Suggested Videos:
Part 11 - What is the difference between is and as keyword in c#
Part 12 - Difference between int and Int32 in c#
Part 13 - Reverse each word in a string using c#
One of our YouTube channel subscribers faced this question in an interview for Dot Net developer role. The interview question was asked as follows.
An abstract class has a default implementation for a method. The method's default implementation is needed in some class but not in some other class. How can you achieve it?
The answer to this question is very simple. If you know what a virtual method is and it's purpose, then you already know the answer.
Let us understand the answer to this question with an example.
Points to remember
Part 11 - What is the difference between is and as keyword in c#
Part 12 - Difference between int and Int32 in c#
Part 13 - Reverse each word in a string using c#
One of our YouTube channel subscribers faced this question in an interview for Dot Net developer role. The interview question was asked as follows.
An abstract class has a default implementation for a method. The method's default implementation is needed in some class but not in some other class. How can you achieve it?
The answer to this question is very simple. If you know what a virtual method is and it's purpose, then you already know the answer.
Let us understand the answer to this question with an example.
public abstract class AbstractClass
{
public virtual void AbstractClassMethod()
{
Console.WriteLine("Default
Implementation");
}
}
public class SomeClass : AbstractClass
{ }
public class SomeOtherClass : AbstractClass
{
public override void AbstractClassMethod()
{
Console.WriteLine("New
Implementation");
}
}
class Program
{
public static void Main()
{
SomeClass sc = new SomeClass();
sc.AbstractClassMethod();
SomeOtherClass soc = new SomeOtherClass();
soc.AbstractClassMethod();
}
}
Points to remember
- In the AbstractClass we created a virtual method with a default implementation.
- Any class that wants the default implementation can simply use it by inheriting from the abstract class. In our case SomeClass inherits from AbstractClass and does not override the default implementation of the virtual method in the base class. This means when we create an instance of SomeClass and call the virtual method the class gets the default implementation of the virtual method.
- On the other hand if a class does not need the default implementation, that class can inherit from the abstract class and override the default implementation. In our example, SomeOtherClass overrides the virtual method it inherited from the base abstract class. This means when we create an instance of SomeOtherClass and call the virtual method the class gets the new implementation and not the default implementation.
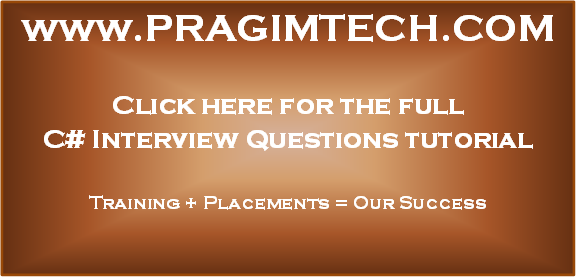
Sir, this is karthik. i'm a great fan of your channel previously you are uploading the web api tutorial & uploaded 19 tutorials successfully. I followed and learned the topic which you shared. Now i'm waiting for the new upload. please upload the continuation of the topic. Thanking you
ReplyDeletei faced this question when i passed C# exam certificate
ReplyDeleteHi Sir,
ReplyDeleteCould You please make videos on these topics which were asked in intervies,
Idisposeable,
Garbage collection Cycle,
how Using section works internally
Diferrence between managed and unmanaged,
Extension Methods,
Operator overloading
Thank You