Suggested Videos
Part 3 - Angular routing and navigation | Text | Slides
Part 4 - Angular base href | Text | Slides
Part 5 - Angular forms tutorial | Text | Slides
In this video we will discuss working with radio buttons in Angular Template Driven forms.
We want to include "Gender" radio buttons in the Create Employee form as shown below. When we select employee "Gender" using the radio buttons, the selected gender value should reflect in the Angular generated form model as shown in the image below. Also, we we click the "Save" button we want the selected gender value to be logged to the console.
To achieve this all you have to do is include the following HTML in create-employee.component.html file
Code Explanation
For your referece, here is the complete HTML in create-employee.component.html
Part 3 - Angular routing and navigation | Text | Slides
Part 4 - Angular base href | Text | Slides
Part 5 - Angular forms tutorial | Text | Slides
In this video we will discuss working with radio buttons in Angular Template Driven forms.
We want to include "Gender" radio buttons in the Create Employee form as shown below. When we select employee "Gender" using the radio buttons, the selected gender value should reflect in the Angular generated form model as shown in the image below. Also, we we click the "Save" button we want the selected gender value to be logged to the console.

To achieve this all you have to do is include the following HTML in create-employee.component.html file
<div
class="form-group">
<label>Gender</label>
<div class="form-control">
<label class="radio-inline">
<input type="radio"
name="gender"
value="male"
[(ngModel)]="gender">
Male
</label>
<label class="radio-inline">
<input type="radio"
name="gender"
value="female"
[(ngModel)]="gender">
Female
</label>
</div>
</div>
Code Explanation
- The name attribute is required to group the radio buttons as one unit and make the selection mutually exclusive. Make sure both the radio buttons have the same value for the "name" attribute. Otherwise the radio button selection won't be mutually exclusive.
- It is also important that you set the "value" attribute for each radio button. This value is posted to the server when the form is submitted.

For your referece, here is the complete HTML in create-employee.component.html
<form
#employeeForm="ngForm"
(ngSubmit)="saveEmployee(employeeForm)">
<div class="panel
panel-primary">
<div class="panel-heading">
<h3 class="panel-title">Create
Employee</h3>
</div>
<div class="panel-body">
<div class="form-group">
<label for="fullName">Full
Name</label>
<input id="fullName"
type="text"
class="form-control"
name="fullName"
[(ngModel)]="fullName">
</div>
<div class="form-group">
<label for="email">Email</label>
<input id="email"
type="text"
class="form-control"
name="email"
[(ngModel)]="email">
</div>
<div class="form-group">
<label
for="phoneNumber">Phone
Number</label>
<input id="phoneNumber"
type="text"
class="form-control"
name="phoneNumber"
[(ngModel)]="phoneNumber">
</div>
<div class="form-group">
<label>Contact Preference</label>
<div class="form-control">
<label
class="radio-inline">
<input
type="radio"
name="contactPreference"
value="email"
[(ngModel)]="contactPreference">
Email
</label>
<label
class="radio-inline">
<input
type="radio"
name="contactPreference"
value="phone"
[(ngModel)]="contactPreference">
Phone
</label>
</div>
</div>
<div class="form-group">
<label>Gender</label>
<div class="form-control">
<label
class="radio-inline">
<input
type="radio"
name="gender"
value="male"
[(ngModel)]="gender">
Male
</label>
<label
class="radio-inline">
<input
type="radio"
name="gender"
value="female"
[(ngModel)]="gender">
Female
</label>
</div>
</div>
</div>
<div class="panel-footer">
<button class="btn
btn-primary" type="submit">Save</button>
</div>
</div>
</form>
Angular Generated Forom Model :
{{employeeForm.value | json}}
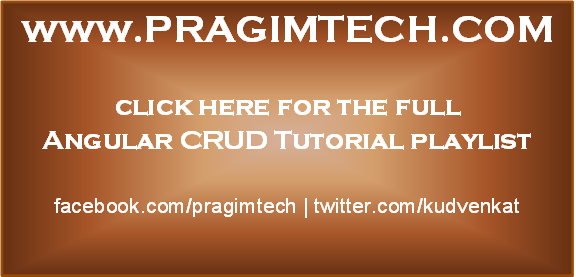
i am using bootstrap 3 with angular 6 so these code not giving me proper format so how how to make same format form in angular6 with bosstrap3
ReplyDeleteemployee.componenent.ts
ReplyDeleteimport { Component } from '@angular/core';
import { NgForm } from '@angular/forms';
@Component({
selector: 'app-create-employee',
templateUrl: './create-employee.component.html',
styleUrls: ['./create-employee.component.scss']
})
export class CreateEmployeeComponent {
fullName:string="";
email:string="";
gender!:boolean;
saveEmployee(employeeForm: NgForm): void {
console.log(employeeForm.form);
}
}