Suggested Videos
Part 15 - Ternary operator in JavaScript
Part 16 - Loops in JavaScript
Part 17 - do while loop in JavaScript
In this video we will discuss for loop in JavaScript with an example.
To understand for loop, let us print even numbers starting from ZERO till a number provided by the user at runtime.
First, let us do this using a while loop. The following while loop example prints all even numbers starting from ZERO till a number provided by the user at runtime. For example if the end user provides 10 at runtime, even numbers starting from ZERO till 10 are printed.
With while loop
1. The initialization of the variable is done at one place
2. Boolean condition is checked at another place
3. The variable participating in the boolean expression is updated at another place
For loop example : With for loop all three can be done in one place. To make this clear, look at the syntax of the for loop below.
for(initialization; boolean Condition; update variable)
{
statements;
}
Now, let us rewrite the above example using a for loop, instead of while loop
Notice that all the 3 things, i.e
1. Variable initialization
2. Boolean condition check and
3. Updating the variable participating in the boolean expression are all done at one place in the for loop. All these 3 things are optional in a for loop.
Example : Variable initialization is optional in a for loop. Notice that we have moved variable intialization (var start = 0;) outside of the for loop head.
Example : Just like variable initialization, condition check is also optional in a for loop. Notice that we have moved condition check (start > targetNumber) from the head of the for loop, into it's body and we used a break statement to end the loop, otherwise this could become an infinite for loop.
Example : In this example we have moved the expression that updates the variable participating in the boolean expression (start = start + 2) from the head of the for loop, into it's body.
Part 15 - Ternary operator in JavaScript
Part 16 - Loops in JavaScript
Part 17 - do while loop in JavaScript
In this video we will discuss for loop in JavaScript with an example.
To understand for loop, let us print even numbers starting from ZERO till a number provided by the user at runtime.
First, let us do this using a while loop. The following while loop example prints all even numbers starting from ZERO till a number provided by the user at runtime. For example if the end user provides 10 at runtime, even numbers starting from ZERO till 10 are printed.
var targetNumber = Number(prompt("Please enter your target number", ""));
var start = 0;
while (start <= targetNumber)
{
document.write(start + "<br/>");
start = start + 2;
}
With while loop
1. The initialization of the variable is done at one place
2. Boolean condition is checked at another place
3. The variable participating in the boolean expression is updated at another place
For loop example : With for loop all three can be done in one place. To make this clear, look at the syntax of the for loop below.
for(initialization; boolean Condition; update variable)
{
statements;
}
Now, let us rewrite the above example using a for loop, instead of while loop
var targetNumber = Number(prompt("Please enter your target number", ""));
for (var start = 0; start <= targetNumber;
start = start + 2)
{
document.write(start + "<br/>");
}
Notice that all the 3 things, i.e
1. Variable initialization
2. Boolean condition check and
3. Updating the variable participating in the boolean expression are all done at one place in the for loop. All these 3 things are optional in a for loop.
Example : Variable initialization is optional in a for loop. Notice that we have moved variable intialization (var start = 0;) outside of the for loop head.
var targetNumber = Number(prompt("Please enter your target number", ""));
var start = 0;
for (; start <= targetNumber; start =
start + 2)
{
document.write(start + "<br/>");
}
Example : Just like variable initialization, condition check is also optional in a for loop. Notice that we have moved condition check (start > targetNumber) from the head of the for loop, into it's body and we used a break statement to end the loop, otherwise this could become an infinite for loop.
var targetNumber = Number(prompt("Please enter your target number", ""));
var start = 0
for (; ; start = start + 2)
{
if (start > targetNumber)
{
break;
}
document.write(start + "<br/>");
}
Example : In this example we have moved the expression that updates the variable participating in the boolean expression (start = start + 2) from the head of the for loop, into it's body.
var targetNumber = Number(prompt("Please enter your target number", ""));
var start = 0
for (; ; )
{
if (start > targetNumber)
{
break;
}
document.write(start + "<br/>");
start = start + 2;
}
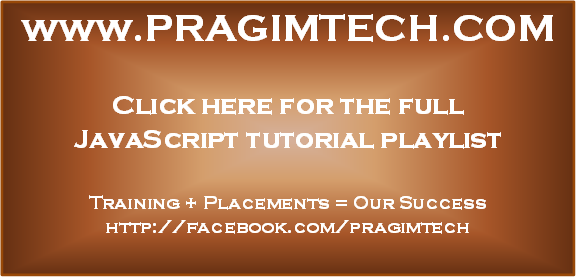
No comments:
Post a Comment
It would be great if you can help share these free resources