Suggested Videos
Part 40 - AngularJS optional url parameters
Part 41 - AngularJS route resolve
Part 42 - AngularJS ui-router tutorial
In this video we will discuss configuring states in an angular application. According to angular documentation, a state corresponds to a "place" in the application.
To configure a state use state() method of $stateProvider service. state() method has 2 parameters
i) name - A unique state name, e.g. "home", "courses", "students"
ii) stateConfig - State configuration object
State configuration object has several properties. Some of them are listed below.
template
templateUrl
controller
controllerAs
resolve
url
Let us now configure states for the sample application that we have been working with. We will configure home, courses and students states. Here are the steps
Step 1 : Modify the code in config() function in script.js as shown below. Notice we are using state() method of $stateProvider service to configure states.
Step 2 : Modify studentsController function in script.js as shown below. Notice instead of $route service we are injecting $state service. We used $route service reload() method to reload just the current route instead of the entire app. $route service is in ngRoute module. Since we removed ngRoute module from our application, we cannot use $route service. Instead let's use $state service. Notice to reload just the current state we are using reload() method of the $state service.
Step 3 : Modify the links in index.html (layout view) as shown below. Notice we are using ui-sref to create links with UI-Router. ui-sref points to the state of the application. sref stands for state reference. You may also use href to create links and activate a state. Yet another method available to activate a state is by using state service go() method. We will discuss different ways to activate state in a later video. For now let us use ui-sref attribute.
At this point, home, courses and students links should work as expected. The other links are broken. We will discuss fixing these in our upcoming videos.
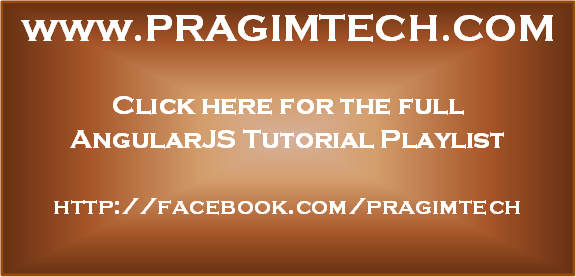
Part 40 - AngularJS optional url parameters
Part 41 - AngularJS route resolve
Part 42 - AngularJS ui-router tutorial
In this video we will discuss configuring states in an angular application. According to angular documentation, a state corresponds to a "place" in the application.
To configure a state use state() method of $stateProvider service. state() method has 2 parameters
i) name - A unique state name, e.g. "home", "courses", "students"
ii) stateConfig - State configuration object
State configuration object has several properties. Some of them are listed below.
template
templateUrl
controller
controllerAs
resolve
url
Let us now configure states for the sample application that we have been working with. We will configure home, courses and students states. Here are the steps
Step 1 : Modify the code in config() function in script.js as shown below. Notice we are using state() method of $stateProvider service to configure states.
var app = angular
.module("Demo", ["ui.router"])
.config(function ($stateProvider) {
$stateProvider
.state("home", {
url: "/home",
templateUrl: "Templates/home.html",
controller: "homeController",
controllerAs: "homeCtrl"
})
.state("courses", {
url: "/courses",
templateUrl: "Templates/courses.html",
controller: "coursesController",
controllerAs: "coursesCtrl"
})
.state("students", {
url: "/students",
templateUrl: "Templates/students.html",
controller: "studentsController",
controllerAs: "studentsCtrl",
resolve: {
studentslist: function ($http, $location) {
return $http.get("StudentService.asmx/GetAllStudents")
.then(function (response) {
return response.data;
})
}
}
})
})
.controller("studentsController", function (studentslist, $state,
$location) {
var vm = this;
vm.studentSearch = function
() {
if (vm.name)
$location.url("/studentsSearch/" + vm.name)
else
$location.url("/studentsSearch")
}
vm.reloadData = function () {
$state.reload();
}
vm.students = studentslist;
})
Step 3 : Modify the links in index.html (layout view) as shown below. Notice we are using ui-sref to create links with UI-Router. ui-sref points to the state of the application. sref stands for state reference. You may also use href to create links and activate a state. Yet another method available to activate a state is by using state service go() method. We will discuss different ways to activate state in a later video. For now let us use ui-sref attribute.
<a ui-sref="home">Home</a>
<a ui-sref="courses">Courses</a>
<a ui-sref="students">Students</a>
At this point, home, courses and students links should work as expected. The other links are broken. We will discuss fixing these in our upcoming videos.
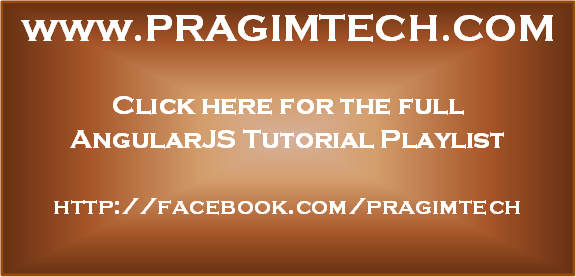
hi,
ReplyDeleteI am stuck in this place. the ui router is not working for me. I am using VS2013. everytime i use , it gives me an error. please assist.
need also to change the to
ReplyDelete