Suggested Videos
Part 37 - Difference between $scope and $rootScope
Part 38 - AngularJS cancel route change
Part 39 - AngularJS route change events
In this video we will discuss angular optional URL parameters. Let us understand this with an example. Here is what we want to do.
On the list of students page, we want to search employees by name.
For example if we type "Ma" and click search button, on the subsequent page we want to display all the student names that start with "Ma" as shown below.
Here are the steps to achieve this.
Step 1 : Add a method to the asp.net web service to retrieve students by name from the database.
Step 2 : Modify studentsController function in script.js to include studentSearch() function. This function needs to be called when the Search button is clicked.
Step 3 : Modify students.html partial template in Templates folder as shown below.
Step 4 : Add studentsSearchController function in script.js
Step 5 : Include a new route for searching students in script.js. To make a parameter optional, all you have to do is include a "?" at the end.
Step 6 : Add a new HTML file to the Templates folder. Name it studentsSearch.html. Copy and paste the following HTML.
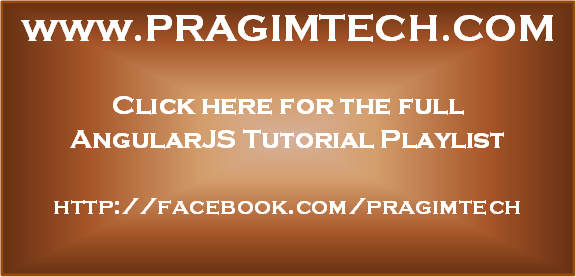
Part 37 - Difference between $scope and $rootScope
Part 38 - AngularJS cancel route change
Part 39 - AngularJS route change events
In this video we will discuss angular optional URL parameters. Let us understand this with an example. Here is what we want to do.
On the list of students page, we want to search employees by name.
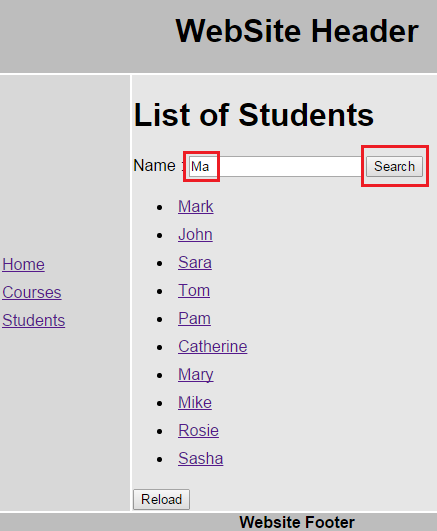
For example if we type "Ma" and click search button, on the subsequent page we want to display all the student names that start with "Ma" as shown below.
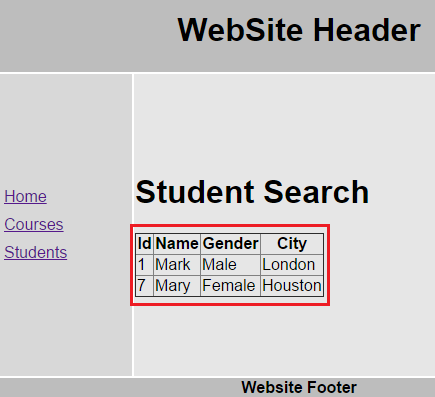
Here are the steps to achieve this.
Step 1 : Add a method to the asp.net web service to retrieve students by name from the database.
[WebMethod]
public void GetStudentsByName(string name)
{
List<Student> listStudents = new List<Student>();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd =
new
SqlCommand("Select * from
tblStudents where name like @name", con);
SqlParameter param = new SqlParameter()
{
ParameterName = "@name",
Value = name + "%"
};
cmd.Parameters.Add(param);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Student student = new
Student();
student.id = Convert.ToInt32(rdr["Id"]);
student.name = rdr["Name"].ToString();
student.gender = rdr["Gender"].ToString();
student.city = rdr["City"].ToString();
listStudents.Add(student);
}
}
JavaScriptSerializer js = new JavaScriptSerializer();
Context.Response.Write(js.Serialize(listStudents));
}
Step 2 : Modify studentsController function in script.js to include studentSearch() function. This function needs to be called when the Search button is clicked.
.controller("studentsController", function ($http, $route, $location) {
var vm = this;
vm.studentSearch = function
() {
if (vm.name)
$location.url("/studentsSearch/" + vm.name)
else
$location.url("/studentsSearch")
}
vm.reloadData = function () {
$route.reload();
}
$http.get("StudentService.asmx/GetAllStudents")
.then(function (response) {
vm.students =
response.data;
})
})
Step 3 : Modify students.html partial template in Templates folder as shown below.
<h1>List
of Students</h1>
Name : <input type="text" ng-model="studentsCtrl.name" />
<button ng-click="studentsCtrl.studentSearch()">Search</button>
<ul>
<li ng-repeat="student in
studentsCtrl.students">
<a href="students/{{student.id}}">
{{student.name}}
</a>
</li>
</ul>
<button ng-click="studentsCtrl.reloadData()">Reload</button>
Step 4 : Add studentsSearchController function in script.js
.controller("studentsSearchController", function ($http, $routeParams) {
var vm = this;
if ($routeParams.name) {
$http({
url: "StudentService.asmx/GetStudentsByName",
method: "get",
params: { name: $routeParams.name }
}).then(function (response) {
vm.students = response.data;
})
}
else {
$http.get("StudentService.asmx/GetAllStudents")
.then(function (response) {
vm.students =
response.data;
})
}
})
Step 5 : Include a new route for searching students in script.js. To make a parameter optional, all you have to do is include a "?" at the end.
.when("/studentsSearch/:name?", {
templateUrl: "Templates/studentsSearch.html",
controller: "studentsSearchController",
controllerAs: "studentsSearchCtrl"
})
<h1>Student
Search</h1>
<table border="1" style="border-collapse:collapse">
<thead>
<tr>
<th>Id</th>
<th>Name</th>
<th>Gender</th>
<th>City</th>
</tr>
</thead>
<tr ng-repeat="student in
studentsSearchCtrl.students">
<td>{{student.id}}</td>
<td>{{student.name}}</td>
<td>{{student.gender}}</td>
<td>{{student.city}}</td>
</tr>
</table>
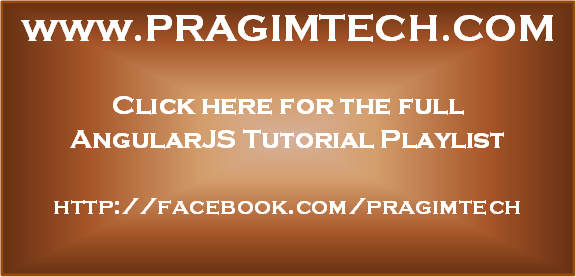
No comments:
Post a Comment
It would be great if you can help share these free resources