Suggested Videos
Part 29 - Angular form group validation | Text | Slides
Part 30 - Angular 5 services tutorial | Text | Slides
Part 31 - Create operation in angular | Text | Slides
In this video, we will discuss angular ngSwitch directive. Let us understand switch case with an example. Switch case in angular is a combination of 3 directives
Consider the following department data. Depending on the employee's department id we want to display department name.
departments: Department[] = [
{ id: 1, name: 'Help Desk' },
{ id: 2, name: 'HR' },
{ id: 3, name: 'IT' },
{ id: 4, name: 'Payroll' }
];
ngSwitch example
Code Explanation
Part 29 - Angular form group validation | Text | Slides
Part 30 - Angular 5 services tutorial | Text | Slides
Part 31 - Create operation in angular | Text | Slides
In this video, we will discuss angular ngSwitch directive. Let us understand switch case with an example. Switch case in angular is a combination of 3 directives
- ngSwitch directive
- ngSwitchCase directive
- ngSwitchDefault directive
Consider the following department data. Depending on the employee's department id we want to display department name.
departments: Department[] = [
{ id: 1, name: 'Help Desk' },
{ id: 2, name: 'HR' },
{ id: 3, name: 'IT' },
{ id: 4, name: 'Payroll' }
];
ngSwitch example
<div
[ngSwitch]="employee.department">
<span *ngSwitchCase="1">
Help Desk </span>
<span *ngSwitchCase="2">
HR </span>
<span *ngSwitchCase="3">
IT </span>
<span *ngSwitchCase="4">
Payroll </span>
<span *ngSwitchDefault>
N/A </span>
</div>
Code Explanation
- [ngSwitch]="employee.department". The expression (employee.department) returns department id (1, 2 , 3 etc)
- <span *ngSwitchCase="1"> Help Desk </span>. If the department id is 1, then this switchcase is executed and it displays Help Desk
- <span *ngSwitchDefault> N/A </span>. The default switch case is executed if the department id is not 1, 2, 3 and 4
- Jus like ngIf and ngFor, the directives ngSwicthCase and ngSwitchDefault are also structural directives, hence they have an asterisk in front of them
- If multiple ngSwitchCases match the switch expression value, then all those ngSwitchCases are displayed
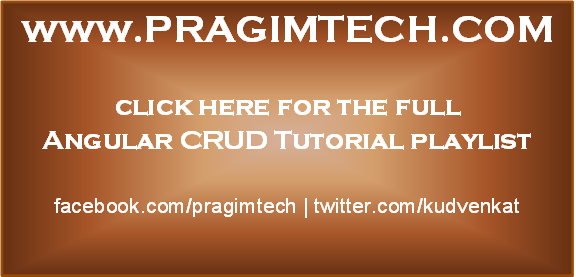
Why declare : departments: Department[] = [ {id: 1, name: 'XX'} ],
ReplyDeleteIt could not be simply: departments =[ {id: 1, name:'Help Desk' }] ?
And How you build the 'employee' ? like this: class employee{ departmentid,nameEmploy,..}