Suggested Videos
Part 30 - Angular 5 services tutorial | Text | Slides
Part 31 - Create operation in angular | Text | Slides
Part 32 - Angular switch case example | Text | Slides
In this video we will discuss how to pass data from the Parent component to Child component using input properties. Let us understand this with an example.
At the moment the ListEmplyeesComponent displays the list of employees you see below.
At the moment, ListEmployeesComponent is doing 3 things
Here is the Angular CLI command you can use to generate the component
ng g c employees\DisplayEmployee --no-spec --flat
Copy and paste the following HTML in display-employee.component.html file
Notice in the HTML above we are binding to the employee property. We need to pass the employee object from the Parent component (ListEmployeesComponent) to this Component (DisplayEmployeeComponent). To make this happen we create an input property in DisplayEmployeeComponent. So modify the code in display-employee.component.ts file as shown below.
Copy and paste the following 2 style classes in display-employee.component.css file. Delete these 2 classes from list-employees.component.css. We do not need them there anymore.
Make the following changes in the parent component (list-employees.component.html). Notice, the child component selector (app-display-employee) is nested in the Parent component. The parent component, loops through each employee and passes the employee object to the child component (DisplayEmployeeComponent) using it's Input property.
Part 30 - Angular 5 services tutorial | Text | Slides
Part 31 - Create operation in angular | Text | Slides
Part 32 - Angular switch case example | Text | Slides
In this video we will discuss how to pass data from the Parent component to Child component using input properties. Let us understand this with an example.
At the moment the ListEmplyeesComponent displays the list of employees you see below.

At the moment, ListEmployeesComponent is doing 3 things
- Calls the Angular EmployeeService to retrieve employees data
- Loops through each employee
- Has all the display logic to display employee details
Here is the Angular CLI command you can use to generate the component
ng g c employees\DisplayEmployee --no-spec --flat
Copy and paste the following HTML in display-employee.component.html file
<div
class="panel
panel-primary">
<div class="panel-heading">
<h3 class="panel-title">{{employee.name}}</h3>
</div>
<div class="panel-body">
<div class="col-xs-10">
<div class="row
vertical-align">
<div class="col-xs-4">
<img
class="imageClass"
[src]="employee.photoPath"
/>
</div>
<div class="col-xs-8">
<div
class="row">
<div
class="col-xs-6">
Gender
</div>
<div
class="col-xs-6">
: {{employee.gender}}
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Date of Birth
</div>
<div
class="col-xs-6">
: {{employee.dateOfBirth | date}}
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Contact Preference
</div>
<div
class="col-xs-6">
: {{employee.contactPreference}}
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Phone
</div>
<div
class="col-xs-6">
: {{employee.phoneNumber}}
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Email
</div>
<div
class="col-xs-6">
: {{employee.email}}
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Department
</div>
<div
class="col-xs-6"
[ngSwitch]="employee.department">
:
<span
*ngSwitchCase="1">
Help Desk </span>
<span
*ngSwitchCase="2">
HR </span>
<span
*ngSwitchCase="3">
IT </span>
<span
*ngSwitchCase="4">
Payroll </span>
<span
*ngSwitchDefault>
N/A </span>
</div>
</div>
<div
class="row">
<div
class="col-xs-6">
Is Active
</div>
<div
class="col-xs-6">
: {{employee.isActive}}
</div>
</div>
</div>
</div>
</div>
</div>
</div>
Notice in the HTML above we are binding to the employee property. We need to pass the employee object from the Parent component (ListEmployeesComponent) to this Component (DisplayEmployeeComponent). To make this happen we create an input property in DisplayEmployeeComponent. So modify the code in display-employee.component.ts file as shown below.
import
{ Component, OnInit, Input } from '@angular/core';
import
{ Employee } from '../models/employee.model';
@Component({
selector: 'app-display-employee',
templateUrl: './display-employee.component.html',
styleUrls: ['./display-employee.component.css']
})
export
class DisplayEmployeeComponent implements
OnInit {
// Parent component will use this Input property to
pass
// the employee object to which the template binds
to
@Input() employee: Employee;
constructor() { }
ngOnInit() {
}
}
Copy and paste the following 2 style classes in display-employee.component.css file. Delete these 2 classes from list-employees.component.css. We do not need them there anymore.
.imageClass{
width:200px;
height:200px;
}
.vertical-align{
display: flex;
align-items: center;
}
Make the following changes in the parent component (list-employees.component.html). Notice, the child component selector (app-display-employee) is nested in the Parent component. The parent component, loops through each employee and passes the employee object to the child component (DisplayEmployeeComponent) using it's Input property.
<div
*ngFor="let employee of
employees">
<app-employee-display
[employee]="employee"></app-employee-display>
</div>
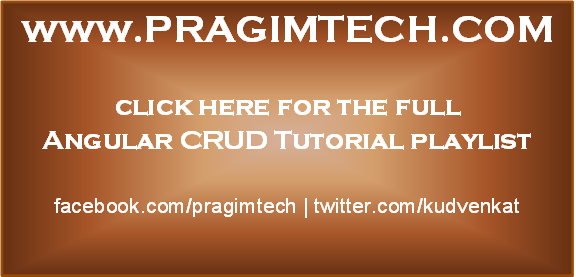
I am getting : Uncaught Error: Template parse errors:
ReplyDeleteCan't bind to 'employee' since it isn't a known property of 'app-employee-display'.
use 'app-display-employee'in last code snippet instead of 'app-employee-display'
ReplyDeleteHi Sir. Please update command
ReplyDeleteng g c employees\DisplayEmployee --no-spec --flat
to
ng g c employees/DisplayEmployee --no-spec --flat
because command giving error
Nice stuff, just correct the typo error in the last, app-employee-display should be app-display-employee
ReplyDeletebdw Thanks for your posts
Hi,
ReplyDeleteI am getting below error.
Uncaught Error: Template parse errors:
'app-employee-display' is not a known element
Please guide what is missing
Reason of the issue:-Selector property of Component decorator is 'app-display-employee' however you are using 'app-employee-display' as directive in list-employees.component.html file. So code should be like this-
Delete