Suggested Videos
Part 62 - Inheritance in JavaScript
Part 63 - Abstract classes in JavaScript
Part 64 - Polymorphism in JavaScript
In this video we will discuss, object reflection in JavaScript with an example.
Object oriented programming languages like C# and Java, support reflection. Reflection allows us to inspect meta data of assemblies, modules and types. Since JavaScript is also an object oriented programming language, it also supports the concept of reflection. Let's understand Object reflection in JavaScript with an example.
Employee object in the following example has 4 public properties
1. firstName
2. lastName
3. email
4. gender
and 3 public functions
1. getFullName
2. getEmail
3. getGender
The following code retrieves all the public properties and methods of the Employee object.
for (var property in employee)
{
document.write(property + "<br/>");
}
Output :
firstName
lastName
gender
email
getFullName
getEmail
getGender
The following code retrieves all the public properties & functions of the Employee object along with their values.
for (var property in employee)
{
document.write(property + " : " + employee[property] + "<br/>");
}
Output :
firstName : Mark
lastName : Matt
gender : Male
email : a@a.com
getFullName : function () { return this.firstName + " " + this.lastName; }
getEmail : function () { return this.email; }
getGender : function () { return this.gender; }
The following code retrieves only the public properties of the Employee object.
for (var property in employee)
{
if (typeof employee[property] != "function")
{
document.write(property + " : " + employee[property] + "<br/>");
}
}
Output:
firstName : Mark
lastName : Matt
gender : Male
email : a@a.com
The following code retrieves only the public functions of the Employee object.
for (var property in employee)
{
if (typeof employee[property] == "function")
{
document.write(property + " : " + employee[property] + "<br/>");
}
}
Output :
getFullName : function () { return this.firstName + " " + this.lastName; }
getEmail : function () { return this.email; }
getGender : function () { return this.gender; }
When 2 objects are related thru inheritance, the child object will have access to parent object methods and properties. In this example, PermanentEmployee is a child of Employee. The following code shows all the properties and methods of the PermanentEmployee object including those it inherited from the Employee object.
var employee = new Employee("Mark", "Matt", "Male", "a@a.com");
var PermanentEmployee = function (annualSalry)
{
this.annualSalary = annualSalry;
}
PermanentEmployee.prototype = employee;
var pe = new PermanentEmployee(50000);
for (var property in pe)
{
document.write(property + " : " + pe[property] + "<br/>");
}
Output :
annualSalary : 50000
firstName : Mark
lastName : Matt
gender : Male
email : a@a.com
getFullName : function () { return this.firstName + " " + this.lastName; }
getEmail : function () { return this.email; }
getGender : function () { return this.gender; }
In this example, we are using hasOwnProperty() method to determine if a property is defined on the actual object or it's prototype. This method returns true if the property is defined by the object itself, otherwise false. The following code retrieves only the public members that are defined in the PermanentEmployee object. The members inherited from the base Employee object are excluded.
for (var property in pe)
{
if (pe.hasOwnProperty(property))
{
document.write(property + " : " + pe[property] + "<br/>");
}
}
Output : annualSalary : 50000
The following code retrieves only the public members inherited from the parent object. Public members defined in PermanentEmployee object are excluded.
for (var property in pe)
{
if (!pe.hasOwnProperty(property))
{
document.write(property + " : " + pe[property] + "<br/>");
}
}
Output :
firstName : Mark
lastName : Matt
gender : Male
email : a@a.com
getFullName : function () { return this.firstName + " " + this.lastName; }
getEmail : function () { return this.email; }
getGender : function () { return this.gender; }
Part 62 - Inheritance in JavaScript
Part 63 - Abstract classes in JavaScript
Part 64 - Polymorphism in JavaScript
In this video we will discuss, object reflection in JavaScript with an example.
Object oriented programming languages like C# and Java, support reflection. Reflection allows us to inspect meta data of assemblies, modules and types. Since JavaScript is also an object oriented programming language, it also supports the concept of reflection. Let's understand Object reflection in JavaScript with an example.
Employee object in the following example has 4 public properties
1. firstName
2. lastName
3. email
4. gender
and 3 public functions
1. getFullName
2. getEmail
3. getGender
var Employee = function
(firstName, lastName, gender, email)
{
this.firstName = firstName;
this.lastName = lastName;
this.gender = gender;
this.email = email;
}
Employee.prototype.getFullName =
function ()
{
return this.firstName
+ " " + this.lastName;
}
Employee.prototype.getEmail =
function ()
{
return this.email;
}
Employee.prototype.getGender =
function ()
{
return this.gender;
}
var employee = new
Employee("Mark", "Matt", "Male",
"a@a.com");
The following code retrieves all the public properties and methods of the Employee object.
for (var property in employee)
{
document.write(property + "<br/>");
}
Output :
firstName
lastName
gender
getFullName
getEmail
getGender
The following code retrieves all the public properties & functions of the Employee object along with their values.
for (var property in employee)
{
document.write(property + " : " + employee[property] + "<br/>");
}
Output :
firstName : Mark
lastName : Matt
gender : Male
email : a@a.com
getFullName : function () { return this.firstName + " " + this.lastName; }
getEmail : function () { return this.email; }
getGender : function () { return this.gender; }
The following code retrieves only the public properties of the Employee object.
for (var property in employee)
{
if (typeof employee[property] != "function")
{
document.write(property + " : " + employee[property] + "<br/>");
}
}
Output:
firstName : Mark
lastName : Matt
gender : Male
email : a@a.com
The following code retrieves only the public functions of the Employee object.
for (var property in employee)
{
if (typeof employee[property] == "function")
{
document.write(property + " : " + employee[property] + "<br/>");
}
}
Output :
getFullName : function () { return this.firstName + " " + this.lastName; }
getEmail : function () { return this.email; }
getGender : function () { return this.gender; }
When 2 objects are related thru inheritance, the child object will have access to parent object methods and properties. In this example, PermanentEmployee is a child of Employee. The following code shows all the properties and methods of the PermanentEmployee object including those it inherited from the Employee object.
var employee = new Employee("Mark", "Matt", "Male", "a@a.com");
var PermanentEmployee = function (annualSalry)
{
this.annualSalary = annualSalry;
}
PermanentEmployee.prototype = employee;
var pe = new PermanentEmployee(50000);
for (var property in pe)
{
document.write(property + " : " + pe[property] + "<br/>");
}
Output :
annualSalary : 50000
firstName : Mark
lastName : Matt
gender : Male
email : a@a.com
getFullName : function () { return this.firstName + " " + this.lastName; }
getEmail : function () { return this.email; }
getGender : function () { return this.gender; }
In this example, we are using hasOwnProperty() method to determine if a property is defined on the actual object or it's prototype. This method returns true if the property is defined by the object itself, otherwise false. The following code retrieves only the public members that are defined in the PermanentEmployee object. The members inherited from the base Employee object are excluded.
for (var property in pe)
{
if (pe.hasOwnProperty(property))
{
document.write(property + " : " + pe[property] + "<br/>");
}
}
Output : annualSalary : 50000
The following code retrieves only the public members inherited from the parent object. Public members defined in PermanentEmployee object are excluded.
for (var property in pe)
{
if (!pe.hasOwnProperty(property))
{
document.write(property + " : " + pe[property] + "<br/>");
}
}
Output :
firstName : Mark
lastName : Matt
gender : Male
email : a@a.com
getFullName : function () { return this.firstName + " " + this.lastName; }
getEmail : function () { return this.email; }
getGender : function () { return this.gender; }
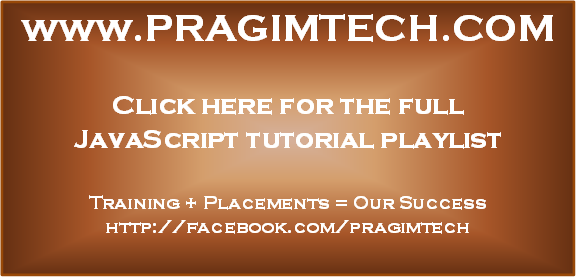
No comments:
Post a Comment
It would be great if you can help share these free resources