Suggested Videos
Part 61 - Overriding JavaScript functions
Part 62 - Inheritance in JavaScript
Part 63 - Abstract classes in JavaScript
In this video we will discuss, how to implement polymorphism in JavaScript with an example.
Object oriented programming languages like C# and Java, support polymorphism. Here is a C# example.
Output :
I am a generic shape
I am a circle
I am a square
I am a generic shape
Since JavaScript is also an object oriented programming language, it also supports the concept of polymorphism. Here is an example.
Ouptut:
I am a generic shape
I am a circle
I am a square
I am a generic shape
Part 61 - Overriding JavaScript functions
Part 62 - Inheritance in JavaScript
Part 63 - Abstract classes in JavaScript
In this video we will discuss, how to implement polymorphism in JavaScript with an example.
Object oriented programming languages like C# and Java, support polymorphism. Here is a C# example.
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender,
EventArgs e)
{
Shape[] shapes = new Shape[]
{
new Shape(), new Circle(),
new Square(), new Triangle()
};
foreach (Shape shape in shapes)
{
Response.Write(shape.draw() + "<br/>");
}
}
}
public class Shape
{
public virtual string
draw()
{
return "I am a generic shape";
}
}
public class Circle : Shape
{
public override string draw()
{
return "I am a circle";
}
}
public class Square : Shape
{
public override string draw()
{
return "I am a square";
}
}
public class Triangle : Shape
{ }
Output :
I am a generic shape
I am a circle
I am a square
I am a generic shape
Since JavaScript is also an object oriented programming language, it also supports the concept of polymorphism. Here is an example.
<script type="text/javascript">
// Shape object is be the base object
var Shape = function ()
{ }
// Add draw function to the Shape
prototype
// Objects derived from Shape should be
able to override draw() method
Shape.prototype.draw = function ()
{
return "I am a generic shape";
}
// Create a Circle object
var Circle = function ()
{ }
// Make shape the parent for Circle
Circle.prototype = Object.create(Shape.prototype);
// Circle object overrides draw() method
Circle.prototype.draw = function ()
{
return "I am a circle";
}
var Square = function ()
{ }
Square.prototype = Object.create(Shape.prototype);
Square.prototype.draw = function ()
{
return "I am a square";
}
var Triangle = function
() { }
Triangle.prototype = Object.create(Shape.prototype);
var shapes = [new Shape(),
new Circle(), new Square(), new Triangle()];
shapes.forEach(function
(shape)
{
document.write(shape.draw() +
"<br/>")
});
</script>
Ouptut:
I am a generic shape
I am a circle
I am a square
I am a generic shape
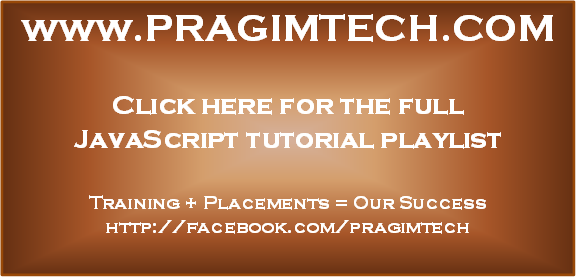
object does not support this property error occurred at Circle.prototype = Object.create(Shape.prototype);
ReplyDeleteI had the same issue and same message. It turns out the issue is not with coding but with settings of IE. All I had to do was reset IE from Tools => internet options => advanced => reset (including personal settings) That did it for me. Let me know if it works.
Delete