Suggested Videos
Part 2 - Setting up Angular 2 in Visual Studio | Text | Slides
Part 3 - Run angular 2 app using f5 from visual studio | Text | Slides
Part 4 - Angular 2 components | Text | Slides
In this video we will discuss template and templateurl properties of the Component decorator. This is continuation to Part 4, please watch Part 4 from Angular 2 tutorial before proceeding.
In Part 4, we have used an inline view template. Notice the code we have implemented in app.component.ts file. We have embedded the view template inline in the .ts file.
import { Component
} from '@angular/core';
@Component({
selector: 'my-app',
template: `<h1>Hello {{name }}</h1>`
})
export class AppComponent {
name: string = 'Angular';
}
The view template is inline in a pair of backtick characters. The first question that comes to our mind is can't we include the HTML in a pair of single or double quotes. The answer is "YES" we can as long as the HTML is in a single line. So this means the above code can be rewritten using a pair of single quotes as shown below.
template: '<h1>Hello {{name }}</h1>'
We can also replace the pair of single quotes with a pair of double quotes as shown below, and the application still continues to work exactly the same way as before.
template: "<h1>Hello {{name }}</h1>"
The obvious next question that comes to our mind is when should we use backticks instead of single or doublequotes
If you have the HTML in more than one line, then you have to use backticks instead of single or double quotes as shown below. If you use single or double quotes instead of backticks you will get an error.
template: `<h1>
Hello {{name }}
</h1>`
Instead of using an inline view template, you can have it in a separate HTML file. Here are the steps to have the view template in a separate HTML file
Step 1 : Right click on the "app" folder and add a new HTML file. Name it "app.component.html".
Step 2 : Include the following HTML in "app.component.html"
<h1>
Hello {{name}}
</h1>
Step 3 : In "app.component.ts", reference the external view template using templateUrl property as shown below. Notice instead of the "template" property we are using "templateUrl" property. Please note that templateUrl path is relative to index.html
import { Component
} from '@angular/core';
@Component({
selector: 'my-app',
templateUrl:
'app/app.component.html'
})
export class AppComponent {
name: string = "Angular";
}
What are the differences between template and templateUrl properties and when to use one over the other
Angular2 recommends to extract templates into a separate file, if the view template is longer than 3 lines. Let's understand why is it better to extract a view template into a seprate file, if it is longer than 3 lines.
With an inline template
- We loose Visual Studio editor intellisense, code-completion and formatting features.
- TypeScript code is not easier to read and understand when it is mixed with the inline template HTML.
- We have Visual Studio editor intellisense, code-completion and formatting features and
- Not only the code in "app.component.ts" is clean, it is also easier to read and understand
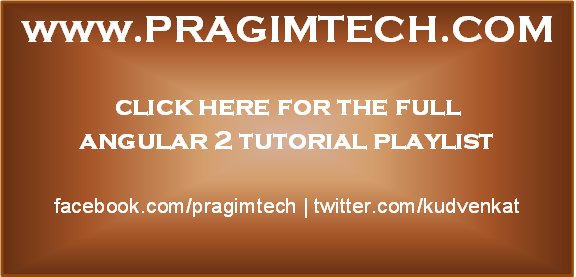
when i am binding the angular expression same html code is showing
ReplyDeleteon excuting the page angular not binding the data.
output:
Hello{{name}}
i think you are directly running the app.component.html file. Try running index.html.
ReplyDeleteall lecture are very good
ReplyDeleteYes, these are in simple language with good understanding..
ReplyDelete