Suggested Videos
Part 64 - Polymorphism in JavaScript
Part 65 - Object reflection in JavaScript
Part 66 - Strict Mode in JavaScript
In this video we will discuss
1. What are cookies and why are they needed
2. How to write a cookie and read it later
Why are cookies needed
Web applications work on HTTP protocol which is a stateless protocol. This means after the web server has processed the client request for a web page, the web server will not remember anything about the client that made the request. Let us understand what this statement means with an example.
1. Design a web page with a DropDownList as show below.
2. When the user selects a color from the DropDownList we want to change the background color of the page to the selected color.
3. We want the web application to remember the user's preferred color. On a subsequent visit to the same page it should display the page with the background color that we previously selected.
4. Now close the browser. Open a new instance of the browser and navigate to the same page. Notice that, the page does not remember the color we selected earlier. This is because web applications work on HTTP protocol which is a stateless protocol. This means after the web server has processed the initial client request, it does not remember aynthing about the settings the client made. There are several ways to make a web application remember these settings. One of the easiest and common ways is by using cookies.
What are cookies
Cookies are small text files that a browser stores in the visitor's computer. A cookie is basically a string of name-value pairs separated by semi-colons.
How does a cookie look
color=red;expires=Fri, 5 Aug 2016 01:00:00 UTC;
In the cookie string above we have 2 name-value pairs.
The expires attribute specifies when the cookie is going to expire. By default cookies are deleted when the current browser session ends. If you want to store the cookie on the client computer even after the browser session has ended then specify either expires or max-age attributes. The color attribute is set to "red".
How to write a cookie : Use the document object's cookie property to write the cookie.
document.cookie = "color=red;expires=Fri, 5 Aug 2016 01:00:00 UTC;"
How to read a cookie : Use the document object's cookie property to read the cookie.
var cookieString = document.cookie;
Here is the complete example which remembers the user color preference
Part 64 - Polymorphism in JavaScript
Part 65 - Object reflection in JavaScript
Part 66 - Strict Mode in JavaScript
In this video we will discuss
1. What are cookies and why are they needed
2. How to write a cookie and read it later
Why are cookies needed
Web applications work on HTTP protocol which is a stateless protocol. This means after the web server has processed the client request for a web page, the web server will not remember anything about the client that made the request. Let us understand what this statement means with an example.
1. Design a web page with a DropDownList as show below.

2. When the user selects a color from the DropDownList we want to change the background color of the page to the selected color.

3. We want the web application to remember the user's preferred color. On a subsequent visit to the same page it should display the page with the background color that we previously selected.
4. Now close the browser. Open a new instance of the browser and navigate to the same page. Notice that, the page does not remember the color we selected earlier. This is because web applications work on HTTP protocol which is a stateless protocol. This means after the web server has processed the initial client request, it does not remember aynthing about the settings the client made. There are several ways to make a web application remember these settings. One of the easiest and common ways is by using cookies.
What are cookies
Cookies are small text files that a browser stores in the visitor's computer. A cookie is basically a string of name-value pairs separated by semi-colons.
How does a cookie look
color=red;expires=Fri, 5 Aug 2016 01:00:00 UTC;
In the cookie string above we have 2 name-value pairs.
The expires attribute specifies when the cookie is going to expire. By default cookies are deleted when the current browser session ends. If you want to store the cookie on the client computer even after the browser session has ended then specify either expires or max-age attributes. The color attribute is set to "red".
How to write a cookie : Use the document object's cookie property to write the cookie.
document.cookie = "color=red;expires=Fri, 5 Aug 2016 01:00:00 UTC;"
How to read a cookie : Use the document object's cookie property to read the cookie.
var cookieString = document.cookie;
Here is the complete example which remembers the user color preference
<select id="ddlTheme" onchange="setColorCookie()">
<option value="Select Color">Select Color</option>
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
<script type="text/javascript">
window.onload = function ()
{
if (document.cookie.length != 0)
{
var nameValueArray = document.cookie.split("=");
document.getElementById("ddlTheme").value = nameValueArray[1];
document.bgColor = nameValueArray[1];
}
}
function setColorCookie()
{
var selectedValue = document.getElementById("ddlTheme").value;
if (selectedValue != "Select
Color")
{
document.bgColor = selectedValue;
document.cookie = "color=" + selectedValue
+
";expires=Fri,
5 Aug 2016 01:00:00 UTC;";
}
}
</script>
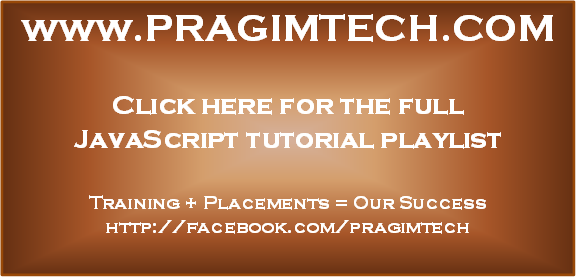
Dear Mr. Kuvenkat,
ReplyDeleteThank you very much for posting videos. I learned quite a lot from you than any other website or tutorial. Can you please post a video on dependency injection and inversion of control? Thank you, Sir.
I have a question:
ReplyDeleteWhy does the document.cookie only get 1 namevaluepair?