Suggested Videos
Part 6 - Bootstrap radio buttons in Angular | Text | Slides
Part 7 - Angular radio button checked by default | Text | Slides
Part 8 - Bootstrap checkbox in angular | Text | Slides
In this video we will discuss
Let us understand working with a select element in Angular with a simple example. We want to include "Department" select list as shown in the image below.
Here is the HTML for the "Department" select list
At the moment, we have hard coded the select list options in the HTML. In our next video we will discuss, how to get the select list options from the component class. Notice each option also has a corresponding value. The value is the department id which is what we want to save in the database table when the form is submitted. We will discuss, saving the data to a database table in a later video.
At this point, when we select an option, notice the corresponding option value is included against the "department" property in the Angular auto-generated form model.
Also notice, when we click the "Save" button, the "department" property along with the selected option value is logged to the console in browser developer tools.
How to have one of the select list option selected by default
If we include "selected" attribute on one of the options of the select list, we expect that option to be selected by default when the form initially loads. In the example below, we have included the "selected" attribute on the "IT" option, but when the form reloads, the "IT" option is not selected.
<option value="3" selected>IT</option>
If you remove the "ngModel" directive from the select list, then the the "IT" option gets selected as expected. Notice the "ngModel" directive is removed from the select list.
In Angular, we use "ngModel" directive for two-way data binding. So the moment we put it back in place the "selected" attribute does not work. To make it work include "department" property in the component class and initialise it with one of the option value which you want to have selected by default. In our case, we want the "IT" option to be selected by default. The "IT" option value is "3". So, I have initialised "department" property with a value of '3'
department = '3'
At this point you will have "IT" option selected by default when the form loads. Now, even if we remove the "selected" attribute from the "IT" option, it is still selected by default when the form loads. This is because of the two-way data binding that we get with "ngModel" directive.
How to disable a select list : To disable a select element, use the disabled attribute
Another important point to keep in mind. By default, disabled form controls are not included in the Angular auto generated form model. Since, the "department" select element is disabled, it will not be included in the Angular generated form model.
In our form, we do not want the select element to be disabled, so please remove the disabled attribute. Also, we do not want any option to be selected by default, so remove the "department" property from the component class.
In our next video, we will discuss, how to get the select list options from the component class, instead of having them hard-coded in the HTML.
Part 6 - Bootstrap radio buttons in Angular | Text | Slides
Part 7 - Angular radio button checked by default | Text | Slides
Part 8 - Bootstrap checkbox in angular | Text | Slides
In this video we will discuss
- Working with a select list in Angular Template Driven forms
- How to have one of the select list option selected by default
- How to disable select list
Let us understand working with a select element in Angular with a simple example. We want to include "Department" select list as shown in the image below.

Here is the HTML for the "Department" select list
<div
class="form-group">
<label for="department">Department</label>
<select id="department"
name="department"
[(ngModel)]="department"
class="form-control">
<option value="1">Help
Desk</option>
<option value="2">HR</option>
<option value="3">IT</option>
<option value="4">Paroll</option>
</select>
</div>
At the moment, we have hard coded the select list options in the HTML. In our next video we will discuss, how to get the select list options from the component class. Notice each option also has a corresponding value. The value is the department id which is what we want to save in the database table when the form is submitted. We will discuss, saving the data to a database table in a later video.
At this point, when we select an option, notice the corresponding option value is included against the "department" property in the Angular auto-generated form model.

Also notice, when we click the "Save" button, the "department" property along with the selected option value is logged to the console in browser developer tools.

How to have one of the select list option selected by default
If we include "selected" attribute on one of the options of the select list, we expect that option to be selected by default when the form initially loads. In the example below, we have included the "selected" attribute on the "IT" option, but when the form reloads, the "IT" option is not selected.
<option value="3" selected>IT</option>
If you remove the "ngModel" directive from the select list, then the the "IT" option gets selected as expected. Notice the "ngModel" directive is removed from the select list.
<div
class="form-group">
<label for="department">Department</label>
<select id="department"
name="department"
class="form-control">
<option value="1">Help
Desk</option>
<option value="2">HR</option>
<option value="3"
selected>IT</option>
<option value="4">Paroll</option>
</select>
</div>
In Angular, we use "ngModel" directive for two-way data binding. So the moment we put it back in place the "selected" attribute does not work. To make it work include "department" property in the component class and initialise it with one of the option value which you want to have selected by default. In our case, we want the "IT" option to be selected by default. The "IT" option value is "3". So, I have initialised "department" property with a value of '3'
department = '3'
At this point you will have "IT" option selected by default when the form loads. Now, even if we remove the "selected" attribute from the "IT" option, it is still selected by default when the form loads. This is because of the two-way data binding that we get with "ngModel" directive.
How to disable a select list : To disable a select element, use the disabled attribute
<select
id="department"
name="department"
[(ngModel)]="department"
class="form-control"
disabled>
Another important point to keep in mind. By default, disabled form controls are not included in the Angular auto generated form model. Since, the "department" select element is disabled, it will not be included in the Angular generated form model.
In our form, we do not want the select element to be disabled, so please remove the disabled attribute. Also, we do not want any option to be selected by default, so remove the "department" property from the component class.
In our next video, we will discuss, how to get the select list options from the component class, instead of having them hard-coded in the HTML.
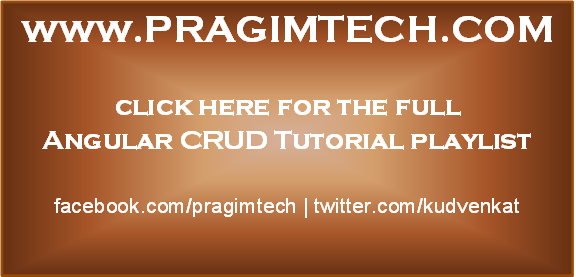
When every code is written to display controls on view template, how come the FORM becomes "Angular auto generated form model". Can someone explain?
ReplyDelete