Suggested Videos
Part 19 - Convert JSON object to string
Part 20 - Convert JSON string to .net object
Part 21 - jQuery DOM manipulation methods
In this video we will discuss how to wrap and unwrap elements
The following jquery methods are used to wrap and unwrap elements. Since these methods modify DOM, they belong to DOM manipulation category.
wrap
unwrap
wrapAll
wrapInner
wrap - Wrap an HTML structure around each element in the set of matched elements.
Consider the following HTML
The following line of code wraps each of the above div element with another div element.
So the HTML in the DOM would now look as shown below. To view the DOM HTML use the browser developer tools.
Example :
Output :
unwrap - Remove the parents of the set of matched elements from the DOM.
Example :
wrapAll - Wrap an HTML structure around all elements in the set of matched elements.
Consider the following HTML
The following line of code wraps all of the matching div element with another div element.
So the HTML in the DOM would now look as shown below.
Example :
wrapInner - Wrap an HTML structure around the content of each element in the set of matched elements.
Consider the following HTML
The following line of code wraps each of the above div element content with another div element.
So the HTML in the DOM would now look as shown below.
Example :
Part 19 - Convert JSON object to string
Part 20 - Convert JSON string to .net object
Part 21 - jQuery DOM manipulation methods
In this video we will discuss how to wrap and unwrap elements
The following jquery methods are used to wrap and unwrap elements. Since these methods modify DOM, they belong to DOM manipulation category.
wrap
unwrap
wrapAll
wrapInner
wrap - Wrap an HTML structure around each element in the set of matched elements.
Consider the following HTML
<div id="div1">
DIV 1
</div>
<div id="div2">
DIV 2
</div>
<div id="div3">
DIV 3
</div>
The following line of code wraps each of the above div element with another div element.
$('div').wrap('<div
class="containerDiv"></div>');
So the HTML in the DOM would now look as shown below. To view the DOM HTML use the browser developer tools.
<div class="containerDiv">
<div id="div1">
DIV 1
</div>
</div>
<div class="containerDiv">
<div id="div2">
DIV 2
</div>
</div>
<div class="containerDiv">
<div id="div3">
DIV 3
</div>
</div>
Example :
<html>
<head>
<title></title>
<style>
.containerDiv {
background-color: red;
color: white;
font-weight: bold;
margin: 5px;
}
</style>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
alert($('div.containerDiv').length);
$('div').wrap('<div
class="containerDiv"></div>');
alert($('div.containerDiv').length);
});
</script>
</head>
<body style="font-family:Arial">
<div id="div1">
DIV 1
</div>
<div id="div2">
DIV 2
</div>
<div id="div3">
DIV 3
</div>
</body>
</html>
Output :
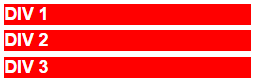
unwrap - Remove the parents of the set of matched elements from the DOM.
Example :
<html>
<head>
<title></title>
<style>
.containerDiv {
background-color: red;
color: white;
font-weight: bold;
margin: 5px;
}
</style>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
alert($('div.containerDiv').length);
$('div').wrap('<div
class="containerDiv"></div>');
alert($('div.containerDiv').length);
$('div').unwrap();
alert($('div.containerDiv').length);
});
</script>
</head>
<body style="font-family:Arial">
<div id="div1">
DIV 1
</div>
<div id="div2">
DIV 2
</div>
<div id="div3">
DIV 3
</div>
</body>
</html>
wrapAll - Wrap an HTML structure around all elements in the set of matched elements.
Consider the following HTML
<div id="div1">
DIV 1
</div>
<div id="div2">
DIV 2
</div>
<div id="div3">
DIV 3
</div>
The following line of code wraps all of the matching div element with another div element.
$('div').wrapAll('<div
class="containerDiv"></div>');
So the HTML in the DOM would now look as shown below.
<div class="containerDiv">
<div id="div1">
DIV 1
</div><div id="div2">
DIV 2
</div><div id="div3">
DIV 3
</div>
</div>
Example :
<html>
<head>
<title></title>
<style>
.containerDiv {
background-color: red;
color: white;
font-weight: bold;
margin: 5px;
}
</style>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
alert($('div.containerDiv').length);
$('div').wrapAll('<div
class="containerDiv"></div>');
alert($('div.containerDiv').length);
$('div').unwrap();
alert($('div.containerDiv').length);
});
</script>
</head>
<body style="font-family:Arial">
<div id="div1">
DIV 1
</div>
<div id="div2">
DIV 2
</div>
<div id="div3">
DIV 3
</div>
</body>
</html>
wrapInner - Wrap an HTML structure around the content of each element in the set of matched elements.
Consider the following HTML
<div id="div1">
DIV 1
</div>
<div id="div2">
DIV 2
</div>
<div id="div3">
DIV 3
</div>
The following line of code wraps each of the above div element content with another div element.
$('div').wrapInner('<div
class="containerDiv"></div>');
So the HTML in the DOM would now look as shown below.
<div id="div1">
<div class="containerDiv">
DIV 1
</div>
</div>
<div id="div2">
<div class="containerDiv">
DIV 2
</div>
</div>
<div id="div3">
<div class="containerDiv">
DIV 3
</div>
</div>
Example :
<html>
<head>
<title></title>
<style>
.containerDiv {
background-color: red;
color: white;
font-weight: bold;
margin: 5px;
}
</style>
<script src="jquery-1.11.2.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('div').wrapInner('<div
class="containerDiv"></div>');
});
</script>
</head>
<body style="font-family:Arial">
<div id="div1">
DIV 1
</div>
<div id="div2">
DIV 2
</div>
<div id="div3">
DIV 3
</div>
</body>
</html>
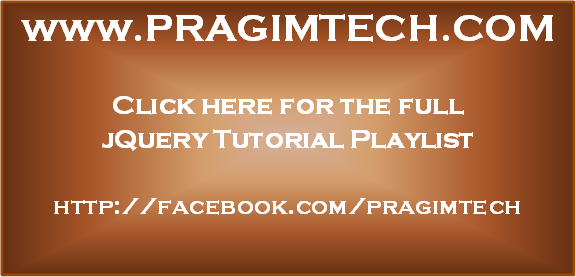
No comments:
Post a Comment
It would be great if you can help share these free resources