Suggested Videos
Part 35 - AngularJS caseInsensitiveMatch and Inline Templates
Part 36 - AngularJS route reload
Part 37 - Difference between $scope and $rootScope
In this video we will discuss, how to cancel route change in Angular with an example. This is extremely useful if you want to warn a user when they are navigating away from a page with unsaved changes.
For our example, let us assume that the user is currently on this students page. When he click on any other links (Home or Courses on the left), we want to present the user with a confirmation box (Are you sure you want to navigate away from this page). If the user clicks OK, the user will be redirected to the new route. If cancel is clicked, route navigation should be cancelled and the user should stay on the students page.
Handle $routeChangeStart event in studentsController function
Notice
1. We are injecting $scope object into the controller function
2. With in $routeChangeStart event handler function, we are using confirm() function to display the confirmation dialog box
3. When the confirmation dialog box is displayed, If the user clicks Cancel, event.preventDefault() is called and it cancels the route change, so the user stays on the same page
4. On the other hand if the user clicks OK, event.preventDefault() is not called and the route is allowed to change.
If you want to include the route that the user is trying to navigate to in the confirmation message you can do so by using next parameter of the $routeChangeStart event handler function as shown below.
With this change in place and while you are on students page and if you click on Home link on the left menu, you will get the following message.
At this point, you might be thinking, how do I know next parameter has $$route.originalPath property. Well you can log the next parameter and see all that properties it has. We will discuss how to do this in our next video.
You can also cancel route change by handling $locationChangeStart event
The next and current parameters of $locationChangeStart event handler has the complete next and current URLs. So when you click on home while on students page, you will see the following message.
In our next video we will discuss the different events that are triggered when a route change occurs in an angular application.
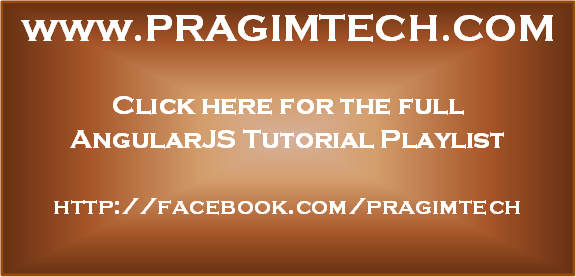
Part 35 - AngularJS caseInsensitiveMatch and Inline Templates
Part 36 - AngularJS route reload
Part 37 - Difference between $scope and $rootScope
In this video we will discuss, how to cancel route change in Angular with an example. This is extremely useful if you want to warn a user when they are navigating away from a page with unsaved changes.
For our example, let us assume that the user is currently on this students page. When he click on any other links (Home or Courses on the left), we want to present the user with a confirmation box (Are you sure you want to navigate away from this page). If the user clicks OK, the user will be redirected to the new route. If cancel is clicked, route navigation should be cancelled and the user should stay on the students page.
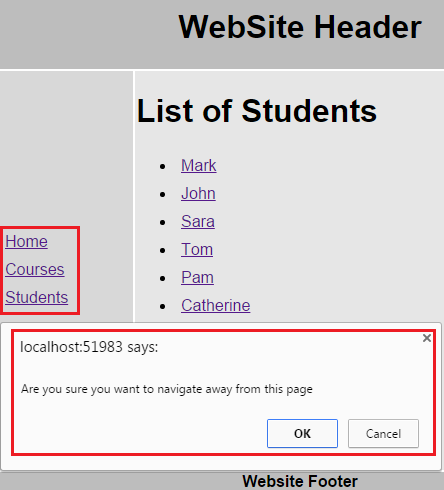
Handle $routeChangeStart event in studentsController function
.controller("studentsController", function ($http, $route, $scope) {
var vm = this;
$scope.$on("$routeChangeStart", function (event, next, current) {
if (!confirm("Are you sure you want
to navigate away from this page")) {
event.preventDefault();
}
});
vm.reloadData = function () {
$route.reload();
}
$http.get("StudentService.asmx/GetAllStudents")
.then(function (response) {
vm.students = response.data;
})
})
1. We are injecting $scope object into the controller function
2. With in $routeChangeStart event handler function, we are using confirm() function to display the confirmation dialog box
3. When the confirmation dialog box is displayed, If the user clicks Cancel, event.preventDefault() is called and it cancels the route change, so the user stays on the same page
4. On the other hand if the user clicks OK, event.preventDefault() is not called and the route is allowed to change.
If you want to include the route that the user is trying to navigate to in the confirmation message you can do so by using next parameter of the $routeChangeStart event handler function as shown below.
.controller("studentsController", function ($http, $route, $scope) {
var vm = this;
$scope.$on("$routeChangeStart", function (event, next, current) {
if (!confirm("Are you sure you want
to navigate away from this page to "
+ next.$$route.originalPath))
{
event.preventDefault();
}
});
vm.reloadData = function () {
$route.reload();
}
$http.get("StudentService.asmx/GetAllStudents")
.then(function (response) {
vm.students =
response.data;
})
})
With this change in place and while you are on students page and if you click on Home link on the left menu, you will get the following message.

At this point, you might be thinking, how do I know next parameter has $$route.originalPath property. Well you can log the next parameter and see all that properties it has. We will discuss how to do this in our next video.
You can also cancel route change by handling $locationChangeStart event
.controller("studentsController", function ($http, $route, $scope) {
var vm = this;
$scope.$on("$locationChangeStart", function (event, next, current) {
if (!confirm("Are you sure you want
to navigate away from this page to " + next)) {
event.preventDefault();
}
});
vm.reloadData = function () {
$route.reload();
}
$http.get("StudentService.asmx/GetAllStudents")
.then(function (response) {
vm.students =
response.data;
})
})
The next and current parameters of $locationChangeStart event handler has the complete next and current URLs. So when you click on home while on students page, you will see the following message.

In our next video we will discuss the different events that are triggered when a route change occurs in an angular application.
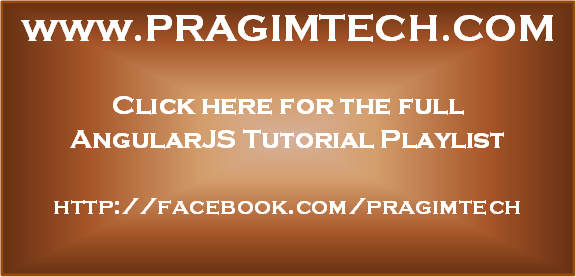
No comments:
Post a Comment
It would be great if you can help share these free resources