex++;
this.arrayIndex++;
} else {
this.employeeToDisplay = this.employees[0];
this.arrayIndex = 1;
this.arrayIndex = 1;
}
}
}
At this point view the page in the browser. Notice only one employee is displayed. Click "View Next Employee" button to see the next employee. It is the child component (DisplayEmployeeComponent) that displays the employee details. For the child component to be able to display the employee details, the parent component is passing the employee object to the child component using the child component input property.
So every time we click the "View Next Employee" button, the INPUT property value changes. When this happens we want to detect the change and react. Let us say for example, we want to log to the console the previously displayed employee name and the currently displayed employee name.
We can achieve this either by using ngOnChanges life cycle hook or Property Setter. Let's look at both the ways.
Detecting and reacting to Input property changes using ngOnChanges life cycle hook
// This life cycle hook receives
SimpleChanges as an Input parameter
// We can use it to retrieve previous
and current values as shown below
ngOnChanges(changes: SimpleChanges) {
const previousEmployee =
<Employee>changes.employee.previousValue;
const currentEmployee =
<Employee>changes.employee.currentValue;
console.log('Previous : '
+ (previousEmployee ? previousEmployee.name : 'NULL')
);
console.log('Current : '
+ currentEmployee.name);
}
Next Video : Detecting and reacting to Input property changes using Property Setter
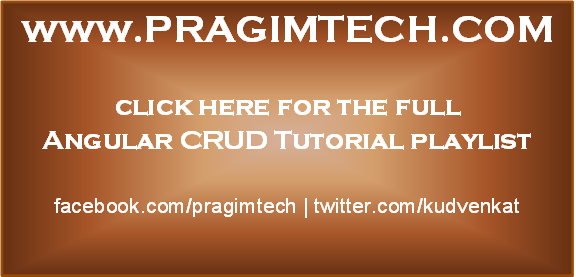
every time we click the "View Next Employee" button, the INPUT property value changes, i m wondering which step the input value got increment. Thanks.
ReplyDeletevalue is not getting incremented if you will see the logic it just replacing the index of employee array and on the basis of index it gives the employee detail. If you will change the id of any employee you will see the different behviour
ReplyDeleteThis code is different than the video tutorial. I have a confusion in this method
ReplyDeletenextEmployee(): void {
if (this.employeeToDisplay.id <= 2) {
this.employeeToDisplay = this.employees[this.employeeToDisplay.id];
} else {
this.employeeToDisplay = this.employees[0];
}
}
I am wondering... how the the increment occur at this line
this.employeeToDisplay = this.employees[this.employeeToDisplay.id];
because in video it is different and understandable , and in blog its differently written.
I will be great if some one clear this magic for all the students ...!
Thanks
check properly the code in blog is same as that in video
Delete//private arrayIndex = 1;
ReplyDeleteif (this.employeeToDispaly.id < this.employees.length) {
this.employeeToDispaly = this.employees[this.employeeToDispaly.id + 1];
}
else {
this.employeeToDispaly = this.employees[0];
}
//private arrayIndex = 1;
Deleteif (this.employeeToDispaly.id < this.employees.length) {
this.employeeToDispaly = this.employees[this.employeeToDispaly.id];
}
else {
this.employeeToDispaly = this.employees[0];
}
This is easier to understand, hope it helps.
ReplyDeleteprivate arrayIndex: number;
nextEmployee(): void {
this.arrayIndex++;
if (this.arrayIndex > 3) {
this.arrayIndex = 1;
}
}