Suggested Videos
Part 20 - Convert JSON string to .net object
Part 21 - jQuery DOM manipulation methods
Part 22 - jQuery wrap elements
In this video we will discuss how to append and prepend elements
To append elements we have
append()
appendTo()
To prepend elements we have
prepend()
prependTo()
Since these methods modify DOM, they belong to DOM manipulation category.
jquery append example : The following example appends the specified HTML to all div elements
Output :
jquery appendto example : The above example can be rewritten using appendTo as shown below.
Output :
What is the difference between append and appendTo
Both these methods perform the same task. The only difference is in the syntax. With append method we first specify the target elements and then the content that we want to append, where as we do the opposite with appendTo method.
jquery prepend example : The following example prepends the specified HTML to all div elements
Output :
jquery prependTo example : The above example can be rewritten using prependTo as shown below.
Output :
What is the difference between prepend and prependTo
Both these methods perform the same task. The only difference is in the syntax. With prepend method we first specify the target elements and then the content that we want to prepend, where as we do the opposite with prependTo method.
jQuery append existing element example : These methods (append, appendTo, prepend, prependTo) can also select an element on the page and insert it into another
Output :
Difference between prepend and append
prepend method, inserts the specified content to the beginning of each element in the set of matched elements, where as append method inserts the specified content to the end of each element in the set of matched elements.
Part 20 - Convert JSON string to .net object
Part 21 - jQuery DOM manipulation methods
Part 22 - jQuery wrap elements
In this video we will discuss how to append and prepend elements
To append elements we have
append()
appendTo()
To prepend elements we have
prepend()
prependTo()
Since these methods modify DOM, they belong to DOM manipulation category.
jquery append example : The following example appends the specified HTML to all div elements
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('div').append('<b>
Tutorial</b>');
});
</script>
</head>
<body style="font-family:Arial">
<div id="div1">jQuery</div>
<div id="div2">C#</div>
<div id="div3">ASP.NET</div>
</body>
</html>
Output :

jquery appendto example : The above example can be rewritten using appendTo as shown below.
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('<b>
Tutorial</b>').appendTo('div');
});
</script>
</head>
<body style="font-family:Arial">
<div id="div1">jQuery</div>
<div id="div2">C#</div>
<div id="div3">ASP.NET</div>
</body>
</html>
Output :
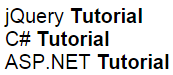
What is the difference between append and appendTo
Both these methods perform the same task. The only difference is in the syntax. With append method we first specify the target elements and then the content that we want to append, where as we do the opposite with appendTo method.
jquery prepend example : The following example prepends the specified HTML to all div elements
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('div').prepend('<b>Tutorial
</b>');
});
</script>
</head>
<body style="font-family:Arial">
<div id="div1">jQuery</div>
<div id="div2">C#</div>
<div id="div3">ASP.NET</div>
</body>
</html>
Output :

jquery prependTo example : The above example can be rewritten using prependTo as shown below.
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('<b>Tutorial
</b>').prependTo('div');
});
</script>
</head>
<body style="font-family:Arial">
<div id="div1">jQuery</div>
<div id="div2">C#</div>
<div id="div3">ASP.NET</div>
</body>
</html>
Output :

What is the difference between prepend and prependTo
Both these methods perform the same task. The only difference is in the syntax. With prepend method we first specify the target elements and then the content that we want to prepend, where as we do the opposite with prependTo method.
jQuery append existing element example : These methods (append, appendTo, prepend, prependTo) can also select an element on the page and insert it into another
<html>
<head>
<title></title>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('div').append($('#mySpan'));
});
</script>
</head>
<body style="font-family:Arial">
<span id="mySpan"> Programming</span>
<div id="div1">jQuery</div>
<div id="div2">C#</div>
<div id="div3">ASP.NET</div>
</body>
</html>
Output :
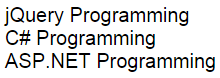
Difference between prepend and append
prepend method, inserts the specified content to the beginning of each element in the set of matched elements, where as append method inserts the specified content to the end of each element in the set of matched elements.
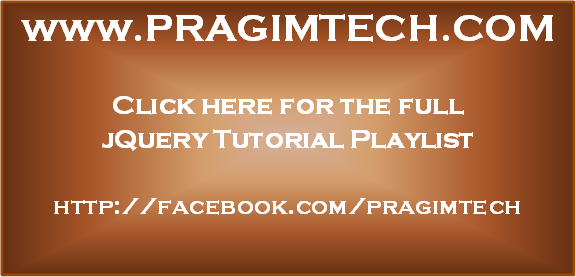
No comments:
Post a Comment
It would be great if you can help share these free resources