Suggested Videos
Part 36 - AngularJS route reload
Part 37 - Difference between $scope and $rootScope
Part 38 - AngularJS cancel route change
In this video we will discuss
1. Different events that are triggered when a route change occurs in an angular application
2. Logging the events and event handler parameters to inspect their respective properties
When route navigation occurs in an Angular application, the following events are triggered
1. $locationChangeStart
2. $routeChangeStart
3. $locationChangeSuccess
4. $routeChangeSuccess
The following code proves the above point
Please note that we are injecting $log service into the controller function to log the events.
In our previous video, we used $$route.originalPath property to get the route that the user is navigating to. How do we know next parameter has $$route.originalPath property. Well the easiest way is to log and inspect their properties. The following code does exactly the same thing.
In this example we have logged just $routeChangeStart & $locationChangeStart events parameters. In a similar way, you can also log $routeChangeSuccess & $locationChangeSuccess events parameters.
Now, launch the browser developer tools and you can see the properties available
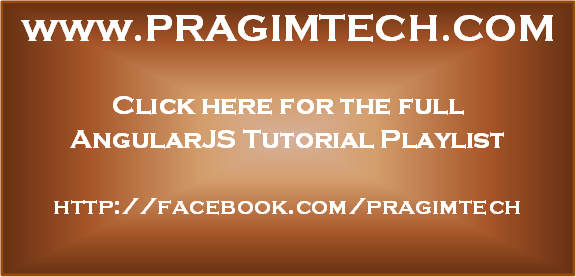
Part 36 - AngularJS route reload
Part 37 - Difference between $scope and $rootScope
Part 38 - AngularJS cancel route change
In this video we will discuss
1. Different events that are triggered when a route change occurs in an angular application
2. Logging the events and event handler parameters to inspect their respective properties
When route navigation occurs in an Angular application, the following events are triggered
1. $locationChangeStart
2. $routeChangeStart
3. $locationChangeSuccess
4. $routeChangeSuccess
The following code proves the above point
.controller("studentsController", function ($http, $route, $rootScope,
$log) {
var vm = this;
$rootScope.$on("$locationChangeStart", function () {
$log.debug("$locationChangeStart fired");
});
$rootScope.$on("$routeChangeStart", function () {
$log.debug("$routeChangeStart fired");
});
$rootScope.$on("$locationChangeSuccess", function () {
$log.debug("$locationChangeSuccess fired");
});
$rootScope.$on("$routeChangeSuccess", function () {
$log.debug("$routeChangeSuccess fired");
});
vm.reloadData = function () {
$route.reload();
}
$http.get("StudentService.asmx/GetAllStudents")
.then(function (response) {
vm.students = response.data;
})
})
Please note that we are injecting $log service into the controller function to log the events.
In our previous video, we used $$route.originalPath property to get the route that the user is navigating to. How do we know next parameter has $$route.originalPath property. Well the easiest way is to log and inspect their properties. The following code does exactly the same thing.
.controller("studentsController", function ($http, $route, $scope,
$log) {
var vm = this;
$scope.$on("$routeChangeStart", function (event, next, current) {
$log.debug("RouteChangeStart Event");
$log.debug(event);
$log.debug(next);
$log.debug(current);
});
$scope.$on("$locationChangeStart", function (event, next, current) {
$log.debug("LocationChangeStart Event");
$log.debug(event);
$log.debug(next);
$log.debug(current);
});
vm.reloadData = function () {
$route.reload();
}
$http.get("StudentService.asmx/GetAllStudents")
.then(function (response) {
vm.students = response.data;
})
})
In this example we have logged just $routeChangeStart & $locationChangeStart events parameters. In a similar way, you can also log $routeChangeSuccess & $locationChangeSuccess events parameters.
Now, launch the browser developer tools and you can see the properties available

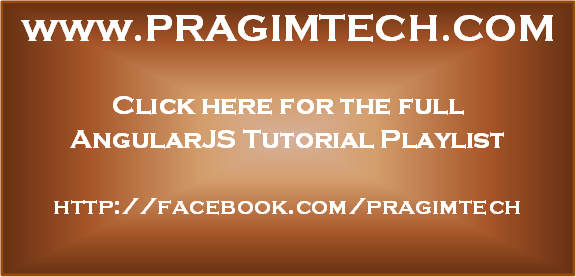
im new in wcf im facing error like that how to fixed
ReplyDeleteHTTP could not register URL http://+:8070/. Your process does not have access rights to this namespace (see http://go.microsoft.com/fwlink/?LinkId=70353 for details).
Why did we change $rootScope to $scope? Is it like the success events get triggered from different controllers?
ReplyDelete