Suggested Videos
Part 58 - Export gridview to pdf
Part 59 - Generate pdf document from gridview data and store on web server
Part 60 - Repeater control in asp.net
In this video we will discuss, how to get value from a control that is present inside an itemtemplate of a gridview templatefield column.
We will be using the following 2 tables in this demo
We want to retrieve products from Products table and display them in a GridView control as shown below. The ID field in the GridView control should be a TemplateField. Inside the Template field we should have a LinkButton, this should display the Product ID and it must be clickable.
Upon clicking on the Product ID, the user should be redirected to product details page and this page should display all the details of the selected product as shown below.
Step 1 : Create Products and ProductDetails tables
Step 2 : Create stored procedures. The first stored procedure gets all the products from Products table. The second stored procedure gets the details of a specific product by ID by joining Products and ProductDetails tables.
Step 3 : Create a new empty asp.net web application project. Name it Demo. Add a connection string in web.config file to your database.
Step 4 : Add a webform to the project. Namt it products.aspx. Copy and paste the following HTML in the aspx page.
Step 5 : Copy and paste the following code in products.aspx.cs
Step 6 : Add another web form to the project. Name it ProductDetails.aspx. Copy and paste the following HTML in the aspx page.
Step 7 : Copy and paste the following code in ProductDetails.aspx.cs
Part 58 - Export gridview to pdf
Part 59 - Generate pdf document from gridview data and store on web server
Part 60 - Repeater control in asp.net
In this video we will discuss, how to get value from a control that is present inside an itemtemplate of a gridview templatefield column.
We will be using the following 2 tables in this demo
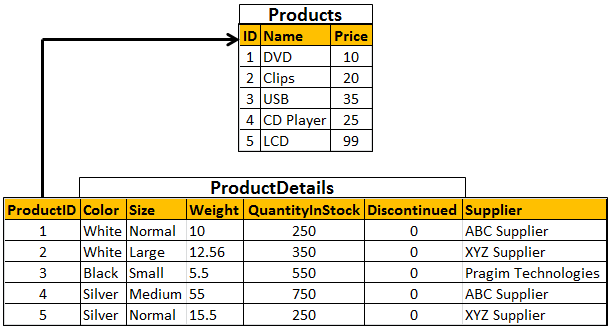
We want to retrieve products from Products table and display them in a GridView control as shown below. The ID field in the GridView control should be a TemplateField. Inside the Template field we should have a LinkButton, this should display the Product ID and it must be clickable.

Upon clicking on the Product ID, the user should be redirected to product details page and this page should display all the details of the selected product as shown below.
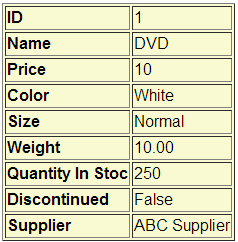
Step 1 : Create Products and ProductDetails tables
Create table Products
(
ID int primary
key,
Name nvarchar(50),
Price int
)
GO
Create table ProductDetails
(
ProductID int foreign key references Products(ID),
Color nvarchar(20),
Size nvarchar(10),
Weight decimal(8,2),
QuantityInStock int,
Discontinued bit,
Supplier nvarchar(20)
)
GO
Insert into Products values (1, 'DVD', 10)
Insert into Products values (2, 'Clips', 20)
Insert into Products values (3, 'USB', 35)
Insert into Products values (4, 'CD Player', 25)
Insert into Products values (5, 'LCD', 99)
GO
Insert into ProductDetails values (1, 'White', 'Normal', 10.00, 250, 0, 'ABC Supplier')
Insert into ProductDetails values (2, 'White', 'Large', 12.56, 350, 0, 'XYZ Supplier')
Insert into ProductDetails values (3, 'Black', 'Small', 5.5, 550, 0, 'Pragim Technologies')
Insert into ProductDetails values (4, 'Silver', 'Medium', 55.00, 750, 0, 'ABC Supplier')
Insert into ProductDetails values (5, 'Silver', 'Normal', 15.5, 250, 0, 'XYZ Supplier')
Create proc spGetBasicProductDetails
as
Begin
Select ID, Name, Price from Products
End
Create proc spGetProductDetailsByID
@ID int
as
Begin
Select ID, Name, Price, Color, Size, Weight,
QuantityInStock, Discontinued,
Supplier
from Products
join ProductDetails on Products.ID = ProductDetails.ProductID
where Products.ID = @ID
End
Step 3 : Create a new empty asp.net web application project. Name it Demo. Add a connection string in web.config file to your database.
<connectionStrings>
<add name="CS"
connectionString="server=.;database=Sample;integrated
security=true"/>
</connectionStrings>
Step 4 : Add a webform to the project. Namt it products.aspx. Copy and paste the following HTML in the aspx page.
<div style="font-family:Arial">
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False">
<Columns>
<asp:TemplateField HeaderText="ID">
<ItemTemplate>
<asp:LinkButton ID="LinkButton1" OnClick="LinkButton1_Click"
Text='<%#Eval("ID")
%>'
runat="server"></asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Price" HeaderText="Price" />
</Columns>
</asp:GridView>
</div>
Step 5 : Copy and paste the following code in products.aspx.cs
using System;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Web.UI.WebControls;
namespace Demo
{
public partial class
Products : System.Web.UI.Page
{
protected void Page_Load(object sender,
EventArgs e)
{
if (!IsPostBack)
{
string cs = ConfigurationManager.ConnectionStrings["CS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spGetBasicProductDetails", con);
cmd.CommandType = CommandType.StoredProcedure;
con.Open();
GridView1.DataSource = cmd.ExecuteReader();
GridView1.DataBind();
}
}
}
protected void LinkButton1_Click(object sender,
EventArgs e)
{
Response.Redirect("~/ProductDetails.aspx?"
+ "ID=" + ((LinkButton)sender).Text);
}
}
}
Step 6 : Add another web form to the project. Name it ProductDetails.aspx. Copy and paste the following HTML in the aspx page.
<div style="font-family: Arial">
<table border="1" style="background: LightGoldenrodYellow">
<tr>
<td>
<b>ID</b>
</td>
<td>
<asp:Label ID="lblID" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Name</b>
</td>
<td>
<asp:Label ID="lblName" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Price</b>
</td>
<td>
<asp:Label ID="lblPrice" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Color</b>
</td>
<td>
<asp:Label ID="lblColor" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Size</b>
</td>
<td>
<asp:Label ID="lblSize" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Weight</b>
</td>
<td>
<asp:Label ID="lblWeight" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Quantity In Stoc</b>
</td>
<td>
<asp:Label ID="lblQuantityInStock" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Discontinued</b>
</td>
<td>
<asp:Label ID="lblDiscontinued" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Supplier</b>
</td>
<td>
<asp:Label ID="lblSupplier" runat="server"></asp:Label>
</td>
</tr>
</table>
</div>
Step 7 : Copy and paste the following code in ProductDetails.aspx.cs
using System;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
namespace Demo
{
public partial class
ProductDetails : System.Web.UI.Page
{
protected void Page_Load(object sender,
EventArgs e)
{
if (!IsPostBack)
{
string productId = Request.QueryString["ID"];
if (productId == null)
{
Response.Redirect("Products.aspx");
}
string cs = ConfigurationManager.ConnectionStrings["CS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("spGetProductDetailsByID", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter parameter = new SqlParameter("@ID", productId);
cmd.Parameters.Add(parameter);
con.Open();
using (SqlDataReader rdr = cmd.ExecuteReader())
{
while (rdr.Read())
{
lblID.Text = rdr["ID"].ToString();
lblName.Text = rdr["Name"].ToString();
lblPrice.Text = rdr["Price"].ToString();
lblColor.Text = rdr["Color"].ToString();
lblSize.Text = rdr["Size"].ToString();
lblWeight.Text = rdr["Weight"].ToString();
lblQuantityInStock.Text =
rdr["QuantityInStock"].ToString();
lblDiscontinued.Text = rdr["Discontinued"].ToString();
lblSupplier.Text = rdr["Supplier"].ToString();
}
}
}
}
}
}
}
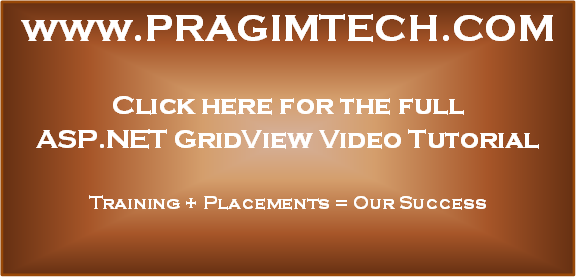
Would it be possible to keep it all on one page? Instead of going to a details page?
ReplyDelete