Suggested Videos
Part 27 - Angular password and confirm password validation | Text | Slides
Part 28 - Angular trigger validation manually | Text | Slides
Part 29 - Angular form group validation | Text | Slides
In this video we will discuss creating a service in angular. Whether you are using Angular 2, Angular 4 or Angular 5, the steps to create a service are the same. We discussed the basics of Angular services and why we need services in detail in Parts 25, 27 and 34 of Angular 2 tutorial. If you are new to services in Angular, please check out those videos by clicking here.
Creating a service
Add a new TypeScript file to the "employees" folder and name it employee.service.ts. Copy and paste the following code. At the moment we have the data hard-coded in the service method. In a later video we will discuss retrieving data from a remote server using HTTP.
Registering the service
A service in angular can be registered in a component or in a module. When a service is registered at a component level, then that service is available only to that component and any of it's children. It's not available to the other components.
One the other hand, if we register a service at a module level, then that service is registered with the root injector and available to all the components in our application. We want our EmployeeService to be available in several components (like employee list component, edit component etc). So let's register it in the root module - AppModule.
We discussed dependency injection and injectors in Parts 32, 33, 35 and 36 in Angular 2 tutorial. If you are new to injectors and dependency injection in Angular, please check out those videos by clicking here.
Include the following import statement to import EmployeeService in app.module.ts file
import { EmployeeService } from './employees/employee.service';
Also make sure to include EmployeeService in the providers array of @NgModule decorator.
Injecting and using the service
We need the employee service we created above in ListEmployeesComponent. So let's import and use the Employee service in ListEmployeesComponent. Modify the code in list-employees.component.ts file as shown below.
Important points to remember about Angular service
Part 27 - Angular password and confirm password validation | Text | Slides
Part 28 - Angular trigger validation manually | Text | Slides
Part 29 - Angular form group validation | Text | Slides
In this video we will discuss creating a service in angular. Whether you are using Angular 2, Angular 4 or Angular 5, the steps to create a service are the same. We discussed the basics of Angular services and why we need services in detail in Parts 25, 27 and 34 of Angular 2 tutorial. If you are new to services in Angular, please check out those videos by clicking here.
Creating a service
Add a new TypeScript file to the "employees" folder and name it employee.service.ts. Copy and paste the following code. At the moment we have the data hard-coded in the service method. In a later video we will discuss retrieving data from a remote server using HTTP.
import { Injectable } from '@angular/core';
import { Employee } from '../models/employee.model';
// The @Injectable() decorator is used to inject other dependencies
// into this service. As our service does not have any dependencies
// at the moment, we may remove the @Injectable() decorator and the
// service works exactly the same way. However, Angular recomends
// to always use @Injectable() decorator to ensures consistency
@Injectable()
export class EmployeeService {
private listEmployees: Employee[] = [
{
id: 1,
name: 'Mark',
gender: 'Male',
contactPreference: 'Email',
email: 'mark@pragimtech.com',
dateOfBirth: new Date('10/25/1988'),
department: 'IT',
isActive: true,
photoPath: 'assets/images/mark.png'
},
{
id: 2,
name: 'Mary',
gender: 'Female',
contactPreference: 'Phone',
phoneNumber: 2345978640,
dateOfBirth: new Date('11/20/1979'),
department: 'HR',
isActive: true,
photoPath: 'assets/images/mary.png'
},
{
id: 3,
name: 'John',
gender: 'Male',
contactPreference: 'Phone',
phoneNumber: 5432978640,
dateOfBirth: new Date('3/25/1976'),
department: 'IT',
isActive: false,
photoPath: 'assets/images/john.png'
},
];
getEmployees(): Employee[] {
return this.listEmployees;
}
}
Registering the service
A service in angular can be registered in a component or in a module. When a service is registered at a component level, then that service is available only to that component and any of it's children. It's not available to the other components.
One the other hand, if we register a service at a module level, then that service is registered with the root injector and available to all the components in our application. We want our EmployeeService to be available in several components (like employee list component, edit component etc). So let's register it in the root module - AppModule.
We discussed dependency injection and injectors in Parts 32, 33, 35 and 36 in Angular 2 tutorial. If you are new to injectors and dependency injection in Angular, please check out those videos by clicking here.
Include the following import statement to import EmployeeService in app.module.ts file
import { EmployeeService } from './employees/employee.service';
Also make sure to include EmployeeService in the providers array of @NgModule decorator.
Injecting and using the service
We need the employee service we created above in ListEmployeesComponent. So let's import and use the Employee service in ListEmployeesComponent. Modify the code in list-employees.component.ts file as shown below.
import { Component, OnInit } from '@angular/core';
import { Employee } from '../models/employee.model';
// Import EmployeeService
import { EmployeeService } from './employee.service';
@Component({
templateUrl: './list-employees.component.html',
styleUrls: ['./list-employees.component.css']
})
export class ListEmployeesComponent implements OnInit {
employees: Employee[];
// Inject EmployeeService using the
constructor
// The private variable
_employeeService which points to
// EmployeeService singelton instance
is then available
// throughout the class and can be
accessed using this keyword
constructor(private _employeeService: EmployeeService) { }
// Call the getEmployees() service
method of EmployeeService
// using the private variable
_employeeService
ngOnInit() {
this.employees = this._employeeService.getEmployees();
}
}
Important points to remember about Angular service
- A service in angular is a class
- Irrespective of whether a service has an injected dependency or not, always decorate the angular service class with @Injectable() decorator for consistency and future proof
- If a service is registered at a component level, then that service is available only to that component and to it's children
- If a service is registered at a module level, then that service is available to all the components in the application
- To use a service in a component inject it into the component class constructor
In our next video, we will discuss creating a new Employee.
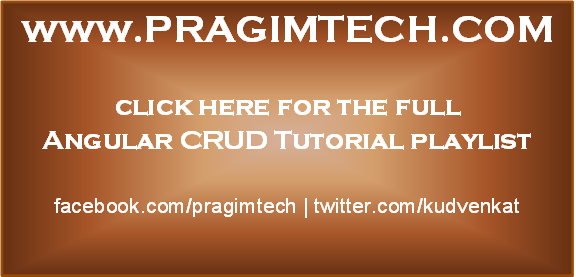
sir, where is list-employees.component.html' ?
ReplyDelete