Suggested Videos:
Part 8 - Can an abstract class have a constructor
Part 9 - Call an abstract method from an abstract class constructor
Part 10 - What happens if finally block thows an exception
What is the difference between is and as keyword in c#
The is operator returns true if an object can be cast to a specific type, otherwise false.
Example 1:
Example 2:
The as operator attempts to cast an object to a specific type, and returns null if it fails.
Example 1:
Example 2:
Example 3:
Another way to cast an object is by using the cast operator. The cast operator throws an exception if the cast fails.
Example:
The above program will throw the following exception
System.InvalidCastException: Unable to cast object of type'Demo.Employee' to type 'Demo.PermanentEmployee'
So, what is the difference between Cast operator and as operator?
as operator will not throw an exception if the cast fails, where as, cast operator will throw an exception.
Part 8 - Can an abstract class have a constructor
Part 9 - Call an abstract method from an abstract class constructor
Part 10 - What happens if finally block thows an exception
What is the difference between is and as keyword in c#
The is operator returns true if an object can be cast to a specific type, otherwise false.
Example 1:
using System;
namespace Demo
{
class Program
{
static void Main()
{
Employee emp = new Employee
{
ID = 101,
Name = "Mark"
};
// Returns true
if (emp is Employee)
{
Console.WriteLine(emp.Name +
" is Employee");
}
else
{
Console.WriteLine(emp.Name +
" is not Employee");
}
// Returns false, as the base type
cannot be converted to derived type
if (emp is PermanentEmployee)
{
Console.WriteLine(emp.Name +
" is PermanentEmployee");
}
else
{
Console.WriteLine(emp.Name +
" is not PermanentEmployee");
}
// Returns false, as the base type
cannot be converted to derived type
if (emp is ContractEmployee)
{
Console.WriteLine(emp.Name +
" is ContractEmployee");
}
else
{
Console.WriteLine(emp.Name +
" is not ContractEmployee");
}
}
}
class Employee
{
public int ID
{ get; set;
}
public string Name
{ get; set;
}
}
class PermanentEmployee : Employee
{
public int AnnualSalary
{ get; set;
}
}
class ContractEmployee : Employee
{
public int HourlySalary
{ get; set;
}
}
}
Example 2:
using System;
namespace Demo
{
class Program
{
static void Main()
{
Employee emp = new PermanentEmployee
{
ID = 101,
Name = "Mark"
};
// Returns true as the derived type
can be converted to base type
if (emp is Employee)
{
Console.WriteLine(emp.Name +
" is Employee");
}
else
{
Console.WriteLine(emp.Name +
" is not Employee");
}
// Returns true, as the actual object
is of type PermanentEmployee
if (emp is PermanentEmployee)
{
Console.WriteLine(emp.Name +
" is PermanentEmployee");
}
else
{
Console.WriteLine(emp.Name +
" is not PermanentEmployee");
}
// Returns false, as PermanentEmployee
object // cannot be converted to ContractEmployee
if (emp is ContractEmployee)
{
Console.WriteLine(emp.Name +
" is ContractEmployee");
}
else
{
Console.WriteLine(emp.Name +
" is not ContractEmployee");
}
}
}
class Employee
{
public int ID
{ get; set;
}
public string Name
{ get; set;
}
}
class PermanentEmployee : Employee
{
public int AnnualSalary
{ get; set;
}
}
class ContractEmployee : Employee
{
public int HourlySalary
{ get; set;
}
}
}
The as operator attempts to cast an object to a specific type, and returns null if it fails.
Example 1:
using System;
namespace Demo
{
class Program
{
static void Main()
{
Employee emp = new Employee
{
ID = 101,
Name = "Mark"
};
PermanentEmployee permanentEmployee = emp
as PermanentEmployee;
if (permanentEmployee == null)
{
Console.WriteLine("permanentEmployee is NULL");
}
else
{
Console.WriteLine("permanentEmployee is not NULL");
}
ContractEmployee contractEmployee = emp
as ContractEmployee;
if (contractEmployee == null)
{
Console.WriteLine("contractEmployee is NULL");
}
else
{
Console.WriteLine("contractEmployee is not NULL");
}
}
}
class Employee
{
public int ID
{ get; set;
}
public string Name
{ get; set;
}
}
class PermanentEmployee : Employee
{
public int AnnualSalary
{ get; set;
}
}
class ContractEmployee : Employee
{
public int HourlySalary
{ get; set;
}
}
}
Example 2:
using System;
namespace Demo
{
class Program
{
static void Main()
{
Employee emp = new PermanentEmployee
{
ID = 101,
Name = "Mark"
};
PermanentEmployee permanentEmployee = emp
as PermanentEmployee;
if (permanentEmployee == null)
{
Console.WriteLine("permanentEmployee is NULL");
}
else
{
Console.WriteLine("permanentEmployee is not NULL");
}
ContractEmployee contractEmployee = emp
as ContractEmployee;
if (contractEmployee == null)
{
Console.WriteLine("contractEmployee is NULL");
}
else
{
Console.WriteLine("contractEmployee is not NULL");
}
}
}
class Employee
{
public int ID
{ get; set;
}
public string Name
{ get; set;
}
}
class PermanentEmployee : Employee
{
public int AnnualSalary
{ get; set;
}
}
class ContractEmployee : Employee
{
public int HourlySalary
{ get; set;
}
}
}
Example 3:
using System;
namespace Demo
{
class Program
{
static void Main()
{
PermanentEmployee emp1 = new PermanentEmployee
{
ID = 101,
Name = "Mark"
};
ContractEmployee emp2 = new ContractEmployee
{
ID = 102,
Name = "Mary"
};
Employee employee1 = emp1 as Employee;
if (employee1 == null)
{
Console.WriteLine("employee1 is NULL");
}
else
{
Console.WriteLine("employee1 is not NULL");
}
Employee employee2 = emp2
as Employee;
if (employee2 == null)
{
Console.WriteLine("employee2 is NULL");
}
else
{
Console.WriteLine("employee2 is not NULL");
}
}
}
class Employee
{
public int ID
{ get; set;
}
public string Name
{ get; set;
}
}
class PermanentEmployee : Employee
{
public int AnnualSalary
{ get; set;
}
}
class ContractEmployee : Employee
{
public int HourlySalary
{ get; set;
}
}
}
Another way to cast an object is by using the cast operator. The cast operator throws an exception if the cast fails.
Example:
using System;
namespace Demo
{
class Program
{
static void Main()
{
Employee emp = new Employee
{
ID = 101,
Name = "Mark"
};
PermanentEmployee permanentEmployee = (PermanentEmployee)emp;
}
}
class Employee
{
public int ID
{ get; set;
}
public string Name
{ get; set;
}
}
class PermanentEmployee : Employee
{
public int AnnualSalary
{ get; set;
}
}
}
The above program will throw the following exception
System.InvalidCastException: Unable to cast object of type'Demo.Employee' to type 'Demo.PermanentEmployee'
So, what is the difference between Cast operator and as operator?
as operator will not throw an exception if the cast fails, where as, cast operator will throw an exception.
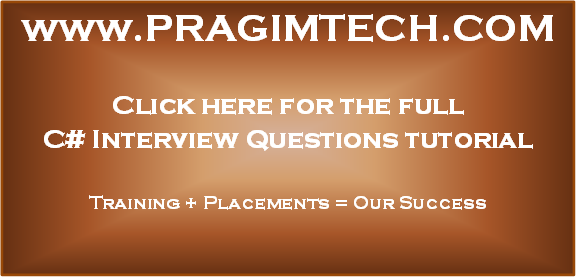
No comments:
Post a Comment
It would be great if you can help share these free resources