Suggested Videos
Part 17 - jQuery method chaining
Part 18 - What is JSON
Part 19 - Convert JSON object to string
In this video we will discuss
1. How to convert .NET object to JSON string
2. How to convert a JSON string to .NET object
We will be using the following Employee class
The following example converts List<Employee> objects to a JSON string. Serialize() method of JavaScriptSerializer class converts a .NET object to a JSON string. JavaScriptSerializer class is present in System.Web.Script.Serialization namespace.
Output :
[{"firstName":"Todd","lastName":"Grover","gender":"Male","salary":50000},{"firstName":"Sara","lastName":"Baker","gender":"Female","salary":40000}]
The following example converts a JSON string to List<Employee> objects. Deserialize() method of JavaScriptSerializer class converts a JSON string to a .NET object.
Output :
Part 17 - jQuery method chaining
Part 18 - What is JSON
Part 19 - Convert JSON object to string
In this video we will discuss
1. How to convert .NET object to JSON string
2. How to convert a JSON string to .NET object
We will be using the following Employee class
public class Employee
{
public string firstName { get; set; }
public string lastName { get; set; }
public string gender { get; set; }
public int salary { get; set; }
}
The following example converts List<Employee> objects to a JSON string. Serialize() method of JavaScriptSerializer class converts a .NET object to a JSON string. JavaScriptSerializer class is present in System.Web.Script.Serialization namespace.
using System;
using System.Collections.Generic;
using System.Web.Script.Serialization;
namespace Demo
{
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
Employee employee1 = new Employee
{
firstName = "Todd",
lastName = "Grover",
gender = "Male",
salary = 50000
};
Employee employee2 = new Employee
{
firstName = "Sara",
lastName = "Baker",
gender = "Female",
salary = 40000
};
List<Employee> listEmployee = new List<Employee>();
listEmployee.Add(employee1);
listEmployee.Add(employee2);
JavaScriptSerializer javaScriptSerializer = new JavaScriptSerializer();
string JSONString =
javaScriptSerializer.Serialize(listEmployee);
Response.Write(JSONString);
}
}
}
Output :
[{"firstName":"Todd","lastName":"Grover","gender":"Male","salary":50000},{"firstName":"Sara","lastName":"Baker","gender":"Female","salary":40000}]
The following example converts a JSON string to List<Employee> objects. Deserialize() method of JavaScriptSerializer class converts a JSON string to a .NET object.
string jsonString = "[{\"firstName\":\"Todd\",\"lastName\":\"Grover\",\"gender\":\"Male\",\"salary\":50000},{\"firstName\":\"Sara\",\"lastName\":\"Baker\",\"gender\":\"Female\",\"salary\":40000}]";
JavaScriptSerializer javaScriptSerializer = new JavaScriptSerializer();
List<Employee> employees = (List<Employee>)javaScriptSerializer.Deserialize(jsonString, typeof(List<Employee>));
foreach (Employee employee in employees)
{
Response.Write("First Name = "
+ employee.firstName + "<br/>");
Response.Write("Last Name = "
+ employee.lastName + "<br/>");
Response.Write("Gender = " +
employee.gender + "<br/>");
Response.Write("Salary = " +
employee.salary + "<br/><br/>");
}
Output :

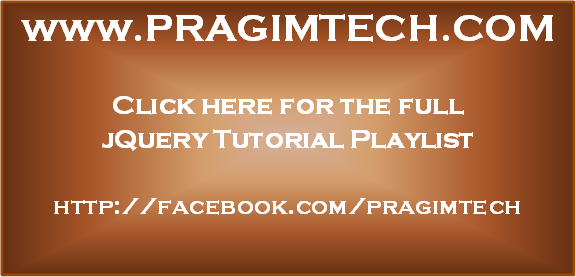
Well sir, Thankx for amazing tutorials. I found error in thi line:
ReplyDeleteList employees = (List)javaScriptSerializer.Deserialize(jsonString, typeof(List));
It says that No overload for method 'Deserialize' takes '2' arguments
Please check.
Rajeev Ranjan
this is the right code----- List=(List)javaScriptSerializer.Deserialize(jsonString, typeof(List));
Deletefirst view correctly the ask use List = (List)javaScriptSerializer.Deserialize(jsonString, typeof(List));
ReplyDelete